Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial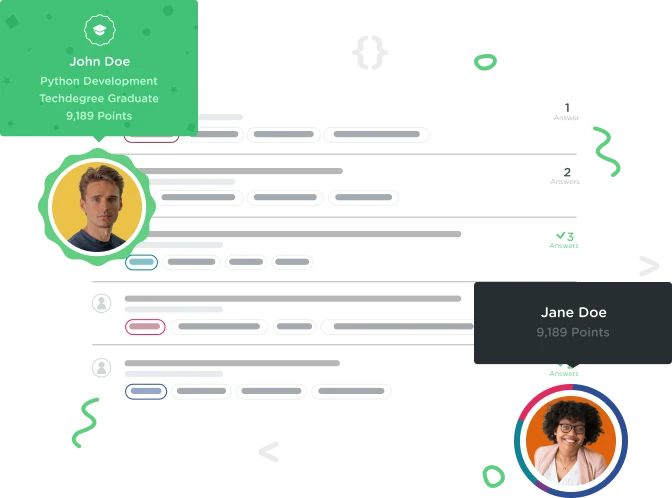

Ericka Erickson
9,982 Pointssetter method is returning the wrong value? how to access get level method
how do i access the get level method
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
get major (){
return this._major
}
set major(major){
this._major = major;
if(this.level == 'Senior '|| this.level=='Junior'){
return major;
}else {}
return 'None';
}
}
var student = new Student(3.9, 60);
2 Answers
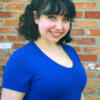
Bella Bradbury
Front End Web Development Techdegree Graduate 32,790 PointsHello Ericka!
This is a really tricky challenge, I definitely had some issues with it when I was completing this course. Let's walk through the challenge together! For the purposes of not inflating this answer more, we're only going to be taking a look at the code within the 'added code' block I've outlined:
class Student {
constructor(gpa, credits){...}
stringGPA() {...}
get level() {...}
// start of added in code
get major (){
return this._major
}
set major(major){
this._major = major;
if(this.level == 'Senior '|| this.level=='Junior'){
return major;
}else {}
return 'None';
}
// end of added in code
}
var student = new Student(3.9, 60);
Firstly, you currently have both a get
and set
method for major()
. This challenge is only looking for a set major()
method so the first step is to delete the get major()
function! After doing so your code should look like:
// start of added in code
set major(major) {
this._major = major;
if (this.level == 'Senior ' || this.level == 'Junior') {
return major;
} else {}
return 'None';
}
// end of added in code
Here is where the instructions fails us a little bit. We're NOT looking to immediately set this._major
to the major
value. Instead we want to only set this._major = major
if the student is a 'Senior' or 'Junior'. Let's move that line into the if...
statement:
// start of added in code
set major(major) {
if (this.level == 'Senior ' || this.level == 'Junior') {
this._major = major;
return major;
} else {}
return 'None';
}
// end of added in code
Next we'll address what should happen if the student is NOT a 'Senior' or 'Junior' using the else...
statement! The first thing to do is fix the syntax error. We need to make sure our else...
code is INSIDE of the curly brackets {}
. Then we need to set this._major
to equal 'None'
:
// start of added in code
set major(major) {
if (this.level == 'Senior ' || this.level == 'Junior') {
this._major = major;
return major;
} else {
this._major = 'None';
return 'None';
}
}
// end of added in code
The last thing to do is address the return statements. We need to make sure that we're returning the value of this._major
instead of just the values themselves:
// start of added in code
set major(major) {
if (this.level === 'Senior' || this.level === 'Junior') {
this._major = major;
return this._major;
} else {
this._major = 'None';
return this._major;
}
}
// end of added in code
And there you go! The code above when substituted for the original 'added code' block will have the challenge completed! You were really close and had a lot of the right ideas 👏 Hopefully this step by step will help you to understand the challenge better!
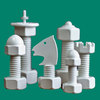
Steven Parker
229,783 PointsI saw your duplicate question first and answered there, but I've moved my answer here to make it possible for you to delete the duplicate (since answered questions cannot be deleted).
The message about "returning" the wrong value is a bit misleading, since setters don't actually return anything. Instead, it's the setter's job to change something inside the class, and in this case it's the _major backing property.
A few other issues:
- there's a space in the string
'Senior '
, so it will never match - you might want to test the level before setting the _major value
- be sure to also set _major to the correct value when the test is not true
- this challenge does not ask you to create a getter method
Steven Parker
229,783 PointsSteven Parker
229,783 PointsAs I mentioned in my (comparatively terse) answer, setter methods do not need to return anything. So the "return" statements can be removed from this code.