Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial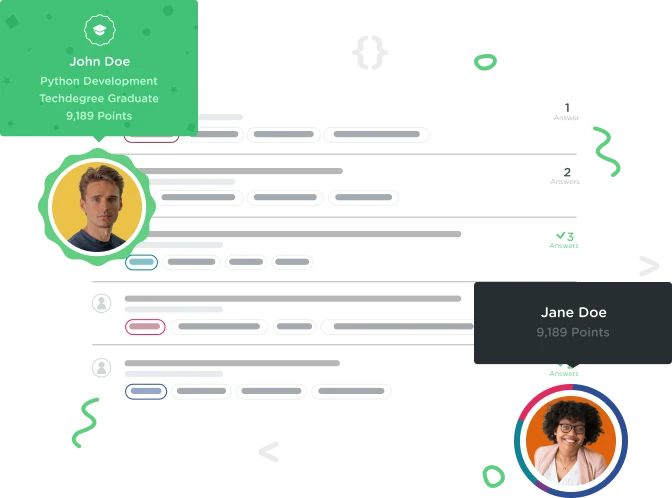

Marcio Porto
6,834 PointsSend action not showing in ActionBar
My action bar is showing fine, but there are no actions on the top right corner (neither with the title or with icon). Any ideas why that might be the case?
RecipientsActivity.java:
package com.marcioporto.android.ribbit;
import android.app.AlertDialog; import android.app.ListActivity; import android.os.Bundle; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.ArrayAdapter; import android.widget.ListView;
import com.parse.FindCallback; import com.parse.ParseException; import com.parse.ParseQuery; import com.parse.ParseRelation; import com.parse.ParseUser;
import java.util.List;
public class RecipientsActivity extends ListActivity {
private static final String TAG = RecipientsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_recipients);
getActionBar().setDisplayHomeAsUpEnabled(true);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
// Start progress bar
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
// Hide progress bar
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
getListView().getContext(),
android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_recipients, menu);
mSendMenuItem = menu.getItem(0);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
switch (id) {
case R.id.action_send:
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
if (l.getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
} else {
mSendMenuItem.setVisible(false);
}
}
}
activity_recipients.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.marcioporto.android.ribbit.RecipientsActivity">
<ListView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@android:id/list"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@android:id/empty"
android:text="@string/empty_recipients_list_message" />
</RelativeLayout>
Manifest file:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.marcioporto.android.ribbit" >
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-feature
android:name="android.hardware.camera"
android:required="true" />
<application
android:name=".ParoliApplication"
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name"
android:screenOrientation="portrait" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:name=".LoginActivity"
android:label="@string/app_name"
android:screenOrientation="portrait" >
</activity>
<activity
android:name=".SignUpActivity"
android:label="@string/app_name"
android:parentActivityName=".LoginActivity"
android:screenOrientation="portrait" >
</activity>
<activity
android:name=".EditFriendsActivity"
android:label="@string/title_activity_edit_friends"
android:parentActivityName=".MainActivity"
android:screenOrientation="portrait"
android:theme="@android:style/Theme.Holo.Light">
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value="com.marcioporto.android.ribbit.MainActivity" />
</activity>
<activity
android:name=".RecipientsActivity"
android:label="@string/title_activity_recipients"
android:parentActivityName=".MainActivity"
android:screenOrientation="portrait"
android:theme="@android:style/Theme.Holo.Light" >
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value="com.marcioporto.android.ribbit.MainActivity" />
</activity>
</application>
</manifest>
11 Answers

Akash Popat
Courses Plus Student 1,154 PointsDont extend ListView. Keep it implementing whatever it was by default, for example in mine in extends AppCompatActivity though in other sdk version I've heard its different. After doing this you'll have to change your XML listview's and textviews's ID and call them in you java by using findViewbyId.

