Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial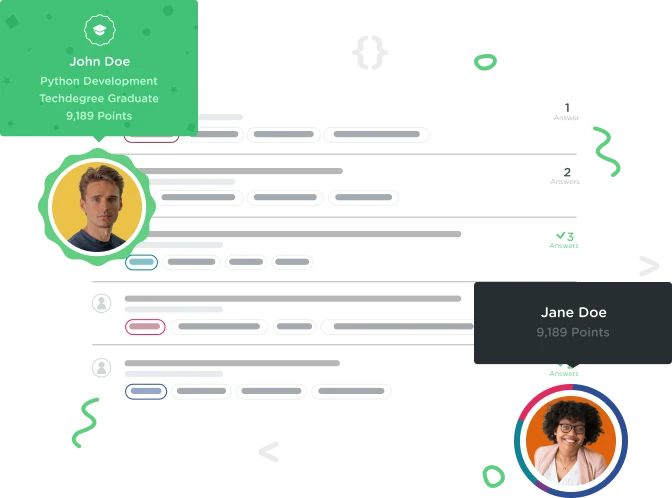
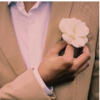
Muaaz Matwadia
Full Stack JavaScript Techdegree Graduate 19,327 Pointssetter solution
Can you find the error?Thanks
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(p){
this._major = p
if(this.level ==='Junior' || 'Senior'){
this._major = p
}else{
this._major = 'none'
}
}
}
var student = new Student(3.9, 60);
1 Answer
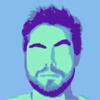
Cameron Childres
11,820 PointsHi Azzie,
The error is in your if condition:
if(this.level ==='Junior' || 'Senior')
Each side of the logical OR operator is evaluated separately. The left side will only be true if this.level is 'Junior' but the right side will always be true since 'Senior' is not being compared to anything and strings are considered to be truthy. This if statement will always run since one side of the OR will always evaluate as true.
Make each side a complete comparison and you should pass the challenge:
if(this.level ==='Junior' || this.level === 'Senior')