Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial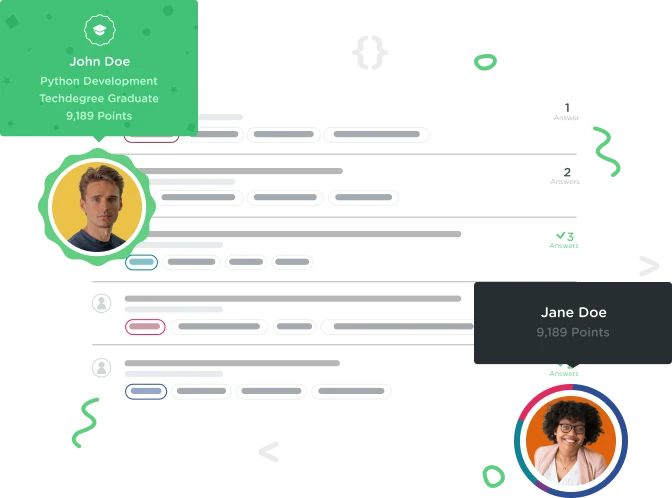

Julian Hurley
3,133 PointsSend an email with Rails
I've been reading up on the ActionMailer, but I'm having real trouble sending an email with ROR3.
I'm trying to follow section 2.1 of this tutorial
When I create a new user, I just get this message:
NameError in UsersController#create
uninitialized constant UsersController::UserMailer
I've had a break from ROR, and now I've found I've forgotten quite a lot!
What are the most likely causes of this error?
My mailer (controller): app/mailers/usermailer.rb:
class Usermailer < ActionMailer::Base
default from: "from@example.com"
def welcome_email(user)
@user = user
@url = 'http://example.com/login'
mail(to: @user.email, subject: 'Welcome to my awesome site')
end
end
My view: app/usermailer/welcome_email.html.erb:
<!DOCTYPE html>
<html>
<head>
<meta content='text/html; charset=UTF-8' http-equiv='Content-Type' />
</head>
<body>
<h1>Welcome to example.com, <%= @user.name %></h1>
<p>
You have successfully signed up to example.com,
your username is: <%= @user.login %>.<br/>
</p>
<p>
To login to the site, just follow this link: <%= @url %>.
</p>
<p>Thanks for joining and have a great day!</p>
</body>
</htm>
Not sure what this is classed as. A "view call" in the users controller to the view welcome_email to @user:
UserMailer.welcome_email(@user).deliver
Here it is in context at app/controllers/users_controller.rb:
class UsersController < ApplicationController
# GET /users
# GET /users.json
def index
@users = User.all
respond_to do |format|
format.html # index.html.erb
format.json { render json: @users }
end
end
# GET /users/1
# GET /users/1.json
def show
@user = User.find(params[:id])
respond_to do |format|
format.html # show.html.erb
format.json { render json: @user }
end
end
# GET /users/new
# GET /users/new.json
def new
@user = User.new
respond_to do |format|
format.html # new.html.erb
format.json { render json: @user }
end
end
# GET /users/1/edit
def edit
@user = User.find(params[:id])
end
# POST /users
# POST /users.json
def create
@user = User.new(params[:user])
respond_to do |format|
if @user.save
# send welcome email after save
UserMailer.welcome_email(@user).deliver
format.html { redirect_to @user, notice: 'User was successfully created.' }
format.json { render json: @user, status: :created, location: @user }
else
format.html { render action: "new" }
format.json { render json: @user.errors, status: :unprocessable_entity }
end
end
end
# PUT /users/1
# PUT /users/1.json
def update
@user = User.find(params[:id])
respond_to do |format|
if @user.update_attributes(params[:user])
format.html { redirect_to @user, notice: 'User was successfully updated.' }
format.json { head :no_content }
else
format.html { render action: "edit" }
format.json { render json: @user.errors, status: :unprocessable_entity }
end
end
end
# DELETE /users/1
# DELETE /users/1.json
def destroy
@user = User.find(params[:id])
@user.destroy
respond_to do |format|
format.html { redirect_to users_url }
format.json { head :no_content }
end
end
end
1 Answer
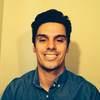
Twiz T
12,588 PointsI think you may have mistyped the name of the user mailer class. In the example above it's
class Usermailer < ActionMailer::Base
and when you restarted from scratch you probably now have
class UserMailer < ActionMailer::Base
Typos are usually the first thing to look for when you see an uninitialized constant error. In the example above in your Users controller you have a reference to
UserMailer.welcome_email(@user).deliver
And the error was saying it didn't know what that was because you had it named as
class Usermailer < ActionMailer::Base
Hope that helps.
-twiz

Julian Hurley
3,133 PointsYes, thankyou very much. Good to know where you've gone wrong!
Julian Hurley
3,133 PointsJulian Hurley
3,133 PointsOkay, I've fixed the error. Not sure what I was doing wrong, but I did it from scratch and now it's working. However, emails are not arriving in their specified inboxes, despite the output from the server stating otherwise:
Sent mail to julian@designimperial.com (2003ms) Date: Fri, 30 Aug 2013 22:26:41 +0100 From: from@example.com To: julian@designimperial.com Message-ID: <52210e11bedc9_16501d84f64257a@Julian-PC.mail> Subject: Welcome to my awesome site! Mime-Version: 1.0 Content-Type: text/html; charset=UTF-8 Content-Transfer-Encoding: 7bit
<!DOCTYPE html> <html> <head> <meta content='text/html; charset=UTF-8' http-equiv='Content-Type' /> </head> <body> <h1>Welcome to example.com, Julian</h1> <p> You have successfully signed up to example.com, your username is: gogo.<br/> </p> <p> To login to the site, just follow this link: http://example.com/login. </p> <p>Thanks for joining and have a great day!</p> </body> </html>
Do I need to upload my rails application to a server or heroku inorder for emails to be sent? I know this is the case with PHP mailing and MAMP