Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial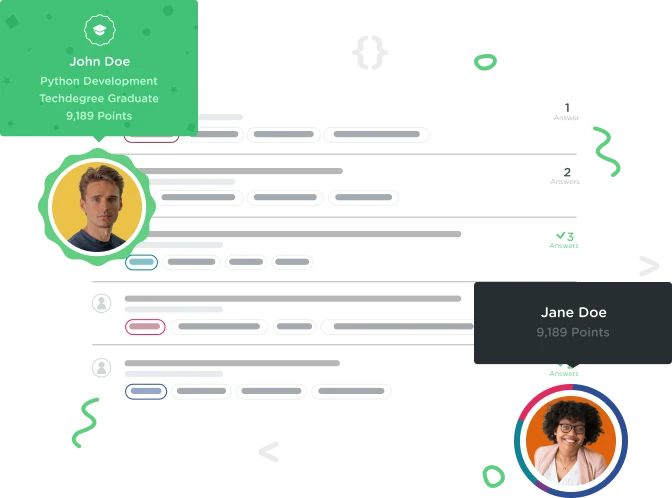

Matheus Baumgart
977 PointsShouldn't the second method be the data instead a callback function?
According to the video, the second method is the data and not the callback as the question suggests, isn't it? The quiz is asking to add a callback as the second method.
$.get("footer.html");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
</head>
<body>
<div id="main">
<h1>AJAX with jQuery</h1>
</div>
<div id="footer"></div>
<script src="jquery.js"></script>
<script src="app.js"></script>
</body>
</html>
2 Answers

jason phillips
18,131 PointsSo with $.get() the only input parameter that is required is the url, which in this example above is the html page. Data in the video example refers to passing a query string variable along with the call to the page you are calling. For example if you wanted to pass a query string variable, you would do it by passing it in second input variable. the callback can return data also. So think about it like this, you tell jquery what page you want to get from, you can also tell jquery to pass along some data, and lastly you tell jquery what to do with the response from the page. What you will often see with this call with named variables like this:
$.get({
url:'footer.html',
data: {
var: 'variableValue'
},
function(data){
//do something with the data
}
});
You will also see people dropping the callback(3 param) in favor of chaining a done callback on the end of the function:
$.get({
url:'footer.html',
data: {
var: 'variableValue'
}
}).done(function(data){
//do something with the data
});
I hope all of that made sense. Check out the reference docs for more information:
https://api.jquery.com/jquery.get/
if you have questions you can contact me at jphillips05@gmail.com
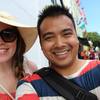
Cernan Bernardo
17,437 PointsIf you take a look at the documentation for jQuery's get() (https://api.jquery.com/jquery.get/), you will see that the function can take 4 different parameters. 3 of them (data, success, and datatype) are optional. The URL is the only required parameter. So if the question is asking you to add a callback function as the second parameter, then it should look like $.get("footer.html", function(data){}); I hope this helps.

Matheus Baumgart
977 PointsI see, thanks for clarifying. My question were also related to the video, because he taught the second should be data, and the third should be callback, and a previous quiz asked the same question with the right answer being "the second argument is the data". I thought it was a rule, my bad. However it could be changed to not confuse other users.
Thanks for the answer anyway.
Matheus Baumgart
977 PointsMatheus Baumgart
977 PointsI see, thanks for clarifying, Jason. My question were also related to the video, because he taught the second should be data, and the third should be callback, and a previous quiz asked the same question with the right answer being "the second argument is the data". I thought it was a rule, my bad. However it could be changed to not confuse other users.
Thanks for the answer anyway.