Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial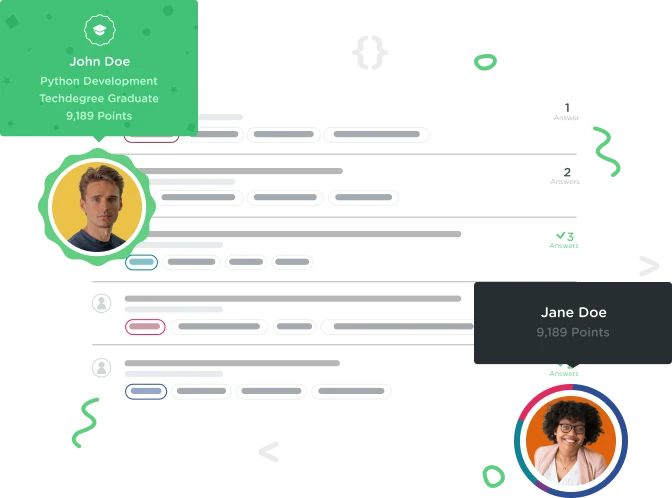
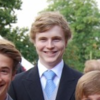
jack hamilton
5,609 PointssetAdapter does not work with "extends ActionBarActivity". Within Android Studio.
So I'm using android studio and I when I created the EditFriendsActivity it populated it with ActionBarActivity. I did try to change it although that threw up a whole load of error.
This is the code for the class file. I've highlighted the error below.
The Error name is "Caanot resolve method 'setAdapter(android.widget.ArrayAdapter<java.lang.String>)' "
Check below for the working answer
4 Answers
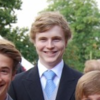
jack hamilton
5,609 PointsEDIT - Between myself and Clement we managed to get a working version for this. The code below is WORKING.
Make sure to update it to your package as well as change the name back to EditFriendsActivity.
package uk.co.jackdh.tapchat;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.HeaderViewListAdapter;
import android.widget.ListAdapter;
import android.widget.ListView;
import com.parse.FindCallback;
import com.parse.Parse;
import com.parse.ParseException;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import com.parse.SaveCallback;
import java.util.List;
public class EditContactsActivity extends ActionBarActivity {
public static final String TAG = EditContactsActivity.class.getSimpleName();
protected List<ParseUser> mUsers;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
private ListView mListView;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_edit_contacts);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
getListView().setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
if (getListView().isItemChecked(position)) {
//add friend
mFriendsRelation.add(mUsers.get(position));
} else {
//remove friend
mFriendsRelation.remove(mUsers.get(position));
}
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e == null ) {}
}
});
}
});
}
protected ListView getListView() {
if (mListView == null) {
mListView = (ListView) findViewById(android.R.id.list);
}
return mListView;
}
protected void setListAdapter(ListAdapter adapter) {
getListView().setAdapter(adapter);
}
protected ListAdapter getListAdapter() {
ListAdapter adapter = getListView().getAdapter();
if (adapter instanceof HeaderViewListAdapter) {
return ((HeaderViewListAdapter)adapter).getWrappedAdapter();
} else {
return adapter;
}
}
@Override
protected void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.orderByAscending(ParseConstants.KEY_USERNAME);
query.setLimit(1000);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> parseUsers, ParseException e) {
if (e == null) {
//success
mUsers = parseUsers;
String[] usernames = new String[mUsers.size()];
int i = 0;
for (ParseUser user : mUsers) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(EditContactsActivity.this, android.R.layout.simple_list_item_checked, usernames);
getListView().setAdapter(adapter);
addFriendCheckMarks();
} else {
//error
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(EditContactsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
private void addFriendCheckMarks() {
mFriendsRelation.getQuery().findInBackground( new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> parseUsers, ParseException e) {
if (e == null) {
//List returned look for match.
for (int i = 0; i < mUsers.size(); i++) {
ParseUser user = mUsers.get(i);
for (ParseUser contact : parseUsers) {
if (contact.getObjectId().equals(user.getObjectId())) {
getListView().setItemChecked(i, true);
}
}
}
} else {
Log.e(TAG, e.getMessage());
}
}
});
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
Then Clean building the project.
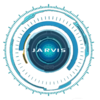
jenyufu
3,311 PointsI used this gist: https://gist.github.com/anonymous/fcb64927625122604f5b that you provided and it worked! I used ctrl+f to change 'contact' to 'friend' too, not just changing EditContractsActivity to EditContactsFriends
change from yours:
for (ParseUser contact : parseUsers) {
if (contact.getObjectId().equals(user.getObjectId())) {
getListView().setItemChecked(i, true);
to
for (ParseUser friend : parseUsers) {
if (friend.getObjectId().equals(user.getObjectId())) {
getListView().setItemChecked(i, true);

