Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial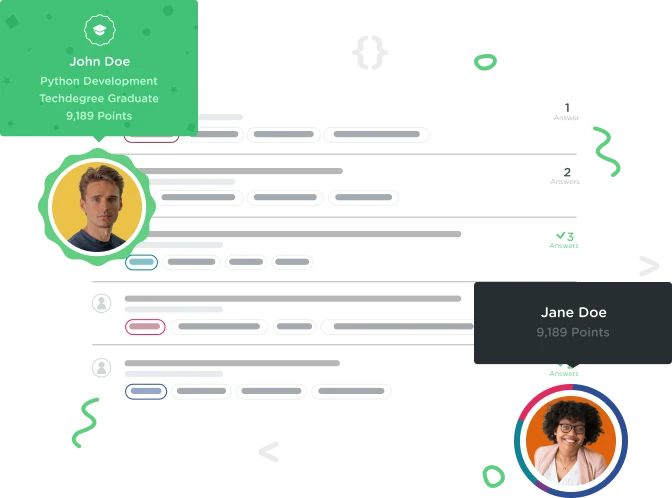
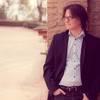
Michal Janek
Front End Web Development Techdegree Graduate 30,654 PointssillyCASE
I am absolutely positive this could be done in a matter of two lines. However, I had an issue of figuring out the general length of a potential argument so that took the longest time. Anyway, my solution passed so...not the cleanliest way but it worked.
def sillycase(arg):
length_arg = len(arg) #the length of potential word/string : treehouse as example
numbers = list(range(0, length_arg)) # I needed to make a range of objects from 0 to maximum of characters of arg.
half_stop = int(len(numbers)/2) #this is my dividing part for slicing
firstHalf = arg[:half_stop] #first half of the argument to (excluding) the half_stop
secondHalf = arg[half_stop:] #second half of the argument (including) the half_stop to the end
newList1 = [] # forstoring the separate characters from the first and second half as they will lower() /upper()
newList2 = []
for i in firstHalf: #each character from the list will be appended to empty list and transformed (upper or lowerCase)
newList1.append(i.lower())
for i in secondHalf:
newList2.append(i.upper())
newArg = "".join(newList1+newList2) #transform particular lists but concatenate them before!
print(newArg)
5 Answers
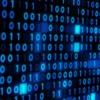
Alexander Davison
65,469 PointsIn fact, this can be accomplished in two lines.
It takes lots of thinking, but it makes sense:
def sillycase(string):
return string[:len(string)//2].lower() + string[len(string)//2:].upper()
Cleaner, easier to read solution:
def sillycase(string):
half_length = int(len(string) / 2)
first_half = string[:half_length]
second_half = string[half_length:]
return first_half.lower() + second_half.upper()
What I am doing:
- I define my function on the first line (duh)
- I return this crazy value in the first code snippet... Let's look at the second snippet's second line of code. I create a variable called
half_length
that is half of the length of the string (if the length of the string is odd it will remove out the numbers after the decimal point so that the variable will be an integer). - On the third and fourth lines of the second snippet of code, I make two variables:
first_half
andsecond_half
. They store the first half and second half of the string. I used slices to do this. - Finally, I return the first half lowercased and the second half uppercased, combined.
Then back in the first snippet I just reduced the amount of code and jammed up it into two lines.
I hope this helps and makes sense. ~Alex
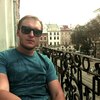
Kyryll Druzhynin
1,067 PointsI hope my version will be easy to understand. It works good with any kind of string.
- We should get the half length of the argument using floor devision.
- Than we use the result of devision to make slices from argument.
- We get the result by compiling two pieces together.
def sillycase(arg):
half = len(arg) // 2
lenght = len(arg)
first_half = arg[:half]
second_half = arg[half:]
result = str(first_half).lower() + str(second_half).upper()
return result

Tonye Jack
Full Stack JavaScript Techdegree Student 12,469 PointsA single line will do just fine.
def sillycase(string):
return "".join(string[:int(len(string)/2)]).lower() + "".join(string[int(len(string)/2):]).upper()

ryanosten
PHP Development Techdegree Student 29,615 PointsMy solution is a bit more complicated, but it should work I think (but it fails the test). In REPL, when I print my result named 'final_silly', it prints the correct value I think. When I return final_silly, I get nothing returned.
def sillycase(word): word = word.upper() arr = list(word) lowered_arr = [] z = int(0) h = int(len(word)/2) i = 0
while i < h:
lowered = arr[i].lower()
lowered_arr.append(lowered)
i += 1
arr[z:h] = lowered[z:h]
final_silly = "".join(arr)
return final_silly

Firuz Abduganiev
3,140 Pointsdef sillycase(word): half = int(len(word) / 2) word = word[:half].lower() + word[half:].upper() return word
Daniel McFarlin
5,168 PointsDaniel McFarlin
5,168 PointsI literally just stared at this answer forever trying to follow you. Then it finally clicked. I had the hardest time trying to figure out how to do this bit of code!