TheBigoso /\
3,217 PointsMy action bar is showing up fine In my app as well. Once I select a picture the actionbar disappears and the send button cant be accessed. When I put in the code send button code presented by ben the app crashes.
I made a Video Showing my problem. https://www.youtube.com/watch?v=8-fttqs5CeQ
Here is my project on github https://github.com/TheBigOso/Ribbit2
I currently know where the problem is occurring. It is hashed out at the bottom of RecipientsActivity.java. This is due to ListActivity being deprecated and android adding AppCompatActivity
So it should look something like this
public class RecipientsActivity extends AppCompatActivity {
not like this
public class RecipientsActivity extends ListActivity {
When you google AppCompatActivity their is a Public Method we can call named setSupportActionBar . https://developer.android.com/reference/android/support/v7/app/AppCompatActivity.html#setSupportActionBar(android.support.v7.widget.Toolbar)
Also their is a reply from Kevin Parks regarding the problem. He uses the new AppCompatActivity. I am a bit confused by what he is doing.
Lets get this done together. TheBigOso
RecipientsActivity.java
import android.app.AlertDialog;
import android.app.ListActivity;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.Window;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import java.util.List;
//AppCompatActivity
public class RecipientsActivity extends ListActivity{
public static final String TAG = RecipientsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_recipients);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
mFriendsRelation.getQuery().findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
getListView().getContext(),
android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
// @Override
// public boolean onCreateOptionsMenu(Menu menu) {
// mSendMenuItem =menu.getItem(0);
// return super.onCreateOptionsMenu(menu);
// }
//
// @Override
// protected void onListItemClick(ListView l, View v, int position, long id) {
// super.onListItemClick(l, v, position, id);
//
// mSendMenuItem.setVisible(true);
// }
}
The Code hashed out at the end of RecipientsActivity.java is making my program crash.
@Override
// public boolean onCreateOptionsMenu(Menu menu) {
// mSendMenuItem =menu.getItem(0);
// return super.onCreateOptionsMenu(menu);
// }
//
// @Override
// protected void onListItemClick(ListView l, View v, int position, long id) {
// super.onListItemClick(l, v, position, id);
//
// mSendMenuItem.setVisible(true);
// }
EditFriendsActivity.Java
import android.app.AlertDialog;
import android.app.ListActivity;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import com.parse.SaveCallback;
import java.util.List;
public class EditFriendsActivity extends ListActivity {
public static final String TAG = EditFriendsActivity.class.getSimpleName();
protected List<ParseUser> mUsers;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(getWindow().FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_edit_friends);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
protected void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
setProgressBarIndeterminateVisibility(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.orderByAscending(ParseConstants.KEY_USERNAME);
query.setLimit(1000);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
setProgressBarIndeterminateVisibility(false);
if (e == null) {
//success
mUsers = users;
String[] usernames = new String[mUsers.size()];
int i = 0;
for (ParseUser user : mUsers) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
EditFriendsActivity.this,
android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
addFriendCheckmarks();
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
if(getListView().isItemChecked(position)){
//add friend
mFriendsRelation.add(mUsers.get(position));
}
else {
//remove friend
mFriendsRelation.remove(mUsers.get(position));
}
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e(TAG, e.getMessage());
}
}
});
}
private void addFriendCheckmarks(){
mFriendsRelation.getQuery().findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if(e == null){
//list returned - look for match
for(int i = 0; i<mUsers.size(); i++){
ParseUser user = mUsers.get(i);
for(ParseUser friend : friends){
if(friend.getObjectId().equals(user.getObjectId())){
getListView().setItemChecked(i, true);
}
}
}
}
else {
Log.e(TAG, e.getMessage());
}
}
});
}
}

