Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial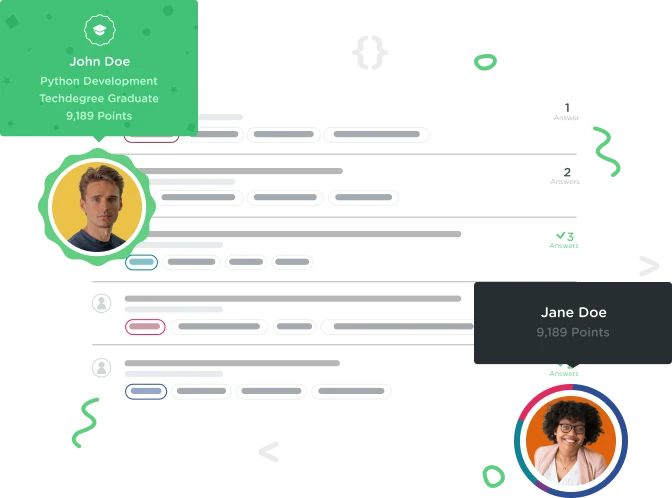

peter keves
6,854 PointsSelf meaning
I still can't properly understand the self the way I see it is that self is basically the instance or self is same as the instance is that correct ? if not what is it
2 Answers
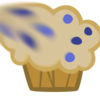
David McCarty
5,214 PointsWhen you pass "self" into a method, essentially what you're doing is passing the instance of that object into the method so that you can call the object and get it's attributes.
Look at it this way, say we have a Class, we'll call it "OurClass"
import random
class OurClass:
name = random.choice(["Bill", "Kary", "Not Bill", "Fred"])
def get_name(self):
return "HI! My name is {}".format(self.name)
As we can see, in our new class we have a name variable that holds a string. Everytime we create a OurClass object it picks a random name from our list of names. Lucky for us we made a method called "get_name" so we can figure out, in a nice way, what our new objects name is!
Let's say we do this like so (we're in a python console now):
>>> import OurClass
>>> new_object = OurClass()
>>> new_object.get_name()
HI! My name is Bill
So, what happened here? Well when we created the object it picked one of the names we provided in the list, this time it was "Bill". We then called the method "get_name" to see what our new object was called.
So, what is "self". Self is basically "new_object" in this instance. Self essentially passes "new_object" back into the method "get_name" automatically so that way we can get it's attributes and special information. In this case, it's got a randomized name.
If we just tried this:
def get_name():
return "HI! My name is {}".format(name)
We get an error! Why? It's because "name" refers to nothing. "self.name" on the other hand calls the passed in objects name attribute. Since it was set randomly to "Bill" it returns the string "Bill" to the .format method.
"Self" is just a placeholder that says "this is a reference to it*self*". So we can use it's attributes without knowing exactly what they are (in case they are random or have been changed by another program)
Hope that helps!
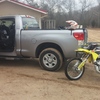
Ryan Ruscett
23,309 PointsHey,
That is correct. Self is the first parameter of every method within a class. I have given you some great links to answers of this very question.
It's definitely a different concept. And does a lot more than what meets the eye. Do we really care about that? After all, we can just use it and it works, orrr It's up to you. You can take it for what it is or you can read more on what it actually is below.