Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial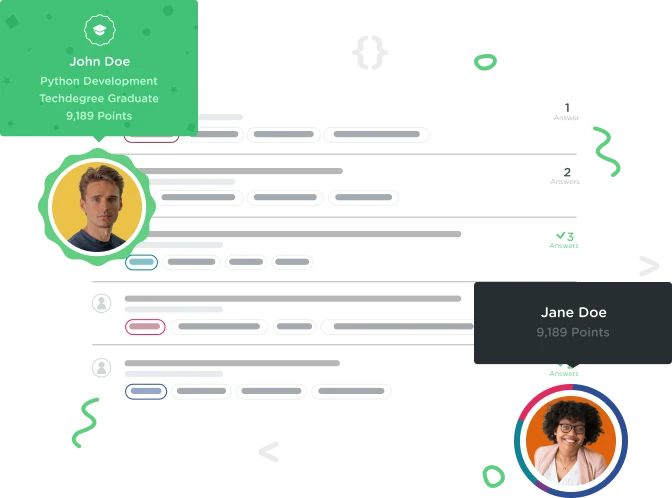
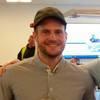
Nicolai Simonsen
4,600 PointsSharing my solution - Any Steelers fans in here?
Just sharing my solution. Any tips/thoughts are greatly appreciated :)
var totalQuestions = 5; // Total number of questions
var correctAnswers = 0; // Number of correctly answered questions
var playerRank = ""; // Player rank
var messageCorrect = "That's the correct answer. Your score is "; // Message for correct answer
var messageIncorrect = "Sorry - the answer is NOT correct. Your score is "; // Message for incorrect answer
// QUIZ Questions::
// 1st Question - "Who wears #84 for the Pittsburgh Steelers?".
var qst1 = prompt("Who wears #84 for the Pittsburgh Steelers?"); // Ask for name of Steelers player
qst1 = qst1.toLowerCase(); // Convert input to lowercase for comparison-simplicity
if (qst1 === "antonio brown" || qst1 === "brown"){ // Check fullname or lastname of Steeler
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
} else if (qst1 === "antonio"){ // If only firstname provided
var qstLastName = prompt("What is the players last name?"); // Ask player for lastname
if (qstLastName === "brown") { // Check lastname
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
} else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
// 2nd Question - "Who wears #81 for the Pittsburgh Steelers?".
var qst2 = prompt("Who wears #81 for the Pittsburgh Steelers?"); // Ask for name of Steelers player
qst2 = qst2.toLowerCase(); // Convert input to lowercase for comparison-simplicity
if (qst2 === "jesse james" || qst2 === "james"){ // Check fullname or lastname of Steeler
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
} else if (qst2 === "jesse"){ // If only firstname provided
var qstLastName = prompt("What is the players last name?"); // Ask player for lastname
if (qstLastName === "james") { // Check lastname
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
} else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
// 3rd Question - "Who wears #7 for the Pittsburgh Steelers?".
var qst3 = prompt("Who wears #7 for the Pittsburgh Steelers?"); // Ask for name of Steelers player
qst3 = qst3.toLowerCase(); // Convert input to lowercase for comparison-simplicity
if (qst3 === "ben roethlisberger" || qst3 === "roethlisberger"){ // Check fullname or lastname of Steeler
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
} else if (qst3 === "ben"){ // If only firstname provided
var qstLastName = prompt("What is the players last name?"); // Ask player for lastname
if (qstLastName === "roethlisberger") { // Check lastname
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
} else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
// 4th Question - "Who wears #92 for the Pittsburgh Steelers?".
var qst4 = prompt("Who wears #92 for the Pittsburgh Steelers?"); // Ask for name of Steelers player
qst4 = qst4.toLowerCase(); // Convert input to lowercase for comparison-simplicity
if (qst4 === "james harrison" || qst4 === "harrison"){ // Check fullname or lastname of Steeler
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
} else if (qst4 === "james"){ // If only firstname provided
var qstLastName = prompt("What is the players last name?"); // Ask player for lastname
if (qstLastName === "harrison") { // Check lastname
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
} else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
// 5th Question - "Who wears #50 for the Pittsburgh Steelers?".
var qst5 = prompt("Who wears #50 for the Pittsburgh Steelers?"); // Ask for name of Steelers player
qst5 = qst5.toLowerCase(); // Convert input to lowercase for comparison-simplicity
if (qst5 === "ryan shazier" || qst5 === "shazier"){ // Check fullname or lastname of Steeler
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
} else if (qst5 === "ryan"){ // If only firstname provided
var qstLastName = prompt("What is the players last name?"); // Ask player for lastname
if (qstLastName === "shazier") { // Check lastname
correctAnswers += 1; // If true, add 1 to correctAnswers
alert(messageCorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
} else {
alert(messageIncorrect + correctAnswers + " of " + totalQuestions); // Display message + score
}
// All questions have been answered. Player will recieve rank based on score.
switch(correctAnswers) { // Determine rank based on correctAnswers
case 1: // If correctAnswers = 1
playerRank = "Waterboy";
break;
case 2: // If correctAnswers = 2
playerRank = "Rookie";
break;
case 3: // If correctAnswers = 3
playerRank = "Pro";
break;
case 4: // If correctAnswers = 4
playerRank = "All-Star";
break;
case 5: // If correctAnswers = 5
playerRank = "Hall of Famer";
break;
default:
playerRank = "Assistant to the Waterboy";
break;
}
alert("You have been assigned the following rank: " + playerRank); // Display rank
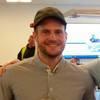
Nicolai Simonsen
4,600 PointsThanks Chris!
1 Answer
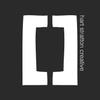
Angelica Hart Lindh
19,465 PointsHi Nicolai,
I liked your solution with the conditional check on the user for the players last name. I thought that was a nice addition to the quiz.
I would recommend that you make use of the built in JavaScript data types such as Arrays and Objects in order to write DRYer code so as to make your code more maintainable, easier to read and shorter.
I have written a refactored version you can find on jsFiddle using some new ES2015 syntax.
Hope it helps to visualize how you can make use of the data types.
Go the Steelers!! (in whatever sport they play)
Cheers
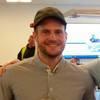
Nicolai Simonsen
4,600 PointsWow, thank you so much for taking the time to help me out! This is priceless - I'll definitely be looking into ES2015 and more 'advanced' JavaScript.
I was actually thinking in terms of an array, but went easy and perhaps lazy with my solution. Time to do some studying and then do it right in another project! Highly motivated right now..
It almost seems wrong not buying you a beer for this.
Again, thanks a lot.
/Nicolai
Chris Davis
16,280 PointsChris Davis
16,280 PointsNice!