Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial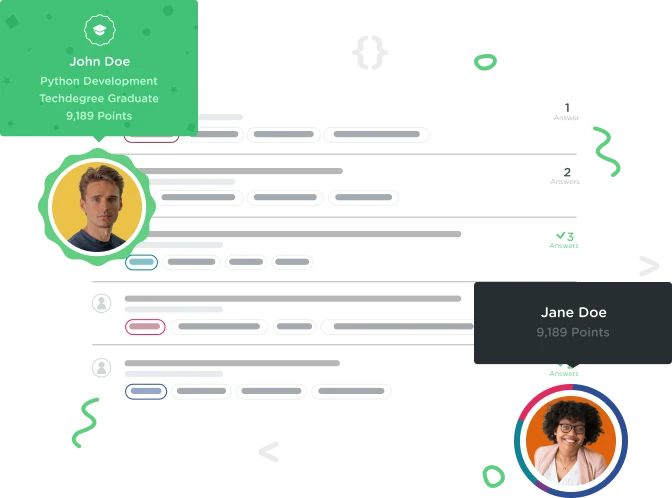

ibbhfsmeaf
4,568 PointsSend forms in an email with PHP
I was watching the PHP Course on here to learn how to get my forms to work, and I got them working: http://desimara.com/forms.php
The problem is I now need to learn how to send them in an email.. can someone help me with this?
I cant continue on with the PHP course because I actually skipped to the point where he did the forms part because that's all I needed, and I cant skip to the email part because he added much more code (shopping card/paypal, etc.) and it completely threw me off.
I just need to email the forms when submitted.
Here is my forms.php code, if it matters:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$first_name = $_POST["first_name"];
$last_name = $_POST["last_name"];
$email = $_POST["email"];
$website_description = $_POST["website_description"];
$design_description = $_POST["design_description"];
$email_body = "";
$divider = "----------------------------------------";
$email_body = $email_body . "First Name: " . $first_name . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Last Name: " . $last_name . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Email: " . $email . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Website Description: " . $website_description . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Design Description: " . $design_description;
header("Location: forms.php?status=complete");
exit;
}
?>
<?php include('header.php'); ?>
<?php if (isset($_GET["status"]) AND $_GET["status"] == "complete") { ?>
<section id="section-one">
<h2>Your request has been sent! I’ll be in touch shortly.</h2>
</section>
<?php } else { ?>
<section id="section-one">
<form method="post" action="forms.php">
<fieldset>
<legend><span class="number">1</span>Personal Information</legend>
<label for="first_name">First Name</label>
<input type="text" id="first_name" name="first_name"><br>
<label for="last_name">Last Name</label>
<input type="text" id="last_name" name="last_name"><br>
<label for="email">Email</label>
<input type="email" id="email" name="email"><br>
</fieldset>
<fieldset>
<legend><span class="number">2</span>Design Information</legend>
<label for="website_description">Description of Website <p class="labelP">(Do you want a portfolio, informational site, etc.)</p></label>
<textarea id="website_description" name="website_description" rows="10" cols="60"></textarea>
<label for="design_description">Description of Design <p class="labelP">(Colors, fonts, etc. If left empty, I will choose.)</p></label>
<textarea id="design_description" name="design_description" rows="10" cols="60"></textarea>
</fieldset>
<hr><br><input type="submit" value="Send Request!" id="finalSubmit"></input>
</form>
</section>
<?php } ?>
<?php include('footer.php'); ?>
</html>
5 Answers
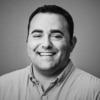
mikes02
Courses Plus Student 16,968 PointsLansana,
Here is some code to review, I tested this with my own e-mail address and it worked:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$first_name = $_POST["first_name"];
$last_name = $_POST["last_name"];
$email = $_POST["email"];
$website_description = $_POST["website_description"];
$design_description = $_POST["design_description"];
$email_body = "";
$divider = "----------------------------------------";
$email_body = $email_body . "First Name: " . $first_name . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Last Name: " . $last_name . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Email: " . $email . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Website Description: " . $website_description . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Design Description: " . $design_description;
$to = 'lxc5296@gmail.com';
$subject = 'Website Request';
$message = $email_body;
$message = wordwrap($message, 70, "\r\n");
mail($to , $subject , $message);
if(!mail($to, $subject, $message)) {
header("Location: index.php?status=error");
} else {
header("Location: index.php?status=complete");
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<?php if (isset($_GET["status"]) AND $_GET["status"] == "complete"): ?>
<section id="section-one">
<h2>Your request has been sent! I’ll be in touch shortly.</h2>
</section>
<?php else: ?>
<section id="section-one">
<form method="post" action="index.php">
<fieldset>
<legend><span class="number">1</span>Personal Information</legend>
<label for="first_name">First Name</label>
<input type="text" id="first_name" name="first_name"><br>
<label for="last_name">Last Name</label>
<input type="text" id="last_name" name="last_name"><br>
<label for="email">Email</label>
<input type="email" id="email" name="email"><br>
</fieldset>
<fieldset>
<legend><span class="number">2</span>Design Information</legend>
<label for="website_description">Description of Website <p class="labelP">(Do you want a portfolio, informational site, etc.)</p></label>
<textarea id="website_description" name="website_description" rows="10" cols="60"></textarea>
<label for="design_description">Description of Design <p class="labelP">(Colors, fonts, etc. If left empty, I will choose.)</p></label>
<textarea id="design_description" name="design_description" rows="10" cols="60"></textarea>
</fieldset>
<hr><br>
<input type="submit" value="Send Request!" id="finalSubmit"></input>
</form>
</section>
<?php endif; ?>
</body>
</html>
Couple things - You had an exit in your IF statement that did not need to be there. You were doing header and footer includes based on whether the e-mail was sent which is not necessary, your header and footer should be there regardless. Also, you should add some required field validation to this, as it is now this form could be submitted completely blank.
Also, you'll notice I am using index.php, that is only because that's what my test file was called, you would need to adjust accordingly.
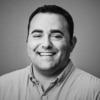
mikes02
Courses Plus Student 16,968 PointsCouple things. One, you are not actually using PHP's mail function anywhere in your code to send the mail. Two, you would probably want to have a conditional to check for success/failure just as a good practice.

