Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial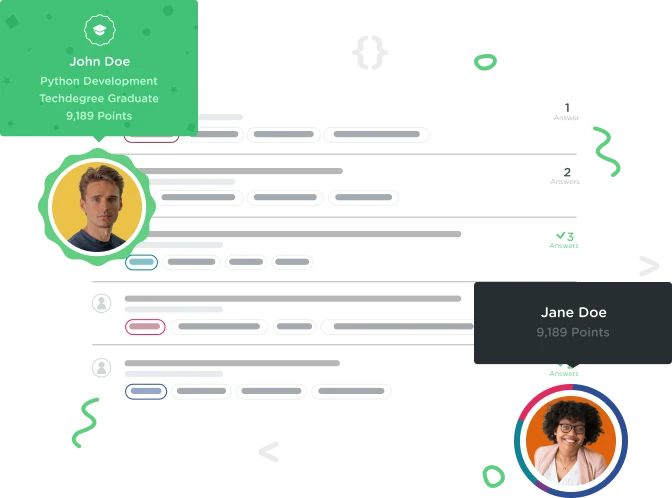
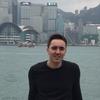
Sam Frampton
3,796 PointsShopping List Take 3 - Need help debugging
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear_screen()
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to show the list.
""")
def add_to_list(items):
show_list()
if len(shopping_list):
position = input("Where should I add {}?\n"
"Press ENTER to add to the end of the list\n"
"> ".format(items))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, items)
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear_screen()
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, items))
index += 1
print("-"*10)
show_help()
while True:
new_item = input(">")
if new_item.upper() == 'DONE' or new_item.upper() == 'QUIT':
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'SHOW':
show_list()
continue
else:
add_to_list(new_item)
show_list()
#Error In terminal#
File "list_app.py", line 66, in <module>
add_to_list(new_item)
File "list_app.py", line 37, in add_to_list
show_list()
File "list_app.py", line 48, in show_list
print("{}. {}".format(index, items))"
^^ This is what I get in the terminal when I enter an item on the list. Can someone review my code and help me get back on track pls?
1 Answer
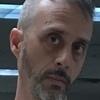
frankgenova
Python Web Development Techdegree Student 15,616 Pointsfirst hint:
for item in shopping_list:
print("{}. {}".format(index, items)) # probably should be item not items
- as you are iterating through shopping list you are storing it as 'item' (singular), on the next line, you are referencing it as 'items' (plural)
- I use pylint and I find it helpful for detecting these kinds of errors
Sam Frampton
3,796 PointsSam Frampton
3,796 PointsThanks, I'll check out Pylint now.