Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial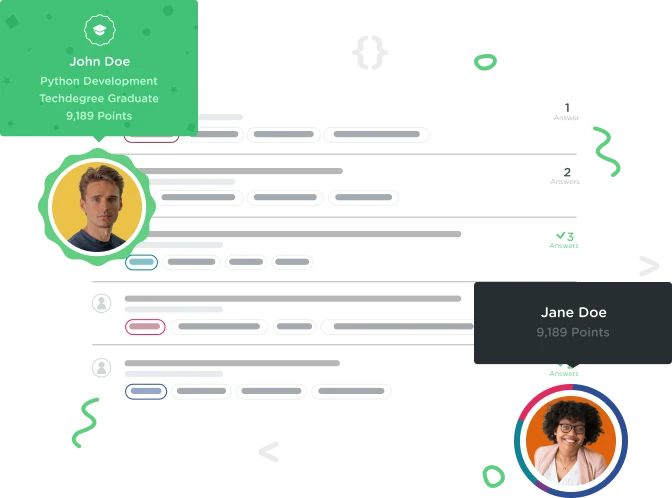
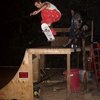
Evan Payne
16,564 PointsSetting a property in the constructor
I understand that we're using an interface for the navigation property to avoid tying ourselves to a concrete implementation...kind of.
My experience with interfaces is that they give us flexibility by giving us the option to pass a variety of objects that implement that interface.
My question: by immediately setting the navigation property to List<T> in the constructor, doesn't that defeat the purpose of the interface? At what point, in practice, would we ever have the opportunity to use a different type that uses ICollection<T>? I could understand if we passed some type of object that implements ICollection to the constructor...like maybe we could pass an array, list, enumerable, etc to instantiate the object and set the navigation property, but we don't. We immediately set it to List<ComicBook>. Why not just use List<T> in the navigation property if we're going to instantiate it that way every time?
Thanks a bunch!
1 Answer
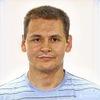
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsI actually come from Java, so that may be not really right, but I try my best.
Yes ICollection
is an interface. But we cannot write
// wrong, won't work
public Series {
ComicBooks = new ICollection<ComicBook>()
}
That won't work. Because for every interface we have to provide implementation in the end.
In the way he wrote his code we want to say roughly: "Hey I know that in the end we will use List<ComicBook>, but because we define this List through ICollection, List cannot use his own methods, he can use only methods that are in ICollection"
We can say that List
is implemented through interface. It is very common technique in OOP.
BUT if you decide to
Why not just use List<T> in the navigation property if we're going to instantiate it that way every time?
Then you cannot switch to new implementation by changing one line, because it can happen that some methods that are in List
they are no longer in LinkedList
PS
There are ton of resources out there, try googling.
Here is one of the examples
http://stackoverflow.com/questions/383947/what-does-it-mean-to-program-to-an-interface
And one more note
It is does not matter what we use in Constructor since we will be using ONLY methods of an interface and not the concrete implementations. In the reality we instantiate ICollection in constructor so that later we don't have NullPointerException
, but always have empty Collection. This is important for Database. I think.
And like I sais before later on if you decide to switch to LinkedList. It is very common.
You can just say
ICollection<ComicBook> books = Series.ComicBooks;
List<ComicBook> list = new List<string>(books);
LinkedList<ComicBook> linkedList = new LinkedList<string>(books);
This should be valid code, or at least concept, i didn't check syntax.
That means EVERY time you ask for ComicBooks from Series, you get ICollection
. You never get List
That is why we have flexibility.
If we define List<ComicBook>
then it won't work.
Evan Payne
16,564 PointsEvan Payne
16,564 PointsAlexander, thank you for your detailed response. Your last point is what I was confused about. Thank you so much!
Kevin Gates
15,053 PointsKevin Gates
15,053 PointsVery well written and explained. Thanks!