Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial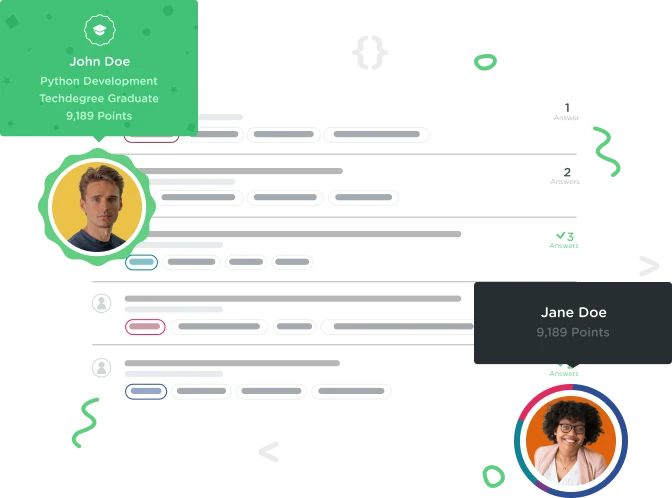

Tony Brackins
28,766 PointsShopping List Project
I haven't been able to get any of these syntax or programs running with Kenneth.
Apparently what he's not going over is how many spaces is supposed to be between each line, because I keep getting errors that I don't have the right amount of indentation or spaces:
shopping_list = list()
print("What should we pick up at the store?");
print("Enter 'Done' to stop adding items.");
while True:
new_item = input("> ")
if new_item == 'DONE':
break
shipping_list.append(new_item)
print("Added! List has {} items.".format(len(shopping_list)))
continue
print("Here's your list:");
for item in shopping_list:
print(item)
2 Answers

Joseph Kato
35,340 PointsHere are a few of my observations:
In Python semicolons are only used to delimit compound statements (so they're not needed after your
print
statements).Your while-loop needs a little reformatting; for instance, here's how I'd write it:
while True:
new_item = input("> ")
if new_item.upper() == 'DONE':
break
shopping_list.append(new_item)
print("Added! List has {} items.".format(len(shopping_list)))
This will break
out of the loop if the user enters "Done" (case insensitive due to the .upper
call). If, however, the user enters anything else, the rest of the loop body will still execute. In contrast, the way you currently have it, the loop will only prompt the user to enter a new item, or it will break out of the loop (it won't actually add the item since the body is aligned with the break
statement).
Also, the continue
statement is unnecessary, since the loop will either break out of its execution or it will add the item. So, there's nothing for the continue
statement to skip over.
So, putting it all together, we have:
shopping_list = list()
print("What should we pick up at the store?")
print("Enter 'Done' to stop adding items.")
while True:
new_item = input("> ")
if new_item.upper() == 'DONE':
break
shopping_list.append(new_item)
print("Added! List has {} items.".format(len(shopping_list)))
print("Here's your list:")
for item in shopping_list:
print(item)

Geoff Parsons
11,679 PointsIt's hard to tell with whitespace but it looks like you may be alternating between spaces and tabs.