Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial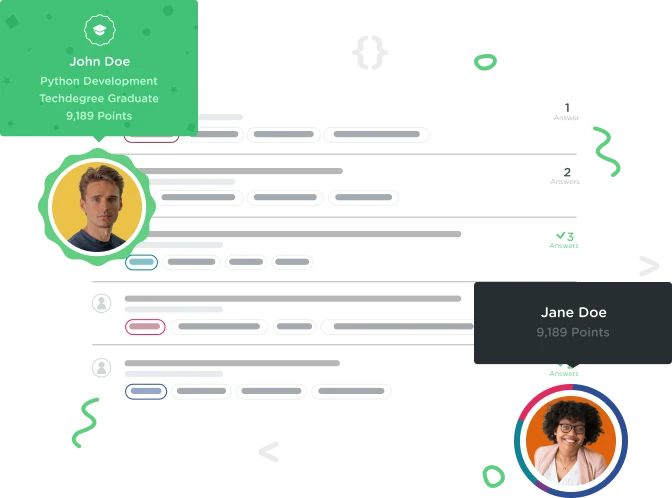
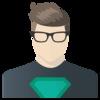
Richard Duncan
5,568 PointsSetting default values to variables inside functions
I'm wondering if anyone can explain the pro's/con's of the following methods for setting default values to variables in javascript functions?
Example a throws a warning in the JSBIN editor of "Expected an assignment or function call and instead saw an expression."
Obviously c is the most succinct. b is a variation on the method taught here on teamtreehouse.
var a = function(data) {
void 0 === data && ( data = 20 );
return data;
};
var b = function(data) {
data = typeof data === "undefined" ? 30 : data;
return data;
};
var c = function(data) {
data = data || 40;
return data;
};
1 Answer
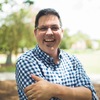
Dave McFarland
Treehouse TeacherThe last one is the best:
var c = function(data) {
data = data || 40;
return data;
};
It's the most succinct and easiest to understand: basically using the || (or) operator you assign the first value if its defined OR the second one if the argument is not defined. This is the most common pattern I see for default value assignment. Any of them work, but I think because option c is shortest, and it's a commonly used convention among JavaScript programmers, it's the better choice.
Dino Paškvan
Courses Plus Student 44,108 PointsDino Paškvan
Courses Plus Student 44,108 PointsOf course, that particular implementation can be problematic if your function really needs to be able to accept a falsy value such as
0
orfalse
or""
(empty string). In that case you will have to check whether your parameter isundefined
if you want to assign a default value to it.Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherGreat advice as usual Dino Paškvan
Yes, if you're expecting (or allow) a false value like
0
orfalse
, you should test forundefined
instead. I would write it in a way that's a little more wordy but easier to read than example (b) above:Dino Paškvan
Courses Plus Student 44,108 PointsDino Paškvan
Courses Plus Student 44,108 PointsIt might also be worth a mention that ES6 will allow for default parameters like this:
This is currently only available in Firefox.
Richard Duncan
5,568 PointsRichard Duncan
5,568 PointsYes I actually found that in testing myself that if you pass 0 into the c function then it returns 40. So that answers that for C.
+1 for ES6 specification Dino Paškvan.
Thanks Dave McFarland I'm comfortable with the use of the ternary operator but I can see why the b variant is taught here.