Akash Popat
Courses Plus Student 1,154 PointsThe commented portion is making your app crash because it is trying to make an overflow menu in the actionbar but you dont have one. If you extend ListActivity you wont have an actionbar to use and therefore you cant make an overflow menu.
Here check my code out. I have made very few changes then the videos.
'''android package com.akashpopat.snap;
import android.app.AlertDialog; import android.net.Uri; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.Toast;
import com.parse.FindCallback; import com.parse.ParseException; import com.parse.ParseFile; import com.parse.ParseObject; import com.parse.ParseQuery; import com.parse.ParseRelation; import com.parse.ParseUser; import com.parse.ProgressCallback; import com.parse.SaveCallback;
import java.util.ArrayList; import java.util.List;
public class RecipientsActivity extends AppCompatActivity {
public static Boolean SENT = false;
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
ListView mListView;
Uri mMediaUri;
String mFileType;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_recipients);
mMediaUri = getIntent().getData();
mFileType = getIntent().getStringExtra(ParseConstants.KEY_FILE_TYPE);
mListView = (ListView) findViewById(R.id.lv);
mListView.setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(RecipientsActivity.this
, android.R.layout.simple_list_item_checked
, usernames);
mListView.setAdapter(adapter);
if (friends.size() > 0) {
findViewById(R.id.khali).setVisibility(View.GONE);
}
} else {
Log.e("hey", e.getMessage());
// Didnt work
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
mListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
if (mListView.getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
} else {
mSendMenuItem.setVisible(false);
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_recipients, menu);
mSendMenuItem = menu.getItem(0);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()){
case R.id.action_send:
ParseObject message = createMessage();
if(message == null){
// error
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage(R.string.error_selecting_file)
.setTitle(R.string.sorry_error)
.setPositiveButton(android.R.string.ok,null);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
send(message);
}
finish();
return true;
}
return super.onOptionsItemSelected(item);
}
private ParseObject createMessage() {
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID,ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME,ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPIENTS_IDS,getRecipientIds());
message.put(ParseConstants.KEY_FILE_TYPE,mFileType);
byte[] fileBytes = FileHelper.getByteArrayFromFile(this, mMediaUri);
if (fileBytes == null)
{
return null;
}
else {
if(mFileType.equals(ParseConstants.TYPE_IMAGE)){
fileBytes = FileHelper.reduceImageForUpload(fileBytes);
}
String fileName = FileHelper.getFileName(this,mMediaUri,mFileType);
ParseFile file = new ParseFile(fileName,fileBytes);
file.saveInBackground(new SaveCallback() {
public void done(ParseException e) {
// Handle success or failure here ...
Log.d("hey","files away");
}
}, new ProgressCallback() {
public void done(Integer percentDone) {
// Update your progress spinner here. percentDone will be between 0 and 100.
Log.d("hey", "files at " + percentDone);
}
});
message.put(ParseConstants.KEY_FILE_, file);
return message;
}
}
private ArrayList<String> getRecipientIds() {
ArrayList<String> recipientIds = new ArrayList<>();
for(int i = 0;i < mListView.getCount();i++)
{
if (mListView.isItemChecked(i)){
recipientIds.add(mFriends.get(i).getObjectId());
}
}
return recipientIds;
}
void send(ParseObject message){
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if(e == null)
{
SENT = true;
Toast.makeText(RecipientsActivity.this,"Sent!", Toast.LENGTH_LONG).show();
}
else {
// error
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(R.string.error_sending_message)
.setTitle(R.string.sorry_error)
.setPositiveButton(android.R.string.ok,null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
} '''

TheBigoso /\
3,217 PointsThank you Akash Popat,
Your a Saint. here is my github project. https://github.com/TheBigOso/Ribbit2
Im looking at your code. now i have these errors
Error:Content is not allowed in prolog. Error:Execution failed for task ':app:mergeDebugResources'.
C:\Oso\Crap\androidStudio\Torpedo\Torpedo\app\src\main\res\libs\commons-io-2.4.jar:1:1: Error: Content is not allowed in prolog. :app:mergeDebugResources FAILED C:\Oso\Crap\androidStudio\Torpedo\Torpedo\app\src\main\res\libs\commons-io-2.4.jar Error:(1, 1) Error: Content is not allowed in prolog.
everything complies but everywhere you see R.something for example in setContentView(R.layout.activity_recipients); the R "Cannot resolve symble R"
i added the commons-ip-2.4.jar file in my libs directory.
do you know how to fix this?
thanks, Oso

Akash Popat
Courses Plus Student 1,154 PointsThis is just a bug because of copy pasting. Trying rebuilding the code and syncing the gradle files and if these two dont work then go to File and invalidate cache and restart.

Akash Popat
Courses Plus Student 1,154 PointsOh and I'm not sure if you changed to xml to reflect the changes in the java. Its not much but you have to change the ids. I kept my ListView id as "lv" and textview which will show when the listview is empty has the id "khali"

TheBigoso /\
3,217 Pointsumm I invalidate cache and restarted it and now it needs an update should be a few min.

TheBigoso /\
3,217 Points<ListView android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/lv" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/khali"
android:text="@string/empty_recipients_list_message"
/>
i changed it. It should look like this right?

Akash Popat
Courses Plus Student 1,154 PointsYeah and the listviews too. But this is only if you copied my java code. Its because I used these ids to reference them.

