Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial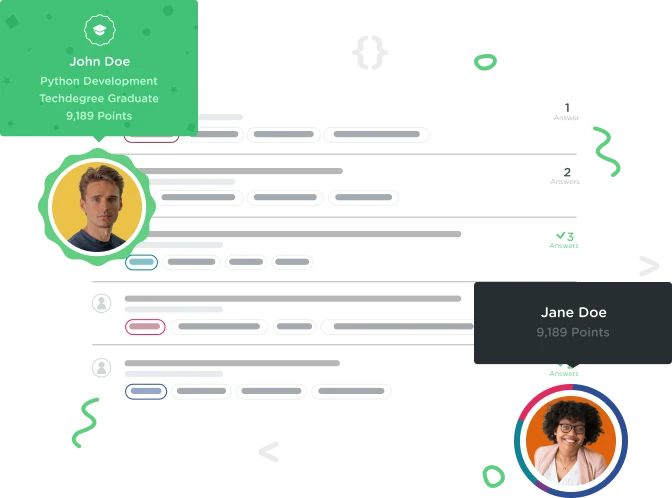
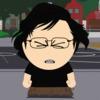
George Bucio
2,743 PointsSibling Traversal Challenge (assigning className to parentNode)
Hello,
I'm not quite sure what kind of answer this challenge is looking for. It's likely I've made an error somewhere, but I just cannot seem to find what I've done incorrectly.
Thanks!
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(event) {
if (event.target.tagName == 'BUTTON') {
let parentLine = event.target.parentNode;
parentLine.className = "highlight";
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
3 Answers
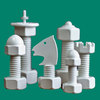
Steven Parker
231,269 PointsThe instructions say "a class of highlight
should be added to the paragraph element immediately preceding that button".
So it's not the button's parent element that should get the class, that would the list item ("li"). It should go on the paragraph right before the button.
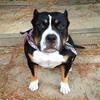
Mark Warren
19,252 PointsThis worked for me:
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
e.target.previousElementSibling.className = "highlight";
}
});
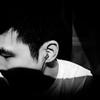
Luke Lai
5,842 PointsMay I ask why it would not work if I assign e.target.previousElementSibling to a new variable, then I change its class name ?
I tried on my own html on the computer, and it works the same way, so I guess it is about the exercise setting ?
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let p = e.target.previousElementSibling;
p.className = 'highlight';
}
});
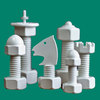
Steven Parker
231,269 PointsIt does work the same with the extra variable. But if you don't intend to use that variable again, why create it?

Jason Egipto
9,185 PointsHey Luke Lai,
Im experiencing the same issue, following :)
George Bucio
2,743 PointsGeorge Bucio
2,743 PointsThanks! I guess I didn't read the instructions carefully enough.
I edited the variable to paragraphSibling and used .previousSibling, etc.
It worked.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsFYI: Since not all nodes are elements, "previousElementSibling" would be a better choice in general, but it's not necessary here since the two elements are adjacent.