Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial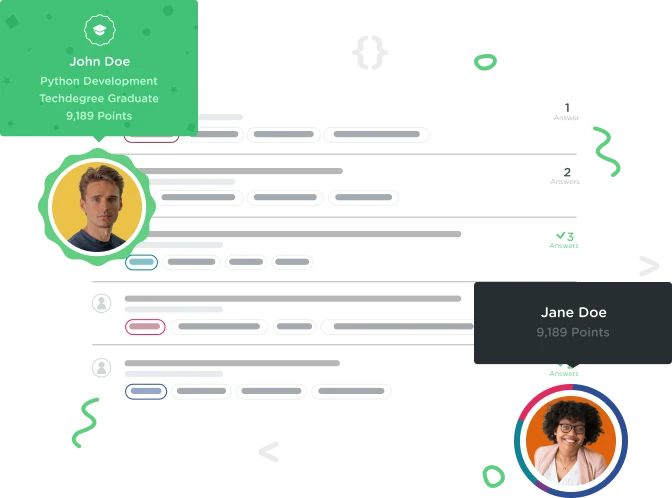
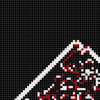
meng chen
378 PointsSet value in activity based on button click from another
Hi, Here are what I'm trying to do: there are three buttons that leads to the same second activity. However, depending on which button is clicked, a value in the second activity will be set differently. I'm wondering what is the most efficient way of doing so. thanks
3 Answers

Ben Jakuben
Treehouse TeacherYou will want to add a piece of data to the Intent you use when you tap on the button. Ernest Grzybowski just posted some helpful code in this other forum post about a similar issue. You can also find more details about passing data with Intents in the Easy Sharing with Intents video.
Now what data should you pass? You could pass a simple String or number to indicate which button. I would recommend making a publicly available constant in your first Activity that you could reference in the second Activity. That way you could make certain you are referencing the same keys and values. Here's a quick example:
FirstActivity.java
public static final String BUTTON_KEY = "BUTTON_KEY";
public static final String BUTTON_VALUE = "first_button";
...
intent.putExtra(BUTTON_KEY, BUTTON_VALUE);
SecondActivity.java
String buttonValue = bundle.getString(FirstActivity.BUTTON_KEY);
if (buttonValue.equals(FirstActivity.BUTTON_VALUE)) {
// do something for the 1st button...
}
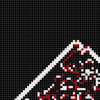
meng chen
378 PointsI have 3 activities structured like such: A > B > C A is the main screen B is the profile list with a button "add profile" C is the page for enter profile information
putExtra was used in C to pass information to B for display, and it seems to work properly. However, when I click "Back" in B to A, and go back to B. No information were displayed, if I enter C and go back to B again, the information entered will come back. I wonder why is it behaving this way

Ben Jakuben
Treehouse TeacherA few brief points (maybe I or someone else can add more detail later, but I'm short on time!):
Check out startActivityForResult. If you start C "for result" then you can pass back the data to B as a result of what was done on C.
How is your data in B being stored/loaded? It sounds like you're losing data because of the Activity lifecycle. When B is paused (the onPause() event), you should store the data in something like SharedPreferences and then fill it back in in the onResume() method. That's one thing you could try, at least.