Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial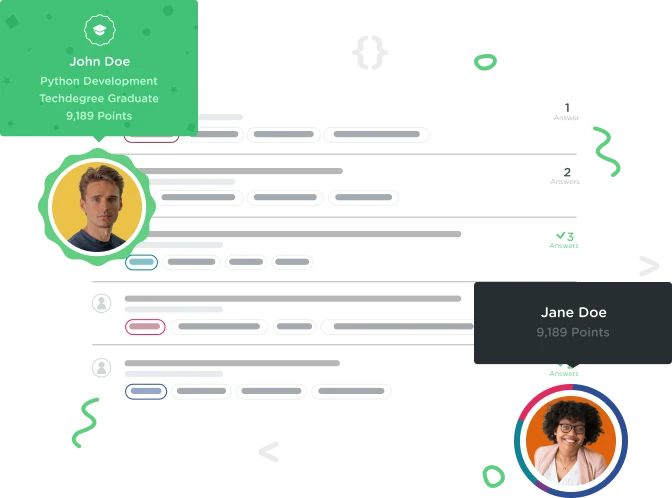

Ankush Jain
2,800 Pointssillycase
not sure why it is not working
# The first half of the string, rounded with round(), should be lowercased.
# The second half should be uppercased.
# E.g. "Treehouse" should come back as "treeHOUSE"
def sillycase (instring) :
wordLen = len(instring)
halfwordLen = int(wordLen/2)
firsthalf = instring[:round(halfwordLen)]
secondhalf = inString[halfwordLen:]
for char in firsthalf:
newFirstString = firsthalf.replace(char,char.lower())
for char in secondhalf:
newSecondString = secondhalf.replace(char,char.upper())
return (newFirstString + newSecondString)
3 Answers
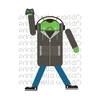
Stephen Bone
12,359 PointsHi Ankush
The initial error I receive is NameError: name 'inString' is not defined on the line below. This is because you've put a capital 'S' in this variable but not anywhere else so this just needs to be changed to match the rest.
secondhalf = inString[halfwordLen:]
So once that's corrected and I call your function with sillycase('treehouse') the output received is treehousE which still isn't quite what we need. The cause of this is that your for loop keeps resetting the values of newfirstString and newSecondString so for example newSecondString does the below on each iteration of the loop:
House
hOuse
hoUse
houSe
housE
I imagine with a bit of thought you could make this work or you could just use the upper and lower methods you already take advantage of to change the entire strings in one go rather than looping. So for example you could just do something like:
firsthalf = firsthalf.lower()
secondhalf = secondhalf.upper()
return firsthalf + secondhalf
Hope it helps!
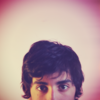
Nabil Kamel
7,170 PointsTry to always use a piece of paper and a pen to really see what you need. The only thing you need to know here is the index of the letter that separates the word.
- the 'round' function was not necessary as you already changed the value into an int.
- you don't need to loop as you are separating the word. You just need to apply the corresponding method(upper or lower)
- always comment your functions inside the definition as I did here.
def sillycase (w) :
"""
The first half of the string, rounded, should be lowercased.
The second half should be uppercased.
E.g. "Treehouse" should come back as "treeHOUSE"
"""
i = int(len(w)/2)
return (w[:i].lower() + w[i:].upper())

Brandon Raper
5,360 PointsI think it gives erroneous results when casting your halfwordLen as an INT. Any odd number divided by two would have the decimal places truncated. My round() is more like this...
i = round(float(len(mystr)/2))