Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial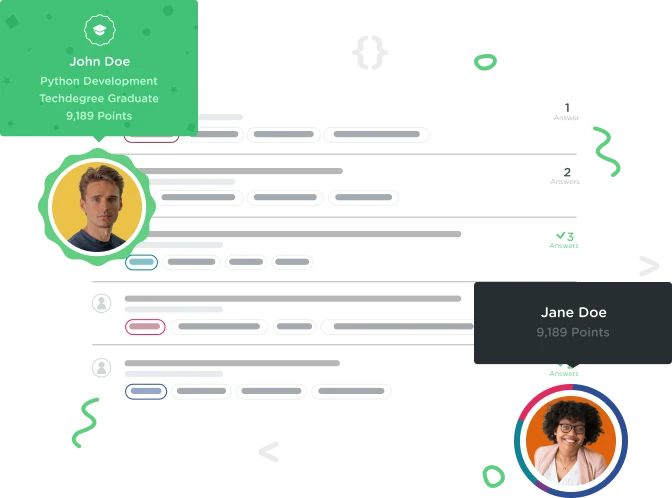
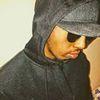
ibrahim Warsame
7,803 PointsShopping list program.
Hi! This is my shopping list program, before watching the video.
shoopping_list = []
def general_info():
print("Add an one item to your list at a time.")
print("Type DONE in all upper case letters to exit")
print("Type SHOW in all upper case letters to see your current list.")
def user_input(inpUt):
if inpUt.isalpha():
shoopping_list.append(inpUt)
else:
print("Invalid")
loop = True
while loop:
general_info()
inpUt = input()
if inpUt == "DONE":
break
elif inpUt == "SHOW":
print(shoopping_list)
user_input(inpUt)
print(shoopping_list)
What do you guys think about the program. I will thank you for any kind of feedback :)
1 Answer
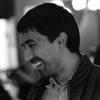
Martin Cornejo Saavedra
18,132 PointsNever modify a variable from the global scope inside a function, that's not a good practice, makes hard to check errors later. Group functionalities to reuse code later.
def general_info():
print("Add an one item to your list at a time.")
print("Type DONE in all upper case letters to exit")
print("Type SHOW in all upper case letters to see your current list.")
def user_input(inpUt):
if inpUt.isalpha():
return True
return False
def show(shoopping_list):
print("----------------------------------")
for item in shoopping_list:
print(item)
print("----------------------------------")
def createList():
shoopping_list = []
while True:
general_info()
inpUt = input()
if inpUt.lower() == "DONE".lower():
break
elif inpUt.lower() == "SHOW".lower():
show(shoopping_list)
continue
elif not user_input(inpUt):
print("Invalid")
continue
shoopping_list.append(inpUt)
return shoopping_list
def main():
shoopping_list = createList()
show(shoopping_list)
main()
ibrahim Warsame
7,803 Pointsibrahim Warsame
7,803 PointsThanks for the feedback! i will try to do what you just said.