Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial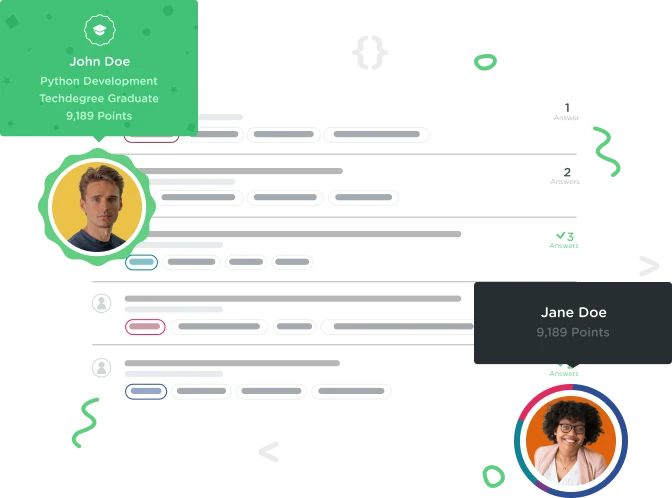

newwebcoder
13,061 PointssetState with "+=" instead of just "+"
Hello everyone,
I don't really understand why we are using "+=" instead of just "+" at 2:20?
Works:
handleScoreChange = (index, delta) => {
this.setState(prevState => ({
score: (prevState.players[index].score += delta),
}));
};
Doesnβt work:
handleScoreChange = (index, delta) => {
this.setState(prevState => ({
score: (prevState.players[index].score + delta),
}));
};
Before we reworked this function to be for both(increment and decrement), we were updating the state with just "+" or "-" like so:
incrementScore = () => {
this.setState(prevState => ({
score: prevState.score + 1
}));
}
decrementScore = () => {
this.setState(prevState => ({
score: prevState.score - 1
}));
}
We were not doing something like score: prevState.score += 1 or score: prevState.score -= 1. Could you please explain what has changed here?
1 Answer
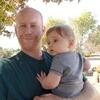
Jordan Kittle
Full Stack JavaScript Techdegree Graduate 20,148 PointsUpdate: Wow I feel stupid. I jumped straight to questions when all this has been answered in Teacher's notes already. In any case, if anybody does what I just did the answer is there.
I am struggling to wrap my head around what's happening here as well. I have a guess but I'm still confused. When we updated the state in the Counter component, we used a key: value statement: score: prevState.score + 1
. In that case, the key 'score' is unique to each player.
Here in the App component, I can't understand how the key of score:
references any player, but I can see how the value we are using to set it prevState.players[index].score += delta
does reference the specific player. I think that is why we use += here, we are using the right side of the key:value statement to set state specific to a player, not the left side like before. I don't really understand yet why we wouldn't use the left side of the key:value pair in the App component to address the specific player. I wish somebody could explain this to me.
Update: I found every answer in this thread: https://teamtreehouse.com/community/is-it-safe-to-mutate-prevstate-ie-prevstateplayersindexscore-delta
It seems this method of updating state might be in question, and the key in the key:value pair is not specific to the player like I thought:
this.setState( prevState => {
// modify previous state
return {
anyNameWillWork : prevState.players[index].score += delta
};
});
In the code above, notice the key "anyNameWillWork." The state is being changed on the right side (the value), and while it is updating the DOM correctly, this thread suggests it is not the best way to do this, and we should be updating the players object in the App component (without mutating prevState) instead. Maybe the correct way was a little too complicated, but I'm wondering if this video just taught me how to do something I shouldn't.