Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial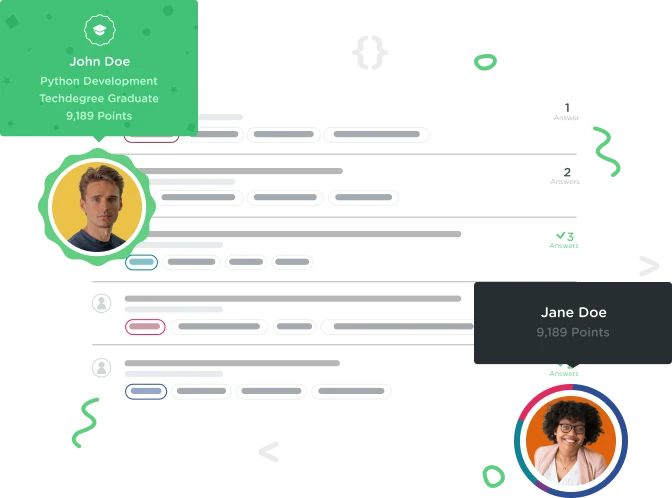

Kasey Knudsen
5,888 Pointsself.is_moving = False
I'm not quite clear on why we are setting self.is_moving to False in the stop method if it's already set to False in the def init method?
3 Answers
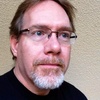
Chris Freeman
Treehouse Moderator 68,457 PointsGood question. While the __init__
method establishes the initial value for the is_moving
attribute, at some point the value may be set to True
by another means. The stop()
method could check to see if the is_moving
attribute needs to be reset before reassigning. Sometimes simply resetting the value regardless of the current value works as well and makes the code intent obvious. Instead of saying "reset if set" it says "reset regardless".
Post back if you want more help. Good luck!!
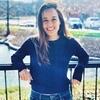
Laura Mora Freeman
8,192 PointsThank you so much Chris for taking the time, it really clarifies it.
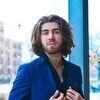
Ali Colak
2,192 PointsHey Chris, Im a little confused on this bit.
"why the first time when the stop function is called, it prints the else statement instead of the if?
Initially, the car not moving (self.is_moving is False). So, the statement is if False⦠this triggers the else block."
def stop(self):
if self.isMoving:
print("The car is stopped!")
self.isMoving = False
else:
print("The car been stopped")
So if self.isMoving -> if False would be the interpretation I'm assuming because it was set to false in:
def __init__(self, model, year, make = "Ford!"):
self.make = make
self.model = model
self.year = year
self.isMoving = False
Wouldn't if False = True because the self.isMoving is False, prompting the first "The Car is Stopped"? Im a little lost as to why the if statement exists if it'll never run
[MOD: added ```python formatting -cf]
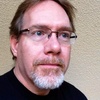
Chris Freeman
Treehouse Moderator 68,457 PointsHey Ali Colak, be careful when reading truthy statements:
# this relies on the actual value of the attribute
# as the entire conditional statement
If could_be_false:
print(βcould_be_false was Trueβ
else:
print(βcould_be_false was Falseβ
# the above behaves exactly the same as
if could_be_false == True:
So, self.isMoving
being initiated to False
means the first block of code will not be executed.
# initially
if self.isMoving: # evaluate as False -> execute else block
# same as
if self.isMoving == True: # evaluates as False -> execute else block
And, as you have stated, the first block of code may never be executed if another method doesnβt set self.isMoving = True
.
The go
method will set this attribute to True
this will enable the first block to execute.
Post back if you need more help. Good luck!!!
Laura Mora Freeman
8,192 PointsLaura Mora Freeman
8,192 PointsCan you comment a little bit more about it, i had the same question, also wasn't it easy to set it to true at the begging?. And why the first time when the stop function is called, it prints the else statement instead of the if? The if is true and the else is false?, so when we reasure self.is_moving = False it goes to the else statement? An also in the go statement i didn't understand the if not self.is_moving and then set to true again. i don't know if i made myself clear. Thanx in advance.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsSure Laura Mora Freeman,
wasn't it easy to set [self.is_moving] to true at the beginning?
Yes, in the
__init__
method,self.is_moving
is set toFalse
when an instance is created.why the first time when the stop function is called, it prints the else statement instead of the if?
Initially, the car not moving (
self.is_moving
isFalse
). So, the statement isif Falseβ¦
this triggers theelse
block.The if is true and the else is false?, so when we reasure self.is_moving = False it goes to the else statement?
Not exactly. The
self.is_moving
is only set toTrue
if thego
method is called.An also in the go statement i didn't understand the if not self.is_moving and then set to true again.
If the car is not moving (is_moving is False), then
if not Falseβ¦
will execute. Expanding my answer above, if the only action after checking if something isFalse
is to set it toTrue
, then theif
isnβt strictly necessary since regardless of its current value,self.is_moving
becomesTrue
. However, since there is a print statement that should only execute on the first call togo
theif
is needed to block the print on subsequent calls togo
if the car is already moving.The methods run are:
__init__
β starts FalseIn summary,
__init__
initializesself.is_moving
toFalse
,go
method setsself.is_moving
toTrue
, andstop
method sets it toFalse
.Hope Iβve covered your question. Post back if you need more help. Good luck!!!