Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial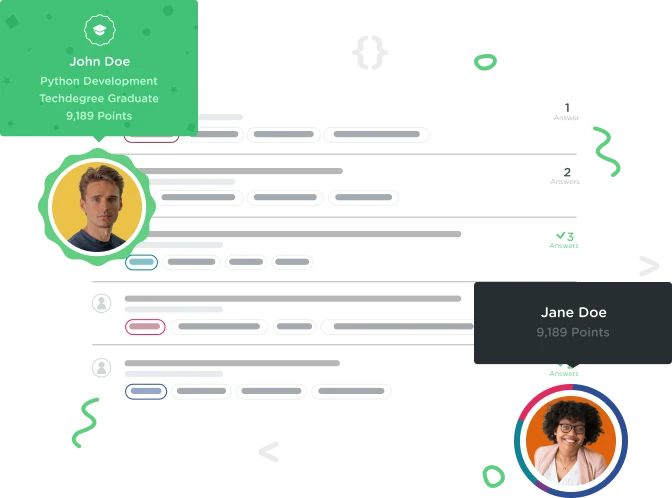

John Skelton
735 PointsShouldn't this work?
def loopy(items): for item in items: if items[0] == 'a': continue
print(items[1:6:1])
loopy("abcdef")
def loopy(items):
for item in items:
if items[0] == 'a':
continue
# else:
print(items[1:6:1])
## print(items)
loopy("abcdef")
3 Answers
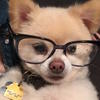
Brian Boring
Courses Plus Student 3,904 PointsSo the question/challenge is: "Loop through each item in items again. If the character at index 0 of the current item is the letter "a", continue to the next one. Otherwise, print out the current member. Example: ["abc", "xyz"] will just print "xyz"."
Currently, the loop in your code doesn't have any effect on the output. All your code is doing right now is printing out characters 1-5 of the argument passed to it (in this case "abcdef"). But the challenge states that if you send it a list (["abc", "xyz"]), then it should loop through the list and only return those items that do not start with "a". So, what needs to be done here is:
create a new list that is empty
new_items = []
run your for loop, but you should add an ELSE. The ELSE needs to add anything that doesn't start with "a" into the new list.
else: new_items.append(item)
Then just return/print your new list
print (new_items)
And you should use Loopy(["abc", "xyz"]) so you know it is producing the correct result.
Hope that helps!

John Skelton
735 PointsThis worked perfectly: Thank you! def loopy(items): new_items = [] for item in items: if "a" in item: continue else: new_items.append(item) print(new_items)
loopy(["abc","xyz"])

John Skelton
735 PointsI got it to work using Brian's suggestion.