Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial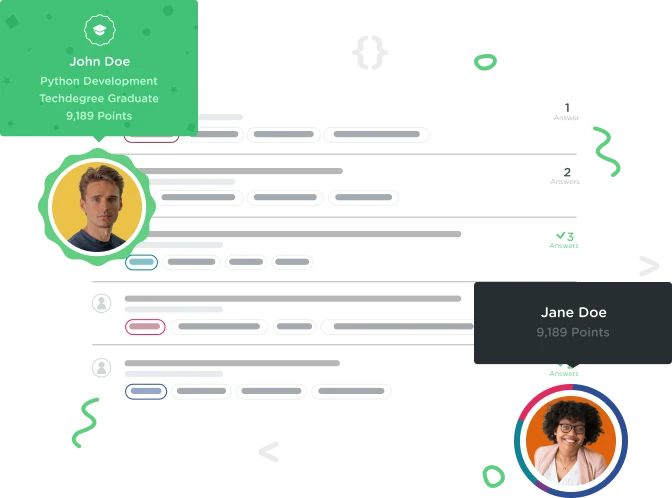
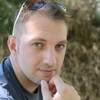
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 Pointsselecting a UL with an ID tag in javascript selectors having a hard time finding the solution.
the question states
The for loop cycles over list items and applies a color to each item using the values stored in the colors array. For example, the first color in the array ( #C2272D) is applied to the first list item, the second color (#F8931F) to the second list item, and so on. Complete the code by setting the variable listItems to refer to a collection. The collection should contain all list items in the <ul> element with the ID of rainbow.
I am unsure how to target the ul with an id tag in this instance.
I have tried listItems= document.querySelectorAll('ul , #rainbow'); listItems= document.querySelectorAll('ul#rainbow'); listItems= document.querySelectorAll('li');
etc but all of it seems to return the same error. Rewatched the video on queryselectors, but its really lacking in information and on the MDN side they dont have an example that matches this. It is straight forward to select multiple elements or target specific things but in this case I just cant figure out the syntax. Perhaps my brain is jus tired.
var listItems;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
listItems= document.querySelectorAll('ul #rainbow');
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
2 Answers

KRIS NIKOLAISEN
54,968 PointsYou are using the ul (by id) as part of the selection. But ultimately you want the list items. querySelectorAll returns a collection. If you had a collection of ul elements the code would apply the same color to all list items and would only iterate for the count of ul elements (in this case once). With a collection of list items you can apply styles to each list item individually.

KRIS NIKOLAISEN
54,968 PointsTo select the list items for the ul with id=rainbow you can use a descendant selector. Here is an example:
div p {
background-color: yellow;
}
The above selects all <p> elements inside <div> elements.
So for the challenge you would use:
var listItems = document.querySelectorAll('#rainbow li')
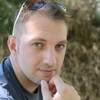
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsHI Kris. thanks for the response. So Based on the question where they specify the UL element with a Rainbow ID should I not be using the UL to grab the element rather than the li?
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsDoron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsI blundered my way through this but im assuming my answer is incorrect.
on line 3 i inserted in
listItems= document.querySelectorAll('li')
so that my code was
this was marked as correct, but in the question they specifically ask to use the UL element with the id of rainbow. my workaround obviously only works because the HTML only contains one list.