Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial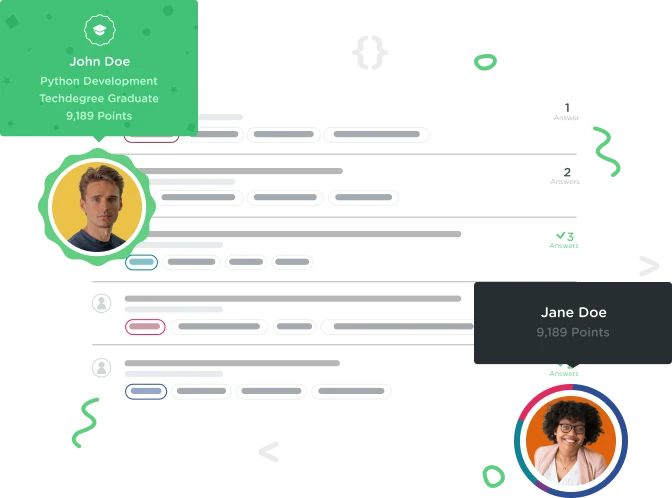
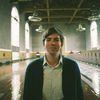
Michael Chambers
Full Stack JavaScript Techdegree Graduate 28,393 PointsShuffle Function Explanation
Can someone provide a detailed explanation of how the variable assignments in the last few lines of this shuffle function work? I've copied the code twice, once with comments so you can see what I (think) I understand and once without for readability.
function shuffle(arr) {
var j, x, i;
for (i = arr.length - 1; i > 0; i--) { // the counter is se to equal the length of the deck -1
//and run until the counter reaches 0. -1 accounts for the 0 index.
j = Math.floor(Math.random() * (i + 1)); // j is equal to a random number between 1 and the // length of the passed in array which should be 52 since the array hold a deck of cards.
x = arr[i]; // x is set to equal the card found at the counter index number
arr[i] = arr[j]; //Unless the comments above are wrong, I think this is where I get lost.
arr[j] = x;
}
return arr;
}
function shuffle(arr) {
var j, x, i;
for (i = arr.length - 1; i > 0; i--) {
j = Math.floor(Math.random() * (i + 1)); //generates a random number between 1 and the number of cards in deck or length of array in other words
x = arr[i];
arr[i] = arr[j];
arr[j] = x;
}
return arr;
}
Cheers and Thanks in Advance!
Michael
1 Answer
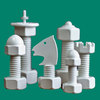
Steven Parker
230,274 PointsIt looks like you may have already figured out your issue, but just in case, here's your original code with corrected/added comments:
j = Math.floor(Math.random() * (i + 1)); // j is equal to a random number between 0 and the current index
x = arr[i]; // x is set to equal the card found at the counter index number
arr[i] = arr[j]; // the card at the current index is replaced by the randomly selected one
arr[j] = x; // the random card is replaced by the one previously at the index
If you want to eliminate the need for the "x" variable, you can replace the last 3 lines with just one using destructuring assignment. Plus, I added a test to skip the swap if the same index was chosen:
if (i != j) // do nothing if the indexes are the same
[arr[j], arr[i]] = [arr[i], arr[j]]; // otherwise swap the cards at indexes i and j
Michael Chambers
Full Stack JavaScript Techdegree Graduate 28,393 PointsMichael Chambers
Full Stack JavaScript Techdegree Graduate 28,393 PointsI found a fantastic video, which gives a detailed breakdown of this function. It's based on the "Fisher-Yates" algorithm. The loop moves backward through the deck and swaps the place of each card with another card in the deck. As the counter moves down, the range of random numbers between 1 and i is also getting smaller. I'm going to try to break down the code I had trouble with in my original comment.
Here's that video! https://www.youtube.com/watch?v=tLxBwSL3lPQ