ibbhfsmeaf
4,568 PointsThis doesn't seem to be working. I did not get an email.
Any suggestions?
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$first_name = $_POST["first_name"];
$last_name = $_POST["last_name"];
$email = $_POST["email"];
$website_description = $_POST["website_description"];
$design_description = $_POST["design_description"];
$email_body = "";
$divider = "----------------------------------------";
$email_body = $email_body . "First Name: " . $first_name . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Last Name: " . $last_name . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Email: " . $email . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Website Description: " . $website_description . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Design Description: " . $design_description;
$to = '';
$subject = 'Website Request';
$message = $email_body;
$message = wordwrap($message, 70, "\r\n");
mail($to , $subject , $message);
if (!mail($to, $subject, $message)){
header("Location: forms.php?status=error");
} else {
header("Location: forms.php?status=complete");
}
exit;
}
?>
<?php include('header.php'); ?>
<?php if (isset($_GET["status"]) AND $_GET["status"] == "complete") { ?>
<section id="section-one">
<h2>Your request has been sent! I’ll be in touch shortly.</h2>
</section>
<?php } else { ?>
<section id="section-one">
<form method="post" action="forms.php">
<fieldset>
<legend><span class="number">1</span>Personal Information</legend>
<label for="first_name">First Name</label>
<input type="text" id="first_name" name="first_name"><br>
<label for="last_name">Last Name</label>
<input type="text" id="last_name" name="last_name"><br>
<label for="email">Email</label>
<input type="email" id="email" name="email"><br>
</fieldset>
<fieldset>
<legend><span class="number">2</span>Design Information</legend>
<label for="website_description">Description of Website <p class="labelP">(Do you want a portfolio, informational site, etc.)</p></label>
<textarea id="website_description" name="website_description" rows="10" cols="60"></textarea>
<label for="design_description">Description of Design <p class="labelP">(Colors, fonts, etc. If left empty, I will choose.)</p></label>
<textarea id="design_description" name="design_description" rows="10" cols="60"></textarea>
</fieldset>
<hr><br><input type="submit" value="Send Request!" id="finalSubmit"></input>
</form>
</section>
<?php } ?>
<?php include('footer.php'); ?>
</html>
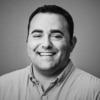
mikes02
Courses Plus Student 16,968 PointsDid you try sending from a local development server or did you upload it and test it?

ibbhfsmeaf
4,568 PointsAh! I uploaded and tested and it gave me the error url.
Here is my updated conditional statement to better suit the error case. What do you think of it? And how can I fix my code so that I don't get an error in the first place?
You can test the form and see the error result at http://desimara.com - click on "request" on the home page.
if (!mail($to, $subject, $message)){ ?>
<?php include('header.php'); ?>
<section id="section-one">
<h2>Something must have went wrong. Please re-submit your information!</h2>
<h2><a href="forms.php" id="request"><?php $section = "Back"; echo $section; ?></a></h2>
</section>
<?php include('footer.php');
} else {
header("Location: forms.php?status=complete");
}
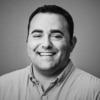
mikes02
Courses Plus Student 16,968 PointsI recommend stripping this down a bit and just get a basic e-mail working and then expand on it from there.

ibbhfsmeaf
4,568 PointsProblem solved. Thanks again.