Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial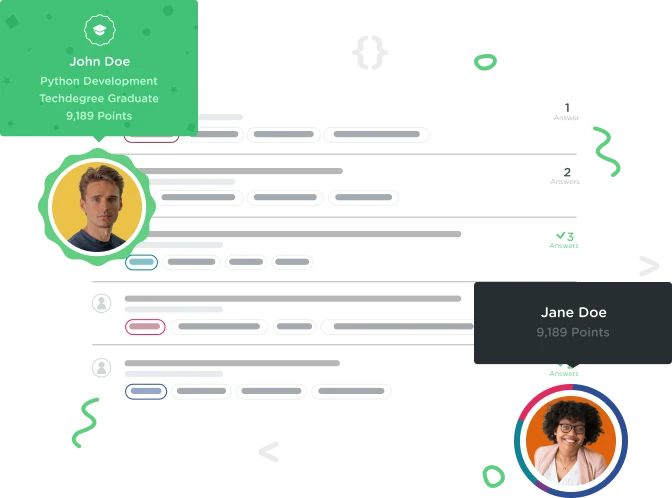

Sohale Vu
18,322 PointsSending the message code challenge
I am trying to do the second task in this code challenge and I'm not sure what I'm doing wrong here is my code:
import android.app.Activity;
import android.widget.EditText;
import android.widget.Toast;
import com.parse.ParseException;
import com.parse.ParseObject;
import com.parse.SaveCallback;
public class MainActivity extends Activity {
protected EditText mDescriptionField;
protected SaveCallback mSaveCallback = new SaveCallback() {
@Override
public void done(ParseException e) {
Toast.makeText(MainActivity.this, "Item saved!", Toast.LENGTH_SHORT).show();
}
};
// Some code omitted!
public void save() {
ParseObject mTask = new ParseObject("Task");
String description = mDescriptionField;
}
}
11 Answers
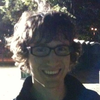
Ben Rubin
Courses Plus Student 14,658 PointsYou left out the second i in "description"
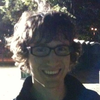
Ben Rubin
Courses Plus Student 14,658 PointsI think it may have been one of the videos before this one where he talks about getting a string from an EditText object. Take a look at http://developer.android.com/reference/android/widget/EditText.html to see the methods that are available to you on mDescriptionField
. You'll need to use one of those methods. After you call that method, you'll then need to call toString()
on the returned value since the returned value is not a string.
Remember, you can't assign something to a string variable that is not a string.

Marc-Oliver Gern
8,747 PointsI had some difficulties as well but figured it out using the eclipse editor:
public void save() {
// Declare object on parse.com
ParseObject todo = new ParseObject("Task");
// Get value from EditText and store it in description (as string)
String description = mDescriptionField.getText().toString();
// Now take the string from description and put it into the ParseObject
todo.put("description", mDescriptionField.getText().toString());
// Save it using methods outlined above
todo.saveInBackground(mSaveCallback);
}
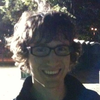
Ben Rubin
Courses Plus Student 14,658 PointsYou can't do String description = mDescriptionField;
because mDescriptionField
is an EditText object, so you can't assign it directly to a string variable. You have to get the text from mDescriptionField
and then assign that to description
. There's a part in the video where Ben talks about how to do this.

Sohale Vu
18,322 PointsHello Ben
Ive tried to figure it out by going back through the video but I could find it. I also tried
'''java String description = (EditText)mDescriptionField; '''
but that didn't work either.

Sohale Vu
18,322 PointsI remember now. I put the getText().toString() extension and it worked out. Thank you for your help.

Sohale Vu
18,322 PointsI don't know why I'm so horribly stuck on this code challenge on part 3 I put down the code below which I believe is right but nothing is working
'''java
import android.app.Activity; import android.widget.EditText; import android.widget.Toast; import com.parse.ParseException; import com.parse.ParseObject; import com.parse.SaveCallback;
public class MainActivity extends Activity {
protected EditText mDescriptionField;
protected SaveCallback mSaveCallback = new SaveCallback() {
@Override
public void done(ParseException e) {
Toast.makeText(MainActivity.this, "Item saved!", Toast.LENGTH_SHORT).show();
}
};
// Some code omitted!
public void save() {
ParseObject mTask = new ParseObject("Task");
String description = mDescriptionField.getText().toString();
mTask.put("descripton", description);
}
}
'''
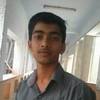
Ajay Maheshwari
Courses Plus Student 6,423 Pointscheck the spelling of description

Sohale Vu
18,322 PointsFar smarter than I sir
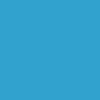
r lomeli
6,000 PointsThanks !
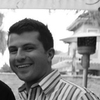
Erin Kabbash
5,210 PointsThanks Marc-Oliver!

MUZ140837 Trust Mubaiwa
5,597 Pointsthis should work:
String description = mDescriptionField.getText().toString();