Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial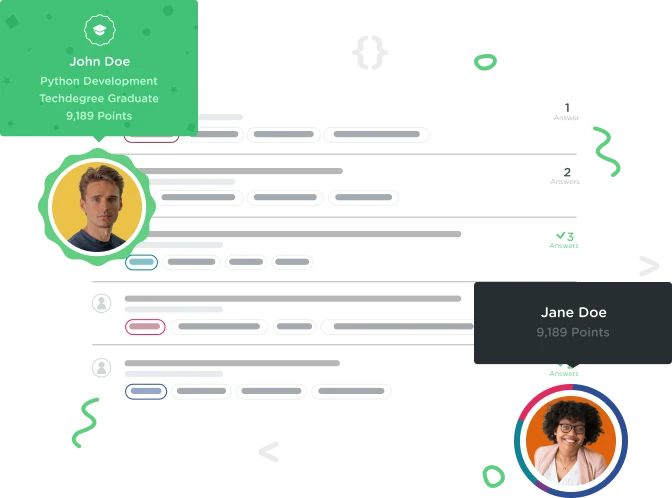
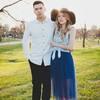
Mike Gugliuzza
8,497 PointsSelecting elements that have data-bind attributes with Python
Hi there- I'm trying to use Python to copy email addresses from a webpage- though the email addresses on the page don't have consistent ID's- instead, the one thing they do have in common is the following databind attribute. Can anyone please tell me how I could select and copy all elements on a page that have the "textWithTitle: EmailAddress" attribute using Python?
data-bind="textWithTitle: EmailAddress, emailPopup: { email: EmailAddress, viewedFrom: ($parent.viewedFrom) ? $parent.viewedFrom : $parents[2].viewedFrom, subject: $parent.emailSubject, cc: $parent.cc }"
Thanks!
3 Answers
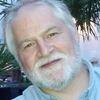
Jeff Muday
Treehouse Moderator 28,716 PointsI don't think I can answer the question with the data you supplied, but the following two concepts will probably get you to a solution:
Python's JSON library using (if you have a well-formed JSON string)
import json
data_string = <some JSON compatible string>
my_data_dictionary = json.loads(data_string)
print(my_data_dictionary['my_key']
-or-
Python's regular expression library which would allow you to parse out the string associated with the key.
-or-
Use Python's string method of find to locate the key, and then the colon and the comma after to delimit the value. You might have to use this kind of kludgy method if the JSON is not well-formed enough to use the JSON library.
An example that locates the key "email" and pulls out the data "joe@treehouse.com"
def find_key_value(data_string, key):
# find the value associated with the key in the data_string
key_end_pos = data_string.find(key) + len(key)
value_start_pos = data_string.find(':', key_end_pos) + 1
value_end_pos = data_string.find(',', value_start_pos)
value = data_string[value_start_pos:value_end_pos].strip()
return value
data_string = 'name: Joe, information: {email:joe@treehouse.com, age:32}'
key = 'email'
print(find_key_value(data_string, key))
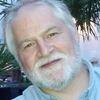
Jeff Muday
Treehouse Moderator 28,716 PointsMy pleasure to help out. Python is very useful (and great fun to program). Eric McKibbin's post above points to some valuable resources you should definitely check out.
Regular expressions (RE) are very powerful but can be frustrating to learn. But it doesn't mean you shouldn't study them (and refer to lots of outside resources and Stack Overflow when you get stuck!)
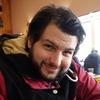
Eric M
11,545 PointsHi Mike,
In addition to Jeff's helpful answer, you'll probably get some use out of the Treehouse course on Scraping Data from the Web with Python and possibly Regular Expressions in Python.
Cheers,
Eric
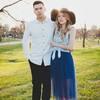
Mike Gugliuzza
8,497 PointsThat's great Eric- I didn't know we had those courses. I'll check them out!
Mike Gugliuzza
8,497 PointsMike Gugliuzza
8,497 PointsThank you Jeff- I think this will definitely point me in the right direction. Thanks for your time!