Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial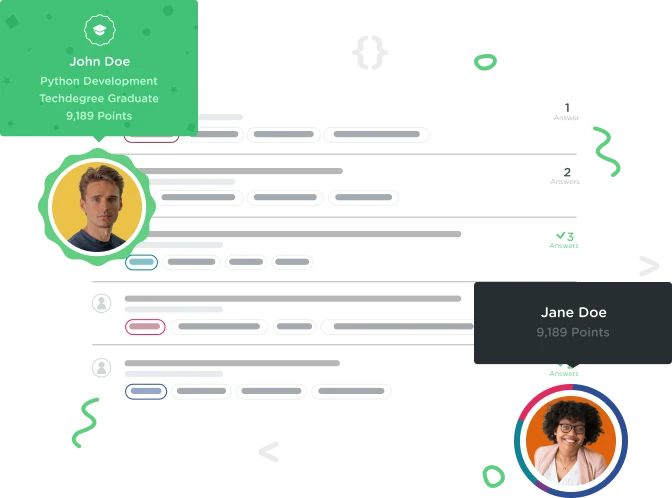

Charles Hedricks
3,149 PointsShow next fact button fails to work after random code updated.
After following the online tutorial for adding random text on button click, the emulator fails to update the messages properly. It appears the Show next fact button does not update the message on click. Only shows static text as defined by label in xml tab.
This is the java code:
public class JustFunFacts extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_just_fun_facts);
// Declare our view variables and assign the views from the layout file
final TextView factLabel = (TextView)findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random(); // Construct a new random generator
int randomNumber = randomGenerator.nextInt(3);
if (randomNumber == 0) {
fact = "Ants stretch when they wake up in the morning.";
}
else if (randomNumber == 1) {
fact = "Ostriches can run faster than horses.";
}
else if (randomNumber == 2) {
fact = "Olympic gold medals are actually made mostly of silver.";
}
else {
fact = "Sorry there was an error!";
}
}
};
showFactButton.setOnClickListener(listener);
}
I have reviewed the videos going back to where we started to update for "random code" and can not see where there is an mistake in the code. The only thing I can see as a problem would be keeping the fun fact tied to the xml string rather than pulling in the new fact string generated by the method.
8 Answers
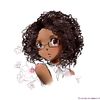
Gloria Dwomoh
13,116 PointsI'm not sure if you added this and it just doesn't appear here but I think you need a brace in the end. I'd add it down below and comment it.
public class JustFunFacts extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_just_fun_facts);
// Declare our view variables and assign the views from the layout file
final TextView factLabel = (TextView)findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random(); // Construct a new random generator
int randomNumber = randomGenerator.nextInt(3);
if (randomNumber == 0) {
fact = "Ants stretch when they wake up in the morning.";
}
else if (randomNumber == 1) {
fact = "Ostriches can run faster than horses.";
}
else if (randomNumber == 2) {
fact = "Olympic gold medals are actually made mostly of silver.";
}
else {
fact = "Sorry there was an error!";
}
}
};
showFactButton.setOnClickListener(listener);
}
}// <-Added brace

Charles Hedricks
3,149 PointsThanks for the suggestion... I cut / pasted the code for the onCreate section, the brace under showFactButton does close out the code according to Android Studio. There are two more sections of code below it that are closed out afterwards, completing the entire app.
Right now - with the code as listed, the button will not acknowledge input, and the text displayed as a placeholder of sorts for the 'fun fact' does not change. Right now the fun fact message is displayed from the strings.xml file, does not change to array information when run in the emulator. No errors are displayed in the log, nor the compiler messages.
Am wondering if the strings.xml or the "onClick" location in the xml properties editor for the button needs to be adjusted. Did not see reference to this in the video so hesitant to proceed.
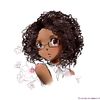
Gloria Dwomoh
13,116 PointsI think you should check your onClick method in your main java file. The code should work as it does for the instructor, if that doesn't happen it means there is a bug.

