Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial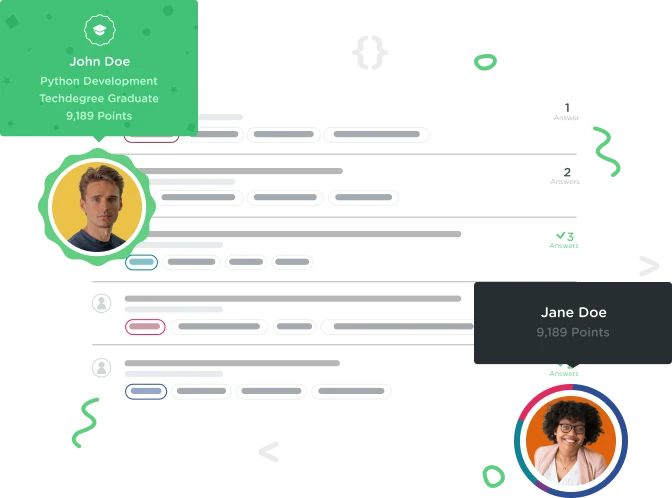

Andrew Bickham
1,461 Pointssillycase
its keeps giving me a bummer message saying I need to use int() or // which I have
def sillycase(word):
first_half = int(len(word))/ 2
first_slice = word[:first_half].lower()
second_half = int(len(word)) - int(first_half)
second_slice = word[second_half:].upper()
return int(first_slice) + int(second_slice)
2 Answers
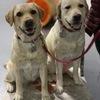
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Andrew,
This line:
first_half = int(len(word))/ 2
takes word
, which is a string, then computes the length of it, which is an int. Then you use the int()
function to turn that into an int (which it already is). Then you divide your int by 2, which turns it into a float.
You can prove that this is true in the Python console:
>>> word = 'hello!'
>>> type(word)
<class 'str'>
>>> length = len(word)
>>> type(length)
<class 'int'>
>>> i_length = int(length)
>>> type(i_length)
<class 'int'>
>>> first_half = i_length / 2
>>> type(first_half)
<class 'float'>
You can solve this by converting your result of the division by 2 to an int, instead of the length (i.e., put the whole calculation inside your int()
call). Alternatively, instead of doing any forced type conversion, you could use the integer division operator //
instead of the regular division operator /
, in which case you'll never a get a conversion to float here.
You have a redundancy in this line:
second_half = int(len(word)) - int(first_half)
The first half of this code is redundant: it turns something that is already an int (len
can only return an int) into an int. The second half of the code turns your float from before back into an int. However, it is too late to do the conversion here: your code will already have crashed with a TypeError in the previous line we looked at. Once you fix the error in that line, first_half
will be an int and so it will be redundant to use the int()
function here.
Moreover, this whole section of code is conceptually redundant. When you determine the index of the halfway point for your string, you have enough to make the second slice. For example:
>>> my_word = 'Python'
>>> my_word[:3] # this will give me all the characters before index 3
'Pyt'
>>> my_word[3:] # this will give me all the characters from index 3
'hon'
Observe that we use the same index value (here 3) to access both the first half and the second half.
Lastly:
return int(first_slice) + int(second_slice)
This code is attempting to convert the lowercase string of the first slice and the uppercase string of the second string of the second slice into ints (which will crash with a ValueError) and then add those ints together.
Remember that you want to return a string, which you just concatenate with +
without turning them into numbers:
>>> concatenated = 'hello ' + 'world'
>>> print(concatenated)
hello world
Hope that clears everything up for you.
Cheers
Alex

Andrei Oprescu
9,547 PointsHey!
I have a noticed your mistake.
When you declare first_half and second_half, you only include len(word), but you also need to include / 2.
for clarification, you need to make the lines like this:
first_half = int(len(word)/ 2)
and also exclude the second_half variable because you will get the same numbers from first_half and second_half.
also, when you return, you shouldn't use int() because you are concatenating two strings.
hope this helps! if you need more help, don't hesitate to ask.
Andrei

Andrew Bickham
1,461 Pointsdef sillycase(word):
first_half = int(len(word)/ 2)
first_slice = word[:first_half].lower()
second_slice = word[first_half:].upper()
return first_slice + second_slice
Add Comment
Hey, andrei & alex so I made the necessary corrections or I thought I did but I keep getting a bummer: try again, message, i honestly cant see what im missing, so if somebody could point out the flaw, that would be very much appreciated it!