Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial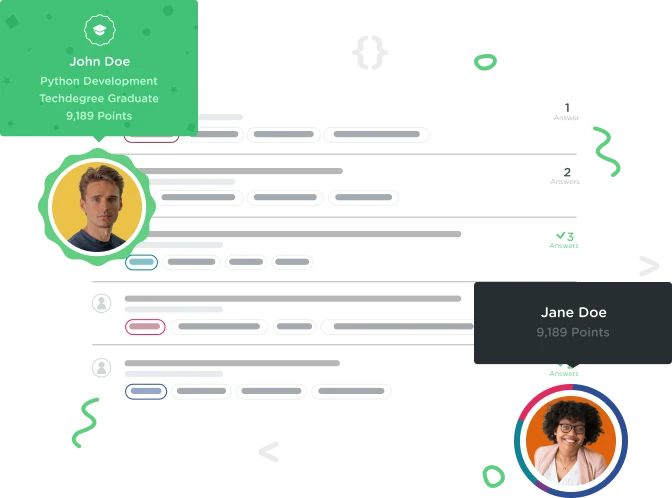
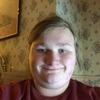
Richard Duffy
16,488 PointsShorter code
Hey,
This is my answer to the challenge:
var html = '';
var rgbColor;
function randomColour() {
return Math.floor(Math.random() * 256 );
}
for( var i = 0; i < 10; i += 1) {
rgbColor = 'rgb('+ randomColour() + ',' + randomColour() + ',' + randomColour() + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
Regards,
Richard.
2 Answers
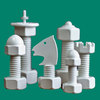
Steven Parker
229,744 PointsIf something is only going to be used once, it might make sense to do it the shortest way.
I think one of the points of the lesson is re usability, which refactoring into more functions can help to achieve. This might actually take more code at first, but as the program grows it will save space (and improve readability) in the long run.

julianweisser
12,123 PointsI did the following because I think it's reusable. Is it the shortest way? No, but it removes the color duplication from the loop and turns it into a function as requested.
var html = '';
var red;
var green;
var blue;
var rgbColor;
function color( red, green, blue ) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
}
for ( i = 0; i < 10; i += 1 ) {
color();
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);