Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial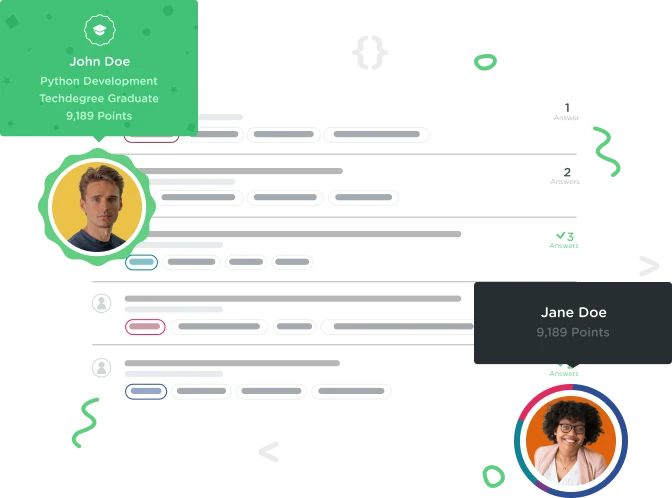
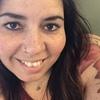
Nichole "Nikki" Carnesi
7,103 PointsShopping list take 3- lines separating my list...
In the shopping list take 3, I was testing out my code and the list worked but as I watched Kenneth's list grow I realized ours looked differently. My list has lines separating each item:
Here's your list:
- milk
__________
- bananas
__________
- oranges
__________
Where Kenneth's has no lines. I am not sure how this is happening, as I am sure my code is just like his. Help me understand!
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear_screen()
print("What should we pick up at the store?")
print("""
Enter 'DONE'' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should I add {}?\n"
"Press ENTER to add to the end of the list\n"
"> ".format(item))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear_screen()
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("_"*10)
show_help()
while True:
new_item = input("> ")
if new_item.upper() == 'DONE' or new_item.upper() == 'QUIT':
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'SHOW':
show_list()
else:
add_to_list(new_item)
show_list()
1 Answer

Umesh Ravji
42,386 PointsHi Nichole, the problem is that you have the print
function indented once too much, placing it inside the for
loop so it runs on each iteration of the loop, instead of just running once at the end. To fix this, just un-indent by one level to make sure it is no longer inside the for
loop.
def show_list():
clear_screen()
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("_"*10)
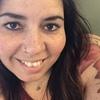
Nichole "Nikki" Carnesi
7,103 PointsThank you Umesh, I fixed it and it works now! :)
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsChristopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsPlease put a link to the video you were watching.