Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial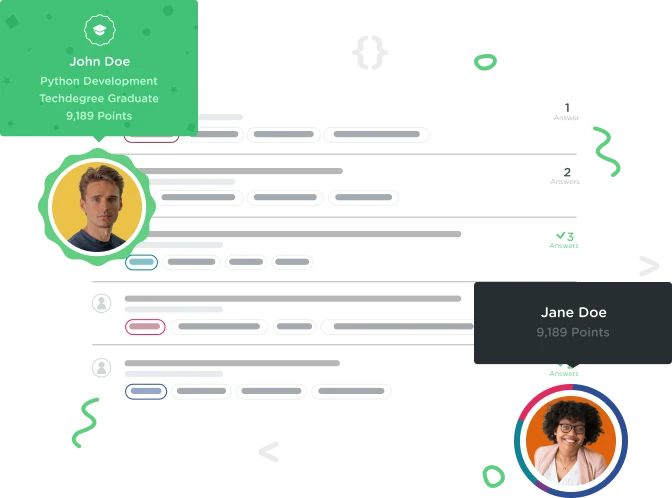
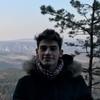
Kareem Jeiroudi
14,984 PointsSharedPreferences are re-initialized in onCreate(). Howcome does the text persist?
I have one practical question about the time mSharedPreferences
is initialized at, which is in the onCreate()
method. How come we retrieve the right text when we call mSharedPreferences.getString(...)
, despite the fact that the variable has been just initialized two lines before that? If you don't know what I mean, take a look at the following Activity class, more specifically, I would just like of anyone to be kind and explain to me how the text persisted in this lifecycle.
The code works as desired, however, I expected it not to 🤔.
import android.content.Context;
import android.content.SharedPreferences;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
private static final String PREFERENCES_FILE = "com.kareemjeiroudi.shared_preferences_app.preferences";
private static final String KEY_EDITTEXT = "KEY_EDITTEXT";
private EditText mEditText;
private SharedPreferences mSharedPreferences;
private SharedPreferences.Editor mEditor;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mEditText = findViewById(R.id.editText);
mSharedPreferences = getSharedPreferences(PREFERENCES_FILE, Context.MODE_PRIVATE);
// retrieve the key-value pairs stored in shared preferences
String editTextString = mSharedPreferences.getString(KEY_EDITTEXT, "");
mEditText.setText(editTextString);
}
@Override
protected void onPause() {
mEditor = mSharedPreferences.edit();
mEditor.putString(KEY_EDITTEXT, mEditText.getText().toString());
mEditor.apply();
super.onPause();
}
}
1 Answer

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsHello Kareem Jeiroudi
The key to SharedPreferences retrieving the value despite being initialized 2 lines before is courtesy of the magic which happens at Onpause() method which I will come to shortly. When you initialize the SharedPreference Android automatically creates an XML file stored under DATA/data/ shared_prefs folder. Changes made by the Edior .apply() ** are stored in this XML file. By the way, **MODE_PRIVATE is the default mode, where the created XML file can only be accessed by the calling Application. PREFERENCE_FILE is the name of the preference file you wish to access. If it does not exist, it will be created.
When *onPause() * method is called (e.g. when the user presses the back button to exit the app) the code inside onPause() is triggered and the Editor.apply() saves changes to the XML File described above.
The next time onCreate() is called, mSharedPreferences.getString(KEY_EDITTEXT, ""); easily reads the data from the existing XML File and returns the String.
Please let us know if this clarifies.
Kareem Jeiroudi
14,984 PointsKareem Jeiroudi
14,984 PointsSo basically, the next time
oncreate()
is called, the preferences that are read are those that are stored inPREFERENCE_FILE
. And despitemSharedPreferences
having just been re-initialized, the preferences inPREFERENCE_FILE
haven't been overwritten yet, which gets done ateditor.apply()
inonPause()
. Right?Sorry if that just another way to say what you wrote above 😅, but that already clarifies a lot. Thank you so much 🙏!
Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsTonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsThanks Kareem Jeiroudi mSharedPreferences inside the onCreate job is only to retrieve and doesn't overwrite anything. The writing happens on the onPause() where we have the Editor.