Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial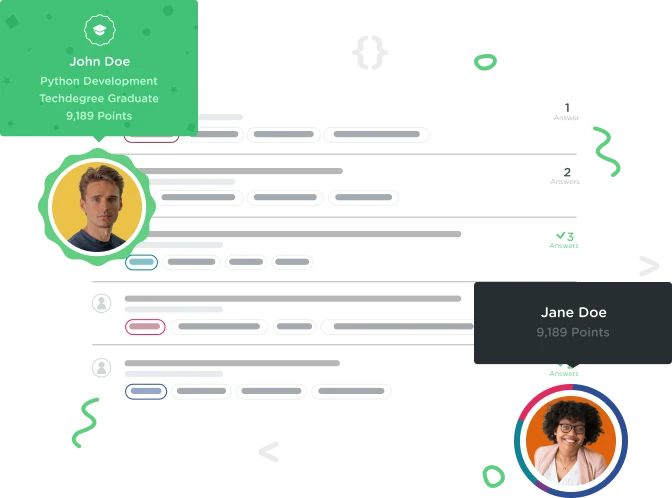
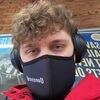
Szymon Dabrowski
2,207 Pointsshopping_list.py Different approach
I just wanted to share what I did differently to Craig in my shopping list application and why I have done so...
To start off I have added an additional command after I have showed the app to someone else. The new command is called "Remove" and it removes the most recent item. The reason for that is sometimes a user can make a mistake and this allows the user to correct that by deleting the most recent item and then trying again. If the list is empty to handle the error I have used an if statement instead of a try block. so if the list is empty it says "The list is empty!". To remove an item I used the .pop()
method so it removes the last item from the list and then I can use the variable "removed_item" to then confirm the correct item was removed.
What I have also noticed when someone else was using the app is that even though the help section shows that you must enter a command in CAPITALS the user still used lower case or only capitalized the first letter which would result in a nasty error. I thought the best approach for this instead of using a "try" block and handling the error and, telling off the user for not using caps. I instead used the .upper()
method to force the string to be converted into upper case. So no matter how the command is typed lower or upper case it will work.
I did not like how Craig has done the show command. Instead of using a for loop to print each item I have used a .join()
method to convert the iterable list into string and then add a ", " between each item. We learned this in a previous lesson. I think this way it is more pretty and easier to read.
I have also made it so when the list is shown or printed with the done command the first letter of each work is capitalized to make it look nicer.
I have fixed the common mistake when adding the first item with an if statement. So when the first item is added instead of "there are 1 items in the list" it says "there is 1 item in the list".
Instead of using continue after each command I have used an else
for adding an item to the list as I prefer it that way.
I have also made copies of the list at different stages. But I am unsure if I needed to do that at all. Perhaps not. But it does not break the code after testing it.
I have also used this project as an opportunity to train to use git and github which I 100% recommend you do too!
I hope you may find my approach useful and, I would love to see how you guys done your shopping list and if you have done anything differently or added something extra to your code
shopping_list = []
def show_help():
print("What should we pick up at the store?")
print("""
To add an item. Enter what you need after the '==>' sign.
Enter "HELP" to show help.
Enter "SHOW" to see your current list.
Enter "REMOVE" to delete the most recent item.
Enter "DONE" to stop adding items.
""")
def add_to_list(item):
shopping_list.append(item)
num_of_items = len(shopping_list)
if num_of_items == 1:
print("The item: '{}' has been added! There is currently {} item in the list."
.format(item, num_of_items))
else:
print("The item: '{}' has been added! There are currently {} items in the list."
.format(item, num_of_items))
def show_list():
current_list = shopping_list.copy()
current_shopping_list = ", ".join(current_list)
current_shopping_list = current_shopping_list.title()
print("Here's your list: {}".format(current_shopping_list))
def remove_item():
if shopping_list == []:
print("The list is empty!")
else:
removed_item = shopping_list.pop()
print("Item: {} has been removed!".format(removed_item))
show_help()
while True:
new_item = str(input("==> "))
if new_item.upper() == "DONE":
break
elif new_item.upper() == "HELP":
show_help()
elif new_item.upper() == "SHOW":
show_list()
elif new_item.upper() == "REMOVE":
remove_item()
else:
add_to_list(new_item)
final_list = shopping_list.copy()
final_shopping_list = ", ".join(final_list)
final_shopping_list = final_shopping_list.title()
print("Here's your completed list:",final_shopping_list)