Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial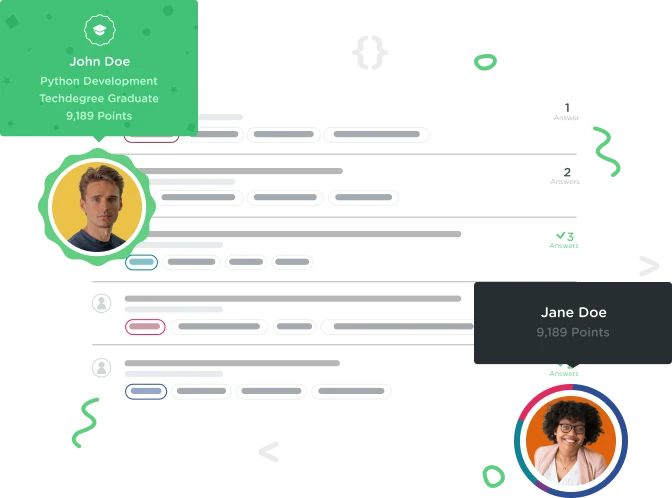
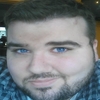
Marcus Parsons
15,719 Points.show() rendering differently in Firefox
Edit: I made a workaround for this that works in Firefox, Chrome, IE. You can check it out in the comments below. And for reference if anyone is using Firefox and notices this behavior, this is due to a bug in Firefox where Firefox gets a 0 value from getComputedStyle
when automatic margins are set. getComputedStyle
is a part of the show() function in jQuery.
Has anyone else noticed that .show() in jQuery renders differently in Firefox than in Chrome? In Chrome, the paragraph with a span that says "It's a trap!" that transitions to be over the Ackbar picture transitions as you would expect, with the span element coming into focus where it is set in the CSS with the margins applied of "100px auto 25px".
I noticed that in Firefox, however, it transitions to where the top and bottom margins are set but the left and right margins are 0 and then after a delay, it disappears and reappears at the correct automatically assigned left and right margins. In other words, it looks nothing like the actual transition.
Anyone have any idea why this happens? Here is the full code from the lesson:
<!-- INDEX.HTML -->
<!DOCTYPE html>
<html>
<head>
<title>Take Evasive Action</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<p class="warning">
<span>It's A Trap!</span>
</p>
<div class="image">
<img src="img/ackbar.gif" />
</div>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
/* style.css */
body {
background: #2d3339
}
.warning {
border: 5px solid #e1dfbe;
width: 200px;
margin: 100px auto 20px;
border-radius: 5px;
padding: 20px 10px;
text-align: center;
font-size: 32px
}
.warning span {
color: #f4f3ce;
font-family: sans-serif;
font-weight: bold;
}
.image {
position: absolute;
top: 20%;
width: 100%;
text-align: center;
z-index: -1
}
//app.js
$(".warning").hide().show("slow");
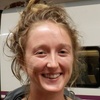
edeharrison
Full Stack JavaScript Techdegree Student 11,127 PointsThanks for the comment on this Marcus. I had the same issue with Firefox while following this video. Finding your post gave me an explanation very quickly.
Cheers
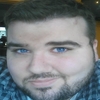
Marcus Parsons
15,719 PointsNo problem, Ede. It is unfortunate that Firefox has yet to fix their getComputedStyle() bug despite several bug requests to fix it. I couldn't stand to watch the animation jump from the far left of the screen, to the top left and then to where it should be, so I had to fix it haha Cheers! :)
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsI conceived a workaround for use with Firefox (and works with Chrome, IE tested). This utilizes a formula that I conceived to get what the margin values are for a centered image (or rather more specifically those with left and right margins set to auto).
If you manually set the margins in the CSS without using auto, this workaround would not be required, but because of the way Firefox uses
getComputedStyle
in the .show() method of jQuery, it is required when using auto margins.