Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial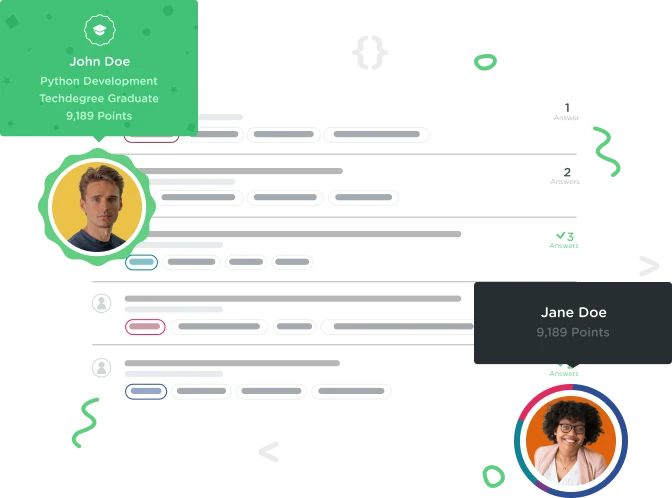

Bryan Runtu
7,367 PointsSending FORM.. Help Please
I have trouble sending a form to my email address. Every time I clicked on submit, it does not do anything. I wonder if my codes are wrong. Please check code below:
HTML
<form class="form contact-form" id="contact_form">
<div class="clearfix">
<div class="cf-left-col">
<!-- Name -->
<div class="form-group">
<input type="text" name="name" id="name" class="input-md round form-control" placeholder="Name" pattern=".{3,100}" required>
</div>
<!-- Email -->
<div class="form-group">
<input type="email" name="email" id="email" class="input-md round form-control" placeholder="Email" pattern=".{5,100}" required>
</div>
</div>
<div class="cf-right-col">
<!-- Message -->
<div class="form-group">
<textarea name="message" id="message" class="input-md round form-control" style="height: 84px;" placeholder="Message"></textarea>
</div>
</div>
</div>
<div class="clearfix">
<div class="cf-left-col">
<!-- Inform Tip -->
<div class="form-tip pt-20"> <i class="fa fa-info-circle"></i> All the fields are required </div>
</div>
<div class="cf-right-col">
<!-- Send Button -->
<div class="align-right pt-10">
<button class="submit_btn btn btn-mod btn-medium btn-round" id="submit_btn">Submit Message</button>
</div>
</div>
</div>
<div id="result"></div>
</form>
jQuery
/* ---------------------------------------------
Contact form
--------------------------------------------- */
$(document).ready(function(){
$("#submit_btn").click(function(){
//get input field values
var user_name = $('input[name=name]').val();
var user_email = $('input[name=email]').val();
var user_message = $('textarea[name=message]').val();
//simple validation at client's end
//we simply change border color to red if empty field using .css()
var proceed = true;
if (user_name == "") {
$('input[name=name]').css('border-color', '#e41919');
proceed = false;
}
if (user_email == "") {
$('input[name=email]').css('border-color', '#e41919');
proceed = false;
}
if (user_message == "") {
$('textarea[name=message]').css('border-color', '#e41919');
proceed = false;
}
//everything looks good! proceed...
if (proceed) {
//data to be sent to server
post_data = {
'userName': user_name,
'userEmail': user_email,
'userMessage': user_message
};
//Ajax post data to server
$.post('contact_me.php', post_data, function(response){
//load json data from server and output message
if (response.type == 'error') {
output = '<div class="error">' + response.text + '</div>';
}
else {
output = '<div class="success">' + response.text + '</div>';
//reset values in all input fields
$('#contact_form input').val('');
$('#contact_form textarea').val('');
}
$("#result").hide().html(output).slideDown();
}, 'json');
}
return false;
});
//reset previously set border colors and hide all message on .keyup()
$("#contact_form input, #contact_form textarea").keyup(function(){
$("#contact_form input, #contact_form textarea").css('border-color', '');
$("#result").slideUp();
});
});
PHP
<?php
if($_POST)
{
$to_Email = "bryanrun2@gmail.com"; //Replace with recipient email address
$subject = 'Message from my website'; //Subject line for emails
//check if its an ajax request, exit if not
if(!isset($_SERVER['HTTP_X_REQUESTED_WITH']) AND strtolower($_SERVER['HTTP_X_REQUESTED_WITH']) != 'xmlhttprequest') {
//exit script outputting json data
$output = json_encode(
array(
'type'=>'error',
'text' => 'Request must come from Ajax'
));
die($output);
}
//check $_POST vars are set, exit if any missing
if(!isset($_POST["userName"]) || !isset($_POST["userEmail"]) || !isset($_POST["userMessage"]))
{
$output = json_encode(array('type'=>'error', 'text' => 'Input fields are empty!'));
die($output);
}
//Sanitize input data using PHP filter_var().
$user_Name = filter_var($_POST["userName"], FILTER_SANITIZE_STRING);
$user_Email = filter_var($_POST["userEmail"], FILTER_SANITIZE_EMAIL);
$user_Message = filter_var($_POST["userMessage"], FILTER_SANITIZE_STRING);
$user_Message = str_replace("\'", "'", $user_Message);
$user_Message = str_replace("'", "'", $user_Message);
//additional php validation
if(strlen($user_Name)<4) // If length is less than 4 it will throw an HTTP error.
{
$output = json_encode(array('type'=>'error', 'text' => 'Name is too short or empty!'));
die($output);
}
if(!filter_var($user_Email, FILTER_VALIDATE_EMAIL)) //email validation
{
$output = json_encode(array('type'=>'error', 'text' => 'Please enter a valid email!'));
die($output);
}
if(strlen($user_Message)<5) //check emtpy message
{
$output = json_encode(array('type'=>'error', 'text' => 'Too short message! Please enter something.'));
die($output);
}
//proceed with PHP email.
$headers = 'From: '.$user_Email.'' . "\r\n" .
'Reply-To: '.$user_Email.'' . "\r\n" .
'X-Mailer: PHP/' . phpversion();
$sentMail = @mail($to_Email, $subject, $user_Message . "\r\n\n" .'-- '.$user_Name. "\r\n" .'-- '.$user_Email, $headers);
if(!$sentMail)
{
$output = json_encode(array('type'=>'error', 'text' => 'Could not send mail! Please check your PHP mail configuration.'));
die($output);
}else{
$output = json_encode(array('type'=>'message', 'text' => 'Hi '.$user_Name .'! Thank you for your email'));
die($output);
}
}
?>
3 Answers
Leo Naga Putra
2,115 PointsHi Bryan,
Your code above is using ajax for calling PHP. If you're using firefox as your browser, press F12 and check on console tab. You will get error message if your code has errors in it, or if your javascript is running without errors, you will get response. Check the response you get from php, it should be in json format.

