Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial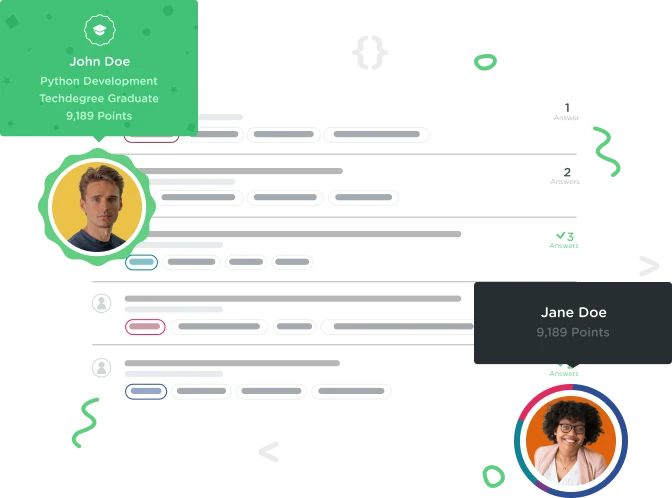

Rakshit H Ravishankar
1,309 PointsShopping list program error:
When the list is not empty and we try to add an element in position 0, the element gets added in the second position.This gives undesirable results This is because, we use .insert(position-1,item). There must be a way to check and make sure that we are not entering '0' for the position.
4 Answers
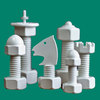
Steven Parker
229,783 Points
There are several ways to deal with this situation.
First, you must decide what kind of behavior you want from the program when 0 is given. Do you want it to add to the top? Or add to the end? Or print an error and require a new input? The way you handle it would vary with the desired outcome.
For an example, if you want it to perform the same as when 1 is given, a simple way to handle it would be to modify line 30 like this:
position = max(abs(int(position)), 1)
This would make 1
the lowest possible value that position could have.

Ullas Savkoor
6,028 PointsI agree with the statement made by mwagner that the item would be added to the end. The possible explanation for addition to 2nd position is you are doing an abs on position - 1 which would result in item being added to index 1. There are 2 possible solutions : Either you expect the user to add the item from index 1 or use a condition which checks if position is in range (1, len(shopping_list)) before adding the item failing which you append the item to the end of list.

Mike Wagner
23,559 Points@Ullas - I'm not actually using abs in that way, so I'm not sure why I'm experiencing the weird behavior. Essentially, what I'm doing is:
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(item)
The earlier code checks if the list is populated and, if it doesn't, sets position = 0, otherwise it asks for which position in the list it should be inserted. It works perfectly fine, unless I actually manually enter 0 as the value, in which case it inserts to position = -2 for some reason. It doesn't matter how long the list is, and I've tried virtually everything I can think of, but somehow I can't come to terms with how 0 - 1 = -2 in this context.

Ullas Savkoor
6,028 Points@mwagner - I would like to replicate the weird behaviour , could you paste your whole code? It would help me to replicate and understand its root cause.

Mike Wagner
23,559 Points@Ullas - I've dropped my first experience with this issue here at repl.it to save space. I can paste the actual code, if you'd prefer, though. Best I can tell, I didn't butcher the code enough to cause this, but I'm still an amateur with Python.

Ullas Savkoor
6,028 Pointsmwagner : Thanks for sharing your code. I was able to replicate it. It is not a weird behavior, basically it is how insert works let me give you an example:
list1 = [1,2,3,4]
to this list1 if I insert 'a' at index 0 the rest of the contents will shift to the right, so
list1.insert(0,'a') is ['a',1,2,3,4]
now if I add 'b' at the last index of list1 ie 4 ,'b' wouldn't be the last item instead 4 will be shifted to the right and 'b' is inserted at index 4
list1.insert(4,'b') would give me ['a',1,2,3,'b',4]
so essentially when a negative index of -1 is given the item at the last position is shifted to the right and at the vacant position the new item would be inserted
ie list1.append(-1,'c') would give ['a',1,2,3,'b','c',4]
Since this is the behavior of insert , you could instead use append() when index of 0 is given.
Hope this clears things up for you ,if not feel free to reply.

Mike Wagner
23,559 PointsUllas Savkoor - Ah, yeah it makes more sense now. I had erroneously assumed that since there wasn't anything to the right of shopping-list[-1] that it that it would have nothing to shift, but I had forgotten it takes the item found at that index and pushes right. It won't be hard to rectify that with a simple check. Thanks for clearing that up.
Mike Wagner
23,559 PointsMike Wagner
23,559 PointsIf entering 0 with the code in the lesson, shouldn't it just reconcile as index -1 (i.e. 0 - 1 = -1) and therefore put it at the end of the list? I'm puzzled by why it seems to drop in index -2 (at least for me) instead, but even more by a need to define extra functionality for something so trivial. Can you provide insight?