Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial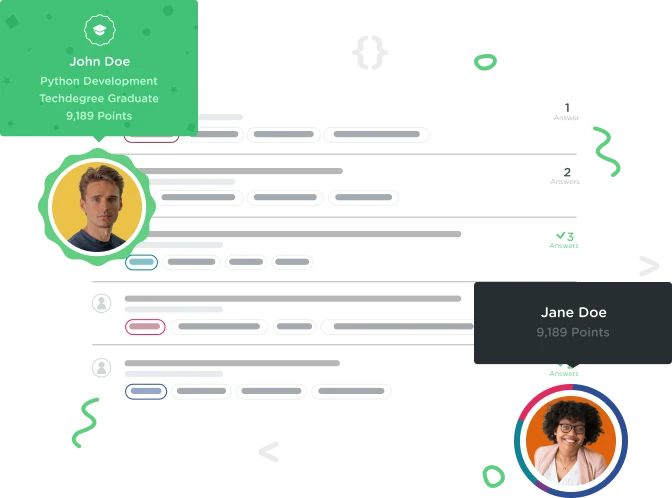

danielchristie
21,505 PointsSeems fine when run in Workspace Mono but test environment states System.ArgumentNullException...
I am on the C# final exam and it requires me to find out the number of times the user would like me to print "yay!" to the screen. I am to ensure their response is valid and a whole number.
Works in Mono but not in the test environment. What am I doing wrong?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
bool persist = true;
var cycles = 0;
while (persist)
{
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
var reply = Console.ReadLine();
try
{
cycles = int.Parse(reply);
if (cycles <= 0)
{
Console.WriteLine(cycles + " is not an acceptable value.");
continue;
}
else
{
persist = false;
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
for (int count = 0; count < cycles; count++)
{
Console.WriteLine("Yay!");
}
}
}
}
2 Answers
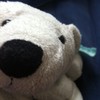
bothxp
16,510 PointsHi Daniel,
I've updated your code to make it pass:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
// bool persist = true;
var cycles = 0;
// while (persist)
// {
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
var reply = Console.ReadLine();
try
{
cycles = int.Parse(reply);
if (cycles <= 0)
{
// Console.WriteLine(cycles + " is not an acceptable value.");
Console.WriteLine("You must enter a positive number.");
// continue;
}
// else
// {
// persist = false;
// }
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
// continue;
}
// }
for (int count = 0; count < cycles; count++)
{
Console.WriteLine("Yay!");
}
}
}
}
I commented out all of the code relating to your while loop and changed one of the writelines.
Firstly your code did work when I was testing it out in a C# workspace. So I can't tell you exactly what the condition was that was causing the error that you were seeing. But what I can tell you is:
You must use the exact wording in the WriteLines that they asked you to. Your use of 'cycles + " is not an acceptable value."' would have caused it to fail the test.
What ever mechanism is running the test in the background is not expecting a while loop in this answer. Time and time again people use a while loop because you have seen it used in the videos for something very similar. But I have not seen an answer that uses a loop pass the test. So I have commented out all of the code relating to the while loop.
I hope that helps a little.
--
Going a little off topic now but I am interested and I have seen this come up quite a few times. Jeremy McLain can a loop be used in this challenge and pass the test? The code:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
int cycles;
String reply;
while(true){
Console.Write("Enter the number of times to print \"Yay!\": ");
reply = Console.ReadLine();
try {
cycles = int.Parse(reply);
for (int count = 0; count < cycles; count++){
Console.WriteLine("Yay!");
}
break;
}
catch(FormatException) {
Console.WriteLine("You must enter a whole number.");
}
}
}
}
}
Will pass task 1, but on task 2 generates the same error that Daniel was getting:
System.ArgumentNullException: Value cannot be null.
Parameter name: String
at System.Number.StringToNumber (System.String str, NumberStyles options, System.NumberBuffer& number, System.Globalization.NumberFormatInfo info, Boolean parseDecimal) [0x00054] in /builddir/build/BUILD/mono-4.2.1/external/referencesource/mscorlib/system/number.cs:1080
at System.Number.ParseInt32 (System.String s, NumberStyles style, System.Globalization.NumberFormatInfo info) [0x00014] in /builddir/build/BUILD/mono-4.2.1/external/referencesource/mscorlib/system/number.cs:751
at System.Int32.Parse (System.String s) [0x00000] in /builddir/build/BUILD/mono-4.2.1/external/referencesource/mscorlib/system/int32.cs:140
at Treehouse.CodeChallenges.Program.Main () <0x40bff830 + 0x00056> in :0
at MonoTester.Run () [0x00197] in MonoTester.cs:124
at MonoTester.Main (System.String[] args) [0x00013] in MonoTester.cs:27
Running the same code in a Workspace seems to happily work when the input is:
treehouse:~/workspaces$ mono Program.exe
Enter the number of times to print "Yay!: 2
Yay!
Yay!
treehouse:~/workspaces$ mono Program.exe
Enter the number of times to print "Yay!:
You must enter a whole number.
Enter the number of times to print "Yay!: 1.1
You must enter a whole number.
Enter the number of times to print "Yay!: -1
treehouse:~/workspaces$ mono Program.exe
Enter the number of times to print "Yay!: test
You must enter a whole number.
Enter the number of times to print "Yay!: -1.1
You must enter a whole number.
So the code works with a null entry, 1, 1.1 -1, -1.1 and text .... so what is causing the task to fail?
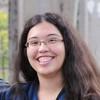
Danielle Gonsalves
Full Stack JavaScript Techdegree Graduate 21,324 PointsHello! I was also having this problem. After doing some research on the msdn, I found out that the Console.ReadLine(); was returning null, getting the same exception. My fix was to check for null. Here's what I did:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
int number;
int i = 0;
while(true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
// if (entry == null)
// {
// break;
// }
try
{
number = int.Parse(entry);
while (i < number)
{
i += 1;
Console.WriteLine("Yay!");
}
break;
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
}
I commented out my null check. After inserting that, it passed the test. This is the msdn article I found, at the second bullet point: Link I believe my problem was that the test environment was redirecting the console from a file, since it worked in Workspaces before adding the null check.
danielchristie
21,505 Pointsdanielchristie
21,505 PointsHey thank you for your validation on my code. I thought it was a bug in the test environment. Glad I am not going crazy. =)