Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial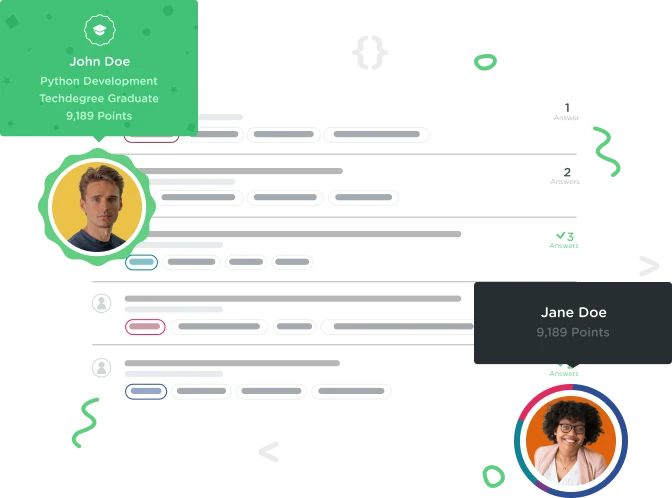

Angelic Thomason
1,889 PointsShould I use a while instead?
Would a for loop or a while loop be better?
var temperatures = [100,99,90,80,70,65,30,10];
for (var i = 0; i < temperatures.lengh; i = 1); {
console.log(temperatures[i]);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Owen McComas
8,472 PointsYou can use either and they will both work perfectly well. That being said, people tend to use for loops quite a bit more often than while loops or do-while loops in the real world. Looking at your for loop, there are a few minor things that will make it so it doesn't work.
- It looks like you just misspelled length in 'temperature.length'
- There shouldn't be a semi-colon at the end of the parenthesis there in the first part of the for-loop.
- You need to specify the iteration declaration. "i = 1" should be i++ or i += 1. This tells the loop to add 1 to the index value each loop.
Your for loop should look something like this.
var temperatures = [100,99,90,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i++ ) {
console.log(temperatures[i]);
}

Angelic Thomason
1,889 PointsOk I see now, it's starting to come a little easier now
Devin Thomas
2,935 PointsDevin Thomas
2,935 PointsYou did everything correct. You want your last statement to increment through each iteration and remove the semi-colon before the open bracket.