Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial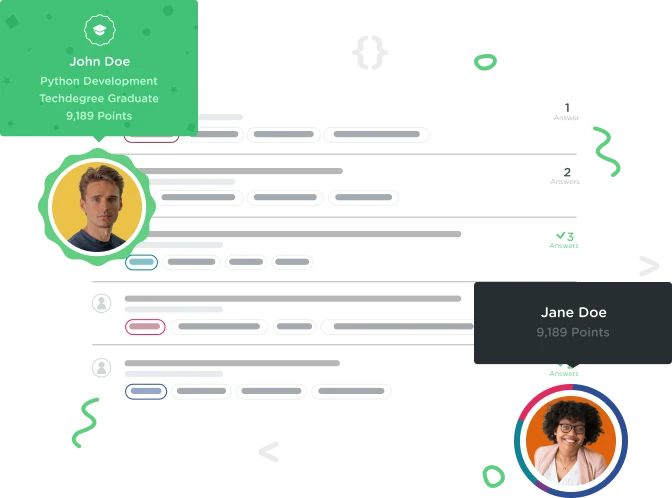

Leo Marco Corpuz
18,975 PointsSets challenge
I get an error saying something else needs to be imported.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import com.example.BlogPost;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public getAllAuthors(){
Set<String> authors=new TreeSet<String>();
authors.add(BlogPost.getAuthor());
return authors;
}
}
1 Answer
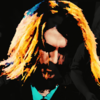
Justin Horner
Treehouse Guest TeacherHello Leo,
You're close to passing this one. Here are the issues I've found.
The first issue I noticed is that your getAllAuthors method is missing a return type. The challenge asks that you return a java.util.Set
of all the authors. So first step is to add the return type like this.
public Set<String> getAllAuthors()
{
...
}
Next, when adding authors to the set, you're trying to access the getAuthor method from the BlogPost
class instead of looping through mPosts to get the blog post objects. The challenge asks that we loop over all the blog posts so that we can call getAuthor to return all of the authors. That would look something like this.
for (BlogPost post : mPosts)
{
authors.add(post.getAuthor());
}
I hope this helps. Please let me know if you have any other related questions. Happy coding π
Leo Marco Corpuz
18,975 PointsLeo Marco Corpuz
18,975 PointsThanks! But I donβt understand how mPosts is referencing the BlogPost class when itβs under the Blog class.
Justin Horner
Treehouse Guest TeacherJustin Horner
Treehouse Guest TeacherYou're welcome!
Inside the Blog class, the mPosts variable has a type of List of BlogPost objects (List<BlogPost>) that is set to the posts that are passed in via the Blog constructor.
The Blog has this list of BlogPosts because they are passed to it when a new Blog object is created. I hope this helps clarify the relationship between the two classes and why we need to loop through the BlogPost objects in the List (mPosts).