Greg Kaleka
39,021 PointsHi Bryan,
First thing I notice: you don't have an action attribute set for your form. You need to have the form submit to a specific url. It looks like you have the logic on the same page, so you could simply set action="."
.
<form action="." class="form contact-form" id="contact_form">
Try this, then you can trouble shoot the rest if there are other issues.
Best,
-Greg

Bryan Runtu
7,367 PointsHi Greg,
Thanks for your respond.
Tried it but didn't work. I tried putting action="contact_me.php" but I got nothing :(

Greg Kaleka
39,021 PointsOK, I would start adding in echo or var_dump() statements in your PHP and walk your way through the process. What's in $_POST? Is it what you expect? Are your email variables being populated correctly?
Basic debugging - you'll figure it out :). Report back once you've found out where you went wrong.
Leo Naga Putra
2,115 Pointsput action = "contact_me.php" and change <button> to <input type="submit" value="Submit">. Try this to check whether you php is running well.

Bryan Runtu
7,367 PointsLeo,
Nohing happen.. I'm confuse :(
Leo Naga Putra
2,115 Pointsok, try to write simple code in another php file. For example, create 1 file and name it as test.php. Put this code inside it:
<?php echo "hello world"; ?>
check whether the code is running.
if test.php above works, then you should put this at the top of your php script : ini_set('display_errors', 1);
that is to make sure your script will show error message if it gets any errors.