Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial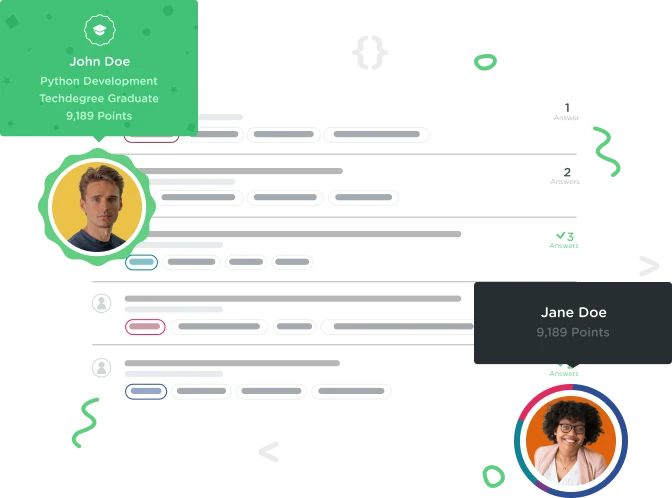
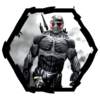
channonhall
12,247 PointsSet the Gravity? How? What?????
How to Set the gravity without android studio?? I'm so confused!
Please help!
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/white" >
<TextView
android:id="@+id/movieTitle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="32sp"
android:text=""
android:layout_centerHorizontal="true"
android:layout_centerVertical="true" />
<ImageView
android:id="@+id/movieImage"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@id/movieTitle" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/movieTitle"
android:layout_centerHorizontal="true"
android:id="@+id/linearLayout">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=""
android:id="@+id/textView"
android:layout_weight="1" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=""
android:id="@+id/textView2"
android:layout_weight="1" />
</LinearLayout>
</RelativeLayout>
3 Answers
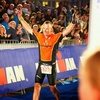
Steve Hunter
57,712 PointsHi Channon,
There's two TextView
objects within the Linear Layout. The first one of these needs its android:gravity
setting to "left"; the second TextView
wants the gravity set to "right".
Some code like, android:gravity=
"left
" would do that. That needs adding into the correct part of the code above.
Let me know how you get on.
Steve.
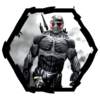
channonhall
12,247 PointsThanks Steve!, Can you help me with another question?
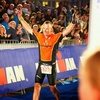
Steve Hunter
57,712 PointsYes, of course.
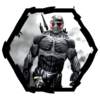
channonhall
12,247 PointsThe Chalenge URL
https://teamtreehouse.com/library/build-a-weather-app/networking/building-an-http-request <
My code
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MovieActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_movie);
// Get some movie information!
String apiUrl = "http://api.rottentomatoes.com/api/public/v1.0/movies.json?apikey=xyz&q=hobbit";
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
try {
Response response = call.execute();
if (response.isSuccessful()) {
Log.v(TAG, response.body().string());
}
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
}
Thanks for helping me steve now I want you're help again...Can you fix this code it has an error.
P.S. I will finaly finish this course!! :D SOOOO EXCITED
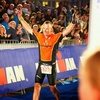
Steve Hunter
57,712 PointsWhat error is it throwing? Is that at runtime or pre-compile?
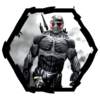
channonhall
12,247 PointsThis is the error in the console/preview
./MovieActivity.java:21: error: cannot find symbol .url(forecastUrl) ^ symbol: variable forecastUrl location: class MovieActivity ./MovieActivity.java:24: error: cannot find symbol Call call = client.newCall(request); ^ symbol: class Call location: class MovieActivity ./MovieActivity.java:24: error: cannot find symbol Call call = client.newCall(request); ^ symbol: method newCall(Request) location: variable client of type OkHttpClient ./MovieActivity.java:26: error: cannot find symbol Response response = call.execute(); ^ symbol: class Response location: class MovieActivity ./MovieActivity.java:28: error: cannot find symbol Log.v(TAG, response.body().string()); ^ symbol: variable TAG location: class MovieActivity ./MovieActivity.java:28: error: cannot find symbol Log.v(TAG, response.body().string()); ^ symbol: variable Log location: class MovieActivity ./MovieActivity.java:30: error: cannot find symbol } catch (IOException e) { ^ symbol: class IOException location: class MovieActivity ./MovieActivity.java:31: error: cannot find symbol Log.e(TAG, "Exception caught: ", e); ^ symbol: variable TAG location: class MovieActivity ./MovieActivity.java:31: error: cannot find symbol Log.e(TAG, "Exception caught: ", e); ^ symbol: variable Log location: class MovieActivity 9 errors
Gtg see you tomorrow!
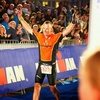
Steve Hunter
57,712 PointsYou've used forecastUrl
- use apiUrl
instead and that's good to go.
The variable forecastUrl
is from the project you're working on, not the challenge. In the challenge, there's a variable declared for this at the top of the onCreate()
method - it is called apiUrl
and it is set to some movie information; pass that in to the url()
method with url(apiUrl)
.
Also, you've added a load of code that's not needed. Delete the Call
and the whole try/catch
block - they're not required. The challenge just wants you to create an instance of OkHttpClient
and a Request
.
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(apiUrl)
.build();
Steve.
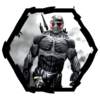
channonhall
12,247 PointsThanks Steve! I Finaly done it!... It's complete..Completed! Wooo Thanks again!
channonhall
12,247 Pointschannonhall
12,247 PointsI'm not that confused but.....Setting the gravity without android studio. I'm like WHAT??