Clement Besson
83 PointsHey Jack, I tried your solution but don't you loose the your navigation bar by doing such? When I did what you suggested, I couldn't see the "up" button to navigate back to the MainActivity...
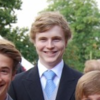
jack hamilton
5,609 PointsHmm you're right, at the time I just used the back button on the phone. I'm not sure how else to compete this without doing it like this though. Any ideas?

Clement Besson
83 PointsFrom what I saw online, we are supposed to use ListFragment instead of ListActivity. I am still trying to understand how to implement the fragment on the EditFriends activity
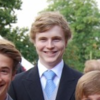
jack hamilton
5,609 PointsHmm okay So a little bit further on I ran into this problem again but this time we need the action bar to show up, so I'm going to have to spend some time working on this. Let me know if you make any breakthroughs and I'll do the same.
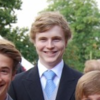
jack hamilton
5,609 PointsClement! I pretty sure I've done it. Check this out, https://gist.github.com/anonymous/ef4110483c3d55b2d5fa
What I had to do was implement Listview myself then on line 118 I created my own on click listener. It's quite ugly but it works.
If you want to use it make sure to change it from EditContactsActivity to EditFriendsActivity ( I changed it due to preferring using contacts instead of friends)!
Let me know if it works.
Edit* I swear I must be going crazy. I had it working correctly and now it has just given up and not working any more.

Clement Besson
83 PointsHey good job dude! it works!! Thanks a lot for sharing!
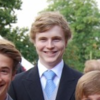
jack hamilton
5,609 PointsNo way! It's working for you, thats great can't believe it's stopped working for me!
Did you change anything after copying the code?!

Clement Besson
83 PointsOk so the tab bar works fine for me the issue is that what I dont have the proper arguments for your method onItemClick line 120 . Can you tell me what should my arguments be thus I can send you my code back to check if it works for you?
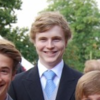
jack hamilton
5,609 PointsHmm I don't provide it any arguments. I literally use it as that. So it's not working now for you neither?

Clement Besson
83 PointsWhen I click a user, it doesnt change the relation on the back end. Is that the problem that you're having?
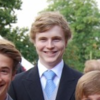
jack hamilton
5,609 PointsYeah that was the problem I had. It was werid as I was working succesffully on the back end as well for around 5 minutes then just stopped.

Clement Besson
83 PointsIm debugging it right now, your onItemClick method never gets called. Im trying to figure it out
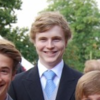
jack hamilton
5,609 PointsI think I've fixed it I moved that line into the onCreate. As I believe that was the problem we were creating a new listener each time we click the check which we didn't need to do.
Check this out.
https://gist.github.com/anonymous/fcb64927625122604f5b
It's currently working for me let me know if it works for you !

Clement Besson
83 PointsDAMN it works. Good job sir
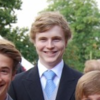
jack hamilton
5,609 Pointshaha, I think I learned more android trouble shooting this than in the entire tutorial! I just hope this helps some more people.
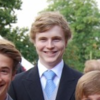
jack hamilton
5,609 PointsYo Clement,
So I was thinking that answer is really ugly so I looked up a better way to do it and I found one.
Basically you need to create a Blank Activity with a fragment.
The blank activity will hold the action bar and then the fragment will hold the list.
The Java file for this looks like this. https://gist.github.com/anonymous/dda385e06e48a6a9e013

rtrind
6,358 PointsYour comments saved me a lot of trouble. Thanks!
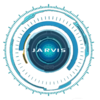
jenyufu
3,311 PointsNotice to all the people doing the lesson: Finish up the whole unit Relating Users in Parse.com and THEN come back and replace your EditFriendsActivity with the one jack hamilton provided.
J C
5,601 PointsJ C
5,601 PointsReplace Ben's line:
setListAdapter(adapter);
with the following:
friends_search_list is just the ID that my ListView has, You will want to replace friends_search_list with your ListView's ID.