Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial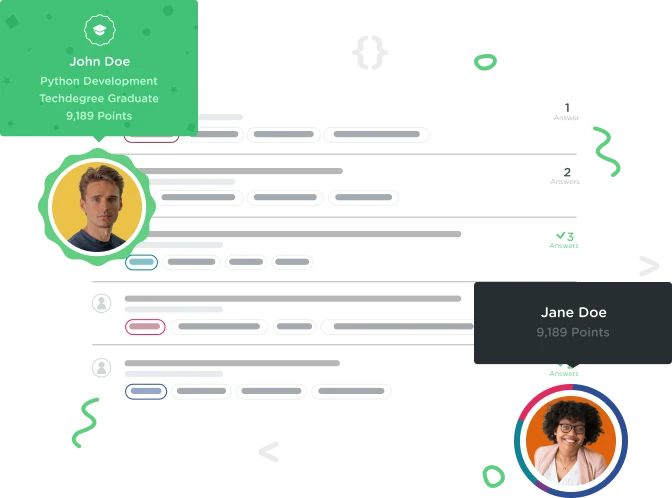

Christopher Kemp
3,446 Points"/signatures/new"
Not sure what i am missing here.
<p>Please enter your name below.</p>
<form method="post" action="/signatures/create">
<input type="text" name="signature">
<input type="submit">
</form>
post "/signatures/create" do
end
require "sinatra"
# Appends the signature to the file as a new line.
def save_signature(signature)
File.open("signatures.txt", "a") do |file|
file.puts signature
end
end
get "/signatures/new" do
erb :new
end
post "/signatures/create" do
save_signature(params[:signature])
redirect URI.escape("#{"/signatures/new"}"
end
1 Answer
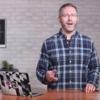
Jay McGavren
Treehouse TeacherLooks like you cleared three of the tasks with no problem, but got stuck on the fourth. I've copied your code below and added comments.
<p>Please enter your name below.</p>
<form method="post" action="/signatures/create">
<input type="text" name="signature">
<input type="submit">
</form>
<!-- The below code isn't causing the challenge to fail, but it shouldn't be here. -->
post "/signatures/create" do
end
require "sinatra"
# Appends the signature to the file as a new line.
def save_signature(signature)
File.open("signatures.txt", "a") do |file|
file.puts signature
end
end
get "/signatures/new" do
erb :new
end
post "/signatures/create" do
save_signature(params[:signature])
# This line is the reason you were getting a "Try again" error - it's not compiling.
# The most serious problem is that there's no closing ) at the end.
# redirect URI.escape("#{"/signatures/new"}"
# But there's a bunch of other stuff going on there that you don't need.
# I'm actually a little surprised that it compiles even with a ), but it looks like
# what you have there is equivalent to this line (which will also pass the challenge):
redirect "/signatures/new"
# "#{"/signatures/new"}" is the same as "/signatures/new". You shouldn't use "#{}"
# string interpolation unless there is Ruby code or a variable that you want to include
# in a string.
# And you also don't need URI.escape, because there are no characters in
# "/signatures/new" that are invalid for a URL.
end