Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial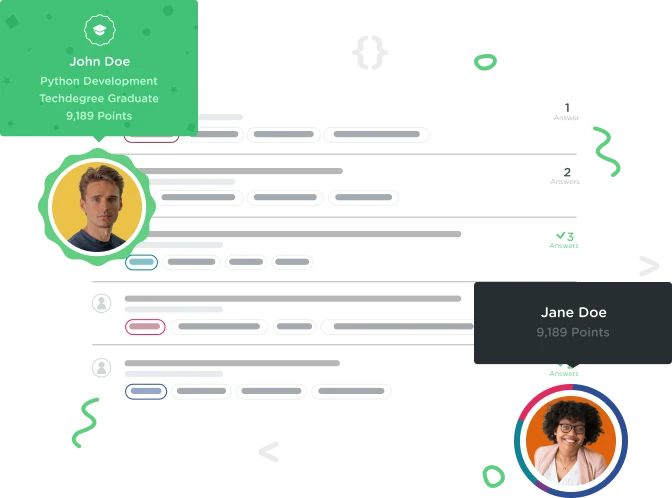

T. Gontarek
7,030 PointsSeeking review for last 3 lines of code.
const h1Text = document.querySelector('h1');
h1Text.textContent='List of things';
// 2: Set the color of the <h1> to a different color
h1Text.style.color = 'lightBlue';
// 3: Set the content of the '.desc' paragraph
// The content should include at least one HTML tag
const pDesc = document.querySelector('p.desc');
pDesc.innerHTML = `<p>This is number 1.<strong>These are strong</strong></p>`
// 4: Set the class of the <ul> to 'list'
const list = document.querySelector('ul')
list.className = 'list';
// 5: Create a new list item and add it to the <ul> (append)
const item = document.createElement('li');
item.innerHTML = "<input>Thing 1";
list.appendChild(item);
// 6: Change all <input> elements from text fields to checkboxes
// My notes in laymens terms for understanding of problem: iterate through the list to change the type of all inputs feilds.
const input = document.querySelectorAll('input');
for (let i = 0; i<input.length; i +=1){
input[i].setAttribute("type","checkbox");
}
// 7: Create a <button> element, and set its text to 'Delete'
// Add the <button> inside the '.extra' <div>
const deleteButton = document.createElement('button');
deleteButton.textContent = 'delete';
const extra = document.querySelector('.extra');
extra.appendChild(deleteButton);
// 8: Remove the '.extra' <div> element from the DOM when a user clicks the 'Delete' button
deleteButton.addEventListener('click', () =>{
extra.remove();
})
Please forgive all the comments. Some are added to help me understand what I am doing. The last 3 lines of code is where I would like someone to lend their expertise. I did mine a little different that the video solution. Must I always use removeChild or is this satisfactory?
1 Answer
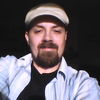
Robert Manolis
Treehouse Guest TeacherHey T, you can use remove instead of removeChild. They both essentially accomplish the same thing. The main difference is that the remove method only requires reference to the element being removed, while the removeChild requires reference to the element to be removed and its parent. Additionally, the remove method doesn't have as much legacy support as removeChild, which you can check if you punch them into the caniuse website.
Hope that helps.
T. Gontarek
7,030 PointsT. Gontarek
7,030 PointsRobert, Thank you for taking the time to answer my question. I'll make sure to use removeChild moving forward.
Thank you!