Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial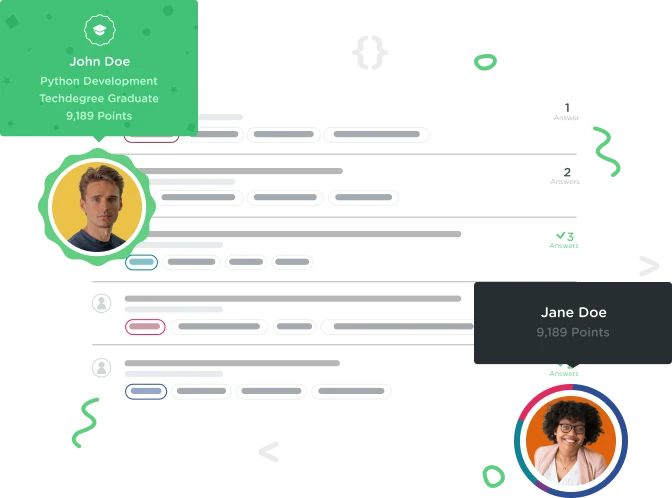
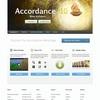
Victor Warner
1,882 Pointssesame
Hey guys I am having a hard time with this challenge, not sure what I am doing wrong.
var secret = prompt("What is the secret password?");
do( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}while(secret === "sesame");
document.write("You know the secret password. Welcome.");
2 Answers
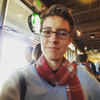
Emmie Cole
3,082 PointsHello!
So your code right now looks like:
var secret = prompt("What is the secret password?");
do( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}while(secret === "sesame");
document.write("You know the secret password. Welcome.");
So the first thing to fix is the way you are declaring the variable secret. You just want to declare it so it exists outside of the loop, but you don't want to trigger the prompt yet. You'll trigger the prompt inside the do...while loop. So let's change that first.
var secret;
do( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}while(secret === "sesame");
document.write("You know the secret password. Welcome.");
So now you've declared the variable outside the loop, but wait until the loop runs to trigger the prompt and set the user's answer in the variable 'secret'. The next thing to fix is the way you are placing the condition right after 'do'. This doesn't make sense because you want to place your curly brackets right after the 'do'. The program will run the code after 'do' regardless of any conditions. Then it will look at the condition after 'while' to see if it needs to repeat that block of code. So first you'll want to delete the initial condition so your code looks like this:
var secret;
do{
secret = prompt("What is the secret password?");
}while(secret === "sesame");
document.write("You know the secret password. Welcome.");
Okay, so now the syntax of the code is right, but the condition in the while loop isn't quite right. If we ran this code the prompt would repeat when the user enters 'sesame' and print 'You know the secret password. Welcome.' whenever the user typed anything else. That's kind of the opposite of what you want, right? So we'll change the condition inside of this while condition to look like this:
var secret;
do{
secret = prompt("What is the secret password?");
}while(secret !== "sesame");
document.write("You know the secret password. Welcome.");
Now the program will know about the secret variable, then open a prompt and set the user's input into that variable. Then it will check if that input matches the string "sesame". If it doesn't match, the code will keep prompting the user and setting the user's input to the variable 'secret' over and over and over again. Once the user's input 'secret' matches the string "sesame" the program will break out of the while loop and continue on to the line that prints the message to the document.

KRIS NIKOLAISEN
54,971 Points1) Declare secret
outside the loop without the prompt. With the existing prompt you'd be prompting the user twice before checking the value of secret
because the code in the do/while loop will execute at least once
2) The syntax you want to use for the loop is as follows:
do {
code block to be executed
}
while (condition);
Here the condition to run is that secret is not equal to sesame
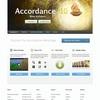
Victor Warner
1,882 Pointsthank you for posting!
Victor Warner
1,882 PointsVictor Warner
1,882 PointsTY very much for the guidance and providing a breakdown of the correct code. ok, my understanding that I need to leave the variable empty, set the var with a prompt for the first line, run it then write a conditional statement for the while loop. though conceptually it makes the idea of creating a var in the global scope and leaving it empty would have never crossed my mind. and how come you cant have a prompt for the var and also in do section, if the condtional statement is true, it does not end the function but goes back to the prompt? If secrect = . sesame then run document.write. It looks like things are out of order when looking at the code.
Emmie Cole
3,082 PointsEmmie Cole
3,082 PointsI'm glad it was helpful! I'll try to answer your questions:
So the reason you can't have the prompt in the var secret AND in the do section is because then the code would trigger the prompt twice. The first time it would set 'secret' to the users input, then it would immediately overwrite 'secret' with the users input from the second prompt. Then it would test to see if 'secret' is equal to "sesame" in the while condition. If the while condition is true it repeats everything inside the do curly braces {}, if the while condition is false it continues to the next line in the code.
I admit that it does look a bit strange, but that is how do-while loops work. I kind of think of it like this:
The treehouse video on do-while loops has a nice animation that walks through how the code runs step by step that really helped me understand it better.