TheBigoso /\
3,217 Pointsyeah I understand. now im still getting this error here is a video of it.
https://www.youtube.com/watch?v=nwp9juCodz4
this is my error log :(
Error:Content is not allowed in prolog. C:\Oso\Crap\androidStudio\Torpedo\Torpedo\app\src\main\res\libs\commons-io-2.4.jar Error:(1, 1) Error: Content is not allowed in prolog. :app:mergeDebugResources FAILED Error:Execution failed for task ':app:mergeDebugResources'.
C:\Oso\Crap\androidStudio\Torpedo\Torpedo\app\src\main\res\libs\commons-io-2.4.jar:1:1: Error: Content is not allowed in prolog.
Thank you for your help.
Oso

Akash Popat
Courses Plus Student 1,154 PointsOkay I found the problem though I dont understand how your code was working up till now.
The problem was your libs folder which should be under the app folder was somehow inside the resources folder. And your app gradle should have ''' compile fileTree(dir: 'libs', include: "commons-io-2.4.jar") '''
After I fixed that I got many other problems in your code which I got from github. For example you didnt change the xml ids but those are simple fixes so I guess it should work after you fix all that.

TheBigoso /\
3,217 PointsThanks for looking through my code. So after doing extensive research I found out that Android Studio had an error and couldn't load the R.class. After reinstalling. Now im back to square one. my program compiles. I can take a picture. Then I press ok. Thats when my app crashes.
same thing happens if i choose a picture i never see my friends.
this is the error log can provided
10-21 17:24:14.905 3190-3190/? E/AndroidRuntime: java.lang.IndexOutOfBoundsException: Invalid index 0, size is 0
10-21 17:24:14.905 3190-3190/? E/AndroidRuntime: at java.util.ArrayList.throwIndexOutOfBoundsException(ArrayList.java:255)
here is my git hub https://github.com/TheBigOso/RibbitSendButton
I Dont know what im missing
What does your menu_main.xml look like? here is mine
Thank you for everthing
TheBigOso
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
tools:context=".MainActivity">
<item android:id="@+id/action_logout"
android:title="@string/menu_logout_label"
/>
<item android:id="@+id/action_edit_friends"
android:title="@string/menu_edit_friends_label"
/>
<item android:id="@+id/action_camera"
android:title="@string/menu_camera_label"
android:icon="@mipmap/ic_camera_enhance_white_24dp"/>
<!--android:showAsAction="always"-->
<item android:id="@+id/action_send"
android:orderInCategory="100"
android:title="@string/menu_send_label"
android:visible="false"
android:icon="@mipmap/ic_camera_enhance_white_24dp"/>
</menu>
RecipientsActivity.Java
package com.fartyou.thedirtyappstore.ribbit2;
import android.app.AlertDialog;
import android.support.v4.app.NavUtils;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import java.util.List;
public class RecipientsActivity extends AppCompatActivity {
public static final String TAG = RecipientsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
protected ListView mListView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_recipients);
mListView = (ListView) findViewById(R.id.lv);
mListView.setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
mFriendsRelation.getQuery().findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(RecipientsActivity.this
, android.R.layout.simple_list_item_checked
, usernames);
mListView.setAdapter(adapter);
if (friends.size() > 0) {
findViewById(R.id.tv).setVisibility(View.GONE);
}
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
mListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
if (mListView.getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
} else {
mSendMenuItem.setVisible(false);
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
mSendMenuItem = menu.getItem(0);
getMenuInflater().inflate(R.menu.menu_main, menu);
mSendMenuItem = menu.getItem(0);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
NavUtils.navigateUpFromSameTask(this);
return true;
case R.id.action_send:
return true;
}
return super.onOptionsItemSelected(item);
}
}
here is my activity_recipients.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context="com.fartyou.thedirtyappstore.ribbit2.RecipientsActivity">
<ListView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/lv"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/tv"
android:text="@string/empty_recipients_list_message"
/>
</RelativeLayout>

Alex Fujimoto
19,013 PointsDid you ever Figure this out? Ive been working on this one video all day and everything I do just makes everything worse. Everything I try just doesnt work and I dont even understand what the baseline problems is.

Marcio Porto
6,834 PointsHonestly, I only got it to work at the end of the Ribbit design course. You're going to make some changes to this file later in order to add some styling and then you'll have the custom action bar with the send button.