Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial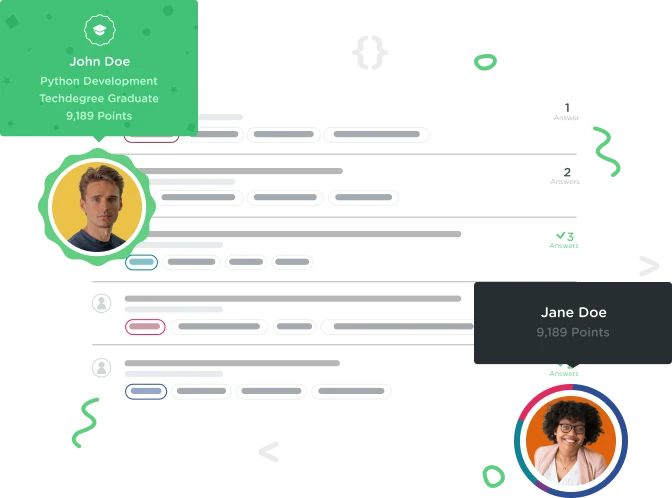

Hanna Han
4,105 PointsSets.py challenge task 2
I don't know what is wrong with my code in task 2. Could you please explain?
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(string):
course_list = []
for key, value in COURSES.items():
if string.intersection(value):
course_list.append(key)
return course_list
def covers_all(string1)
course_list1 = []
for keys, values in COURSES.items():
if len(string1 & values) > 0:
course_list1.append(keys)
return course_list1
2 Answers
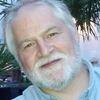
Jeff Muday
Treehouse Moderator 28,717 PointsYou are soooo close! You have the right concept, but only two little tweaks required.
- You were missing a colon on the covers_all declaration
- You were appending courses to the course list that only had a partial match.
This is an easy fix... simply check the length of the intersection with the length of the input set.
Good luck with Python, it's a fun and useful language!
def covers_all(string1):
course_list1 = []
for keys, values in COURSES.items():
if len(string1 & values) == len(string1): # make sure the size of the input matches the intersection
course_list1.append(keys)
return course_list1
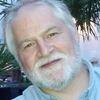
Jeff Muday
Treehouse Moderator 28,717 PointsWhen I get stuck on a challenge, I will write the challenge code in an external IDE that has better debugging support and syntax highlighting. Sometimes those small errors are the only thing between "Bummer" and "Success"!
My favorite is WingWare's IDE (Wing Personal). It is responsive and supports great debugging modes. https://wingware.com/downloads/wingide-personal
An even stronger choice for a Python learner is PyCharm EDU (strong debugger and PEP-8 lint checker)-- it provides subtle suggestions to make your code better fit the PEP-8 standard. The only down-side is that it is more insistent on doing things the "Pycharm way" https://www.jetbrains.com/pycharm-edu/
The other is Visual Studio Code-- though I think this is slightly difficult to set up for a student, some bloggers I follow are beginning to swear by it as the fastest and most flexible environment. The downside is more setup and a little bit harder to add new virtual environments. https://code.visualstudio.com/
Hanna Han
4,105 PointsHanna Han
4,105 PointsThank you fo ther clear answer!