Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial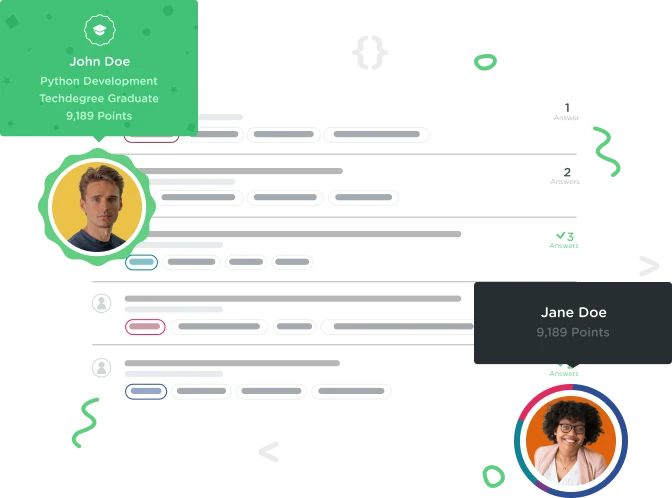

Michael Revy
3,882 Pointssets.py
Didn't get the right output from 'covers' I pass 'covers' a set of topics, I return a list of courses that have the topic(s). I do not return duplicate courses - but even if I do, my solution is still wrong.
I am sure I have read the problem wrong.. any clues / more elegant solution appreciated
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(targetC):
xList = []
try :
xcheck = set({targetC.pop()})
except KeyError :
return xList
while xcheck:
for key in COURSES.keys():
if xcheck.isdisjoint(COURSES[key]) == False:
if key not in xList :
xList.append(key)
try :
xcheck = set({targetC.pop()})
except KeyError :
break
return xList
# Testing Inputs to covers
targetC = ({"Python","functions","Medieval Histroy"})
xList = covers(targetC)
print(xList)
targetC = ({"Python","HTML"})
xList = covers(targetC)
print(xList)
targetC = ({"Python"})
xList = covers(targetC)
print(xList)
targetC = ({""})
xList = covers(targetC)
print(xList)
print(type(xList))
1 Answer

Mike Wagner
23,559 PointsMy brain may be fooling me but your code appears to do what the Challenge asks for, but I'm assuming that it tests with some interesting edge cases that you're not accounting for in some way or another. I'm not entirely sure why your code doesn't pass, since I tested it in my own environment and it appears to work as expected. Alternatively, it could be quite picky about the way it expects to see a problem solved and you just happened to do it in a way that was beyond the simple program's knowledge.
In either case, a simple way to solve your issue and pass that section of the challenge would be to remove all the interesting set()
operations and your xCheck, then to change your for-loop to be something like
for key, value in COURSES.items():
if value & targetC:
if key not in xList:
xList.append(key)
which will iterate over the 'key, value
' pair for each item in COURSES
, checking the value
against your passed in targetC
and, if it matches, it will then append the key
to your xList
, should it not already exist. Essentially, this would seem to do everything you originally tried to do, only in a slightly more common and readable way.
Michael Revy
3,882 PointsMichael Revy
3,882 PointsVery interesting! If I understand .. for each key, you do a bitwise compare of value for that key with targetC. Bitwise compare returns True if there is something in the set created by the compare (&). Then you simply append the key if it is not already in the key list. Use of Bitwise compare (&) is very new to me. Thanks
Mike Wagner
23,559 PointsMike Wagner
23,559 PointsMichael Revy - You should be able to, instead of the bitwise operator, use the logical
and
to achieve the same result if you're more comfortable with that. Essentially, you are correct, though.