Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial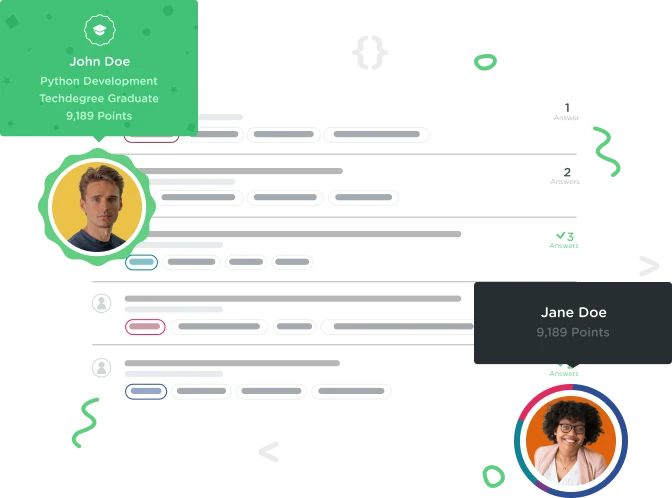

mo ode
4,352 PointsSelect all child elements of section and assign them to the paragraphs variable.
Select all child elements of section and assign them to the paragraphs variable. Can you help?
var section = document.querySelector('section');
var paragraphs;
<!DOCTYPE html>
<html>
<head>
<title>Child Traversal</title>
</head>
<body>
<section>
<p>This is the first paragraph</p>
<p>This is a slightly longer, second paragraph</p>
<p>Shorter, last paragraph</p>
</section>
<footer>
<p>© 2019</p>
</footer>
<script src="app.js"></script>
</body>
</html>
7 Answers
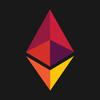
Richard Verbraak
7,739 PointsYou should use the children property.
var paragraphs = section.children;
Look up more videos on DOM traversal. The example about it visualising as a tree helped me a lot.
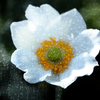
ellie adam
26,377 Pointsvar section = document.querySelector('section'); var paragraphs = section.children;

Michael Barlow
34,317 Pointssection.children
and section.querySelectorAll('p')
will both provide objects through which you can interact with the paragraph DOM elements. It's important to note, however, that they return very different objects. The children
property is an HTMLCollection while querySelectorAll
returns a NodeList, and their APIs differ (e.g. there is no forEach
method on an HTMLCollection). Depending on what you want to do with the paragraphs
variable should impact which one you choose.
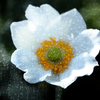
ellie adam
26,377 Pointsvar section = document.querySelector('section');
var paragraphs = section.children;
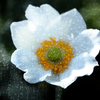
ellie adam
26,377 PointsHow do I delete answer here?? Answer got posted multiple times here.
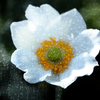
ellie adam
26,377 Pointsvar section = document.querySelector('section'); var paragraphs = section.children;

Muhammad Tahir
4,113 PointsHere is what you need to add:
var paragraphs = section.querySelectorAll('p');