Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial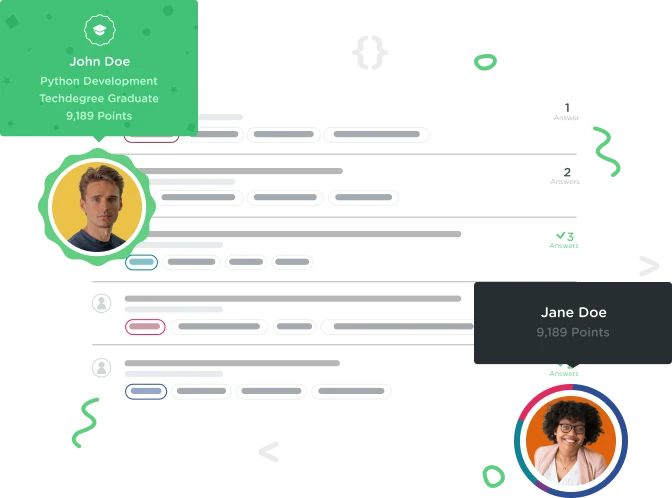
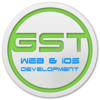
George S-T
6,624 PointsSetters, Getters, Properties and Interface's ?
Ive completed the Objective-C basics part of the track, but there are a few things Im confused with. It would be really helpful if somebody could simplify it all down so I can grasp a basic idea.
What is this idea of Setters and Getters? What do they do?
What is a property? Why do we need them and when do we use them?
What is an interface? What are they used for?
I have of course, watched the videos many times, but I believe that he doesn't explain these things all too well.
1 Answer

eirikvaa
18,015 PointsSetters and getters - basic idea and what they do
Objects have instance variables that we can set. An object of type rectangle may have «width» and «height» as two of its instance variables. We want to access these instance variables to either set them to some value or get the value in our program.
You can either access the instance variables directly or through what is called accessor methods. When you access the instance variable directly, there is no «middle man» - it’s just a direct line from you to the instance variable. When you use accessor methods, you call a method that either sets or gets the value and you don’t touch the instance variable yourself. Setter and getter methods are collectively known as accessor methods.
Properties - why we need them and when we use them
If you have many accessor methods, your classes would be cluttered. If you wanted to set and get the value of 10 instance variables, you would have 20 accessor methods. That's a lot. Instead of doing it this way, you declare what is known as a @property. The next line is important: A @property is just a getter and a setter. You get some things for free when you declare a @property:
- An instance variable named like the name of your property (example: "property")
- A getter method in the following format: - (Type)property
- A setter method in the following format: - (void)setProperty:(Type)property
This is a great way to reduce clutter and have Xcode take care of what would have been repetive coding. This is generally why we want to use a @property.
When do we use them, you ask? That is a tough question. Many are debating it and there is no definitive answer. Some only use properties and some use both (I can't think of anyone that uses only instance variables (in Objective-C)). I like to rely solely on properties. It's just easier that way. I hope this clears things up regarding properties and setter/getter methods.
What is an interface and what are they used for
Well, you are not very specific, but I'm going to assume that you're asking about the @interface file.
There are two types of class files in Objective-C: .h file and .m file. The .h file is known as the header file (hence the .h) and the .m file is known as the implementation file. We usually put all our public information about a class in the header file and our private information and implementation in the implementation file. Subclasses can therefore see all our methods and properties in the header file, but they can't see anything in the implementation file. That's just for the class to use, no one else. Does this clear things up for you? Feel free to ask more.
George S-T
6,624 PointsGeorge S-T
6,624 PointsThanks so much. One last thing, what does it mean by one file being private and one public? Surely when someone uses your program, they don't see the code anyway.
Also, does it matter what you put in each file?
eirikvaa
18,015 Pointseirikvaa
18,015 PointsTo understand the difference between files that hold public information as opposed to files that hold private information, let's consider the following:
First we create a Rectangle class. When we create this class, we get the .h and the .m file. Then we create a Square class and also get a .h and a .m file. Since a square is a rectangle with four sides of equal length, we set that the Square class is a subclass of the Rectangle class.
Now let's declare two properties in the Rectangle class - the width and the height. If we put these two properties in the .h file (which holds the public information), subclasses can access these two properties and set or get them. Since Square is a subclass of Rectangle (an of course has a width and a height), the Square class can use these two properties. If you put the properties in the .m file, they wouldn't be visible to anyone other than the Rectangle class.
The public vs private is an important aspect of object-oriented programming. If something is only used inside your own class, don't make it public. Other classes don't need to see it. The Square class needs the width and height, so make these properties public. So yes, it matters what you put in each file.
I hope this clears things up for you. If not, ask more.