Charles Hedricks
3,149 PointsI was able to re-write the code, from scratch in a new project file, and it worked as it should. I compared both line by line and found no differences, just that the old project still presented issues.. I deleted the old project file and have moved forward with the new.
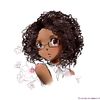
Gloria Dwomoh
13,116 PointsThat's good and strange at the same time. I'm glad it works now.

Brian McIver
1,156 PointsI'm having the same issue as above. Code looks fine, but nothing happens when clicking the button. No errors in logcat or any indication something is wrong. I will rebuild the project, but curious as to what the issue might be. Thanks!
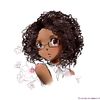
Gloria Dwomoh
13,116 PointsBrian McIver Try to clean gradle. Maybe that helps.

Brian McIver
1,156 PointsSame problem.

Brian McIver
1,156 PointsI am getting these if that makes a difference.
11-14 20:31:29.844 1317-1317/com.mcivermedia.funfacts E/OpenGLRenderer﹕ Getting MAX_TEXTURE_SIZE from GradienCache 11-14 20:31:29.852 1317-1317/com.mcivermedia.funfacts E/OpenGLRenderer﹕ MAX_TEXTURE_SIZE: 16384 11-14 20:31:29.860 1317-1317/com.mcivermedia.funfacts E/OpenGLRenderer﹕ Getting MAX_TEXTURE_SIZE from Caches::initConstraints() 11-14 20:31:29.860 1317-1317/com.mcivermedia.funfacts E/OpenGLRenderer﹕ MAX_TEXTURE_SIZE: 16384
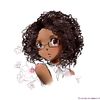
Gloria Dwomoh
13,116 PointsThat is not much of an "error" it is more of informational. I am not sure if you are following the exact same tutorial or you are adding your own changes to it. If you are doing this tutorial it suppose to work if you have no errors, but if you are changing it a bit then that can affect it from running properly if the code is having some hard to see error.

Brian McIver
1,156 PointsI have not changed it in any way.
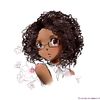
Gloria Dwomoh
13,116 PointsSadly something might be wrong with the code. Like the previous asker, once he rewrote the code everything went right. It is hard to debug it without an error message. To make sure it is not android studio try downloading the instructors code and run it. If it works then it is probably not android studio but if it doesn't then there might be a problem there. Maybe in the settings of the emulator or something else.

Brian McIver
1,156 Pointspackage com.mcivermedia.funfacts;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
//Declare our view variables and assign the Views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
// The button was clicked, so update the fact label with a new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(3);
// Mooshing = adding '+ ""' - String
// Update the label with our dynamic fact
if (randomNumber == 0) {
fact = "Ants stretch when the wake up.";
} else if (randomNumber == 1) {
fact = "Ostriches can run faster than horses";
} else if (randomNumber == 2) {
fact = "Olympic gold medals are actually made mostly of silver";
} else {
fact = "Sorry, there was an error";
}
}
};
showFactButton.setOnClickListener(listener);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_fun_facts, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
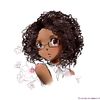
Gloria Dwomoh
13,116 PointsI don't really see an error but it is hard to tell. Try running the instructors code as I said and if it works then that means it is not from Android Studio.

Charles Hedricks
3,149 PointsI think you have an extra semi-colon showing just above the "showFactButton.setOnClickLIstener(listener);" which may be causing issues. You may want to try and remove that from the code then save and re-run.
With my issues, at the start of this thread, I could not find a single out of place line of code, nor any missing elements that would be causing my problems to appear. I ended up deleting my project and rebuilding it from scratch to overcome the issues.

Brian McIver
1,156 PointsI just rebuilt it from the instructor code and moved on from there. I'm almost done and it works great. I'm so stoked about Treehouse and Code Oregon!