Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial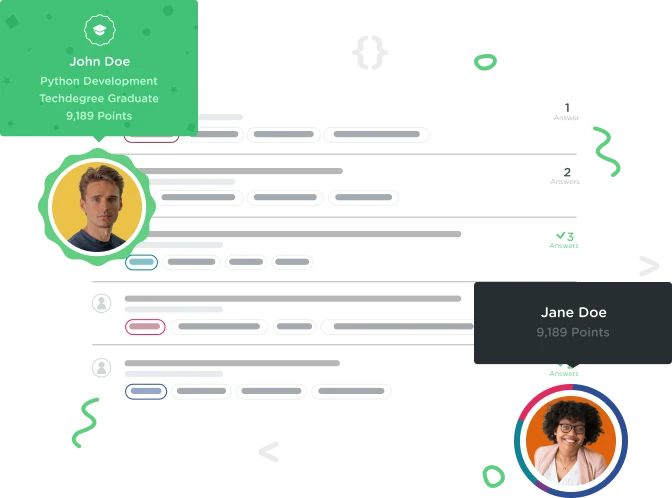
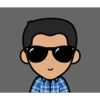
busterlabs
9,276 PointsSigning Up New Users: Part 2 (PFUser)
Challenge Task 1 of 4
In our view controller below we want to sign up new users for our app. In the signup method, start by capturing the username and password from two UITextField properties: 'usernameField' and 'passwordField'. Store the values in the properties 'username' and 'password'.
My code:
import "SignupViewController.h"
import <Parse/Parse.h>
@implementation SignupViewController
- (IBAction)signup:(id)sender { NSString *username = [self.usernameField.text]; NSString *password = [self.passwordField.text];
}
@end
Result:
Bummer! Compilation Error! Don't forget to use 'self' when accessing properties, and make sure the rest of your syntax is correct!
10 Answers
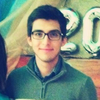
Andres Oliva
7,810 PointsHahahahaha!
This lines of code:
[newUser signUpInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {}];
NSLog("%d",1) }
Should be this:
[newUser signUpInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {
NSLog(@"%@", succeeded);
}];
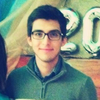
Andres Oliva
7,810 PointsYou shouldn't write those square brackets.
Try this:
NSString *username = self.usernameField.text;
NSString *password = self.passwordField.text;
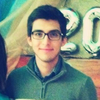
Andres Oliva
7,810 PointsOk, I took a look at the challenge. It says that username and password are properties, so you need to access them as properties of your class, not as local variables.
This should work:
#import "SignupViewController.h"
#import <Parse/Parse.h>
@implementation SignupViewController
- (IBAction)signup:(id)sender {
self.username = self.usernameField.text;
self.password = self.passwordField.text;
}
@end
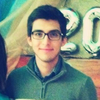
Andres Oliva
7,810 PointsYou need to read the challenges more carefully.
You need to initialize the newUser variable using the "user" class method. You are somehow using a "newUser" method. Also, you need to access username and password as properties (as stated) not as local variables.
Try this:
- (IBAction)signup:(id)sender {
self.username = self.usernameField.text;
self.password = self.passwordField.text;
PFUser *newUser = [PFUser user];
newUser.username = self.username;
newUser.password = self.password;
}
I also noticed that you put an extra curly brace "}" in your code.
Be more careful! In the real development life, these would be errors, and your code wouldn't compile. Just like is happening with the code challenges :/
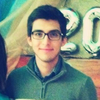
Andres Oliva
7,810 PointsHahahaha.
You are almost correct. You forgot the square brackets this time. This should be the line of code:
[newUser signUpInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {}];
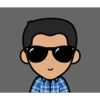
busterlabs
9,276 PointsHi Andres. I tried the your code as below:
import "SignupViewController.h"
import <Parse/Parse.h>
@implementation SignupViewController
- (IBAction)signup:(id)sender { NSString *username = self.usernameField.text; NSString *password = self.passwordField.text; }
@end
Then got another error result:
Bummer! Make sure you set both the 'username' and 'password' properties!
Any other suggestions?
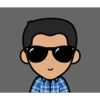
busterlabs
9,276 PointsThanks!
Can you take a look at my second code for challenge 2?
Challenge Task 2 of 4
Next, create a new PFUser variable named 'newUser' and initialize it using the 'user' class method from PFUser. Set the 'username' and 'password' properties of this user using the properties you just set.
My code:
import "SignupViewController.h"
import <Parse/Parse.h>
@implementation SignupViewController
- (IBAction)signup:(id)sender { self.username = self.usernameField.text; self.password = self.passwordField.text; } PFUser *newUser = [PFUser newUser]; newUser.username = username; newUser.password = password; } @end
Result:
Bummer! Compilation Error! Make sure you are correctly initializing the 'newUser' variable, and check your syntax.
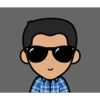
busterlabs
9,276 PointsHaha. Thanks for the heads up. Right on cue, still stuck on #3 now
Challenge Task 3 of 4
Now let's use the 'signUpInBackgroundWithBlock' method to sign up this new user. Send this message to the newUser variable. For the block parameter, copy and paste the following empty block: ^(BOOL succeeded, NSError *error) {}
My code:
newUser signUpInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {}
Result:
Bummer! Compilation Error! Block syntax can be tricky! Copy and paste the empty block from the instructions and check the rest of your syntax.
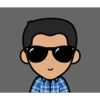
busterlabs
9,276 PointsHi Andres! So you knew this was coming right? Might as well finish the whole challenge why don't you?
hallenge Task 4 of 4
For now, inside the block, simply log the 'succeeded' parameter using NSLog. BOOL values are really just int values (0 or 1), so you can use the int format specifier: %d. Remember: format specifiers are placed in between the double quotes of an NSString literal, and then the values to be plugged in are specified after the NSString, separated by commas.
My code:
import "SignupViewController.h"
import <Parse/Parse.h>
@implementation SignupViewController
- (IBAction)signup:(id)sender { self.username = self.usernameField.text; self.password = self.passwordField.text;
PFUser *newUser = [PFUser user]; newUser.username = self.username; newUser.password = self.password;
[newUser signUpInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {}];
NSLog("%d",1) }
@end
Result:
Bummer! Compilation Error! Check your syntax! Follow the instructions carefully.
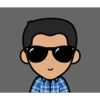
busterlabs
9,276 PointsThanks bud. Appreciate it. This part definitely was a challenge

Levi Lais
5,975 PointsI agree - it was SUPER challenging.