Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial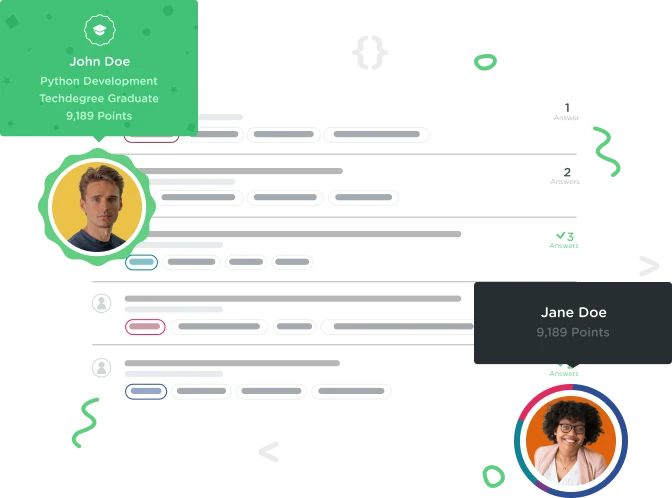

Kenneth Phillips
Courses Plus Student 10,188 PointsSharedPreferences
It throws this error:
./MainActivity.java:21: error: method getBoolean in class SharedPreferences cannot be applied to given types; mSharedPreferences.getBoolean(KEY_CHECKBOX); ^ required: String,boolean found: String reason: actual and formal argument lists differ in length 1 error
Honestly the whole getBoolean() idea has confused me. I looked up the documentation and some examples but that didn't help much. I'd appreciate any help.
import android.view.View;
import android.os.Bundle;
public class MainActivity extends Activity {
private static final String PREFS_FILE = "com.teamtreehouse.sharedpreferencesapp.preferences";
private static final String KEY_CHECKBOX = "key_checkbox";
private SharedPreferences.Editor mEditor;
private SharedPreferences mSharedPreferences;
public CheckBox mCheckBox;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mSharedPreferences = getSharedPreferences(PREFS_FILE, Context.MODE_PRIVATE);
mEditor = mSharedPreferences.edit();
mSharedPreferences.getBoolean(KEY_CHECKBOX);
mCheckBox.setChecked(true);
mCheckBox = (CheckBox) findViewById(R.id.checkBox);
}
@Override
protected void onPause() {
super.onPause();
if (mCheckBox.isChecked()) {
mEditor.putBoolean(KEY_CHECKBOX, true);
mEditor.apply();
}
}
}
1 Answer

Ben Deitch
Treehouse TeacherHey Kenneth!
Not sure if you've solved this yet, but 'getBoolean' actually has two parameters. The 2nd parameter is just a default value in case the specified SharedPreference doesn't exist yet.
mSharedPreferences.getBoolean(KEY_CHECKBOX, true);
Kenneth Phillips
Courses Plus Student 10,188 PointsKenneth Phillips
Courses Plus Student 10,188 PointsSo sorry it took so long to respond. When I put this in it says that Task Two is no longer passing.
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherAh, you also need to move the mCheckBox initialization to before we start using it :)
This works for me:
You'll also want to change your 'onPause' function to store whether or not 'mCheckBox' is checked: