Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial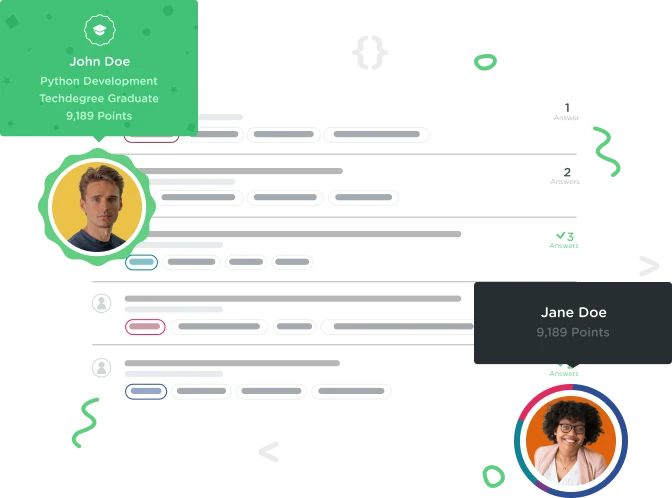

Peregrine Edison-Lahm
1,811 PointsSillycase code challenge: assume the string is of even length?
"Create a function named sillycase that takes a string and returns that string with the first half lowercased and the last half uppercased." Are we supposed to assume that the string entered is has an even number of characters? Otherwise, how do we divide it in half? I suppose that if the length is odd we could just leave the middle character unchanged. Or, we could choose to add the extra character to the first half or second half: def sillycase(mystring): half = len(mystring)//2 If len(mystring)%2 = 1: return lower(mystring[:half+1]) + upper(mystring[half+2:half]) Else: return lower(mystring[:half+1]) + upper(mystring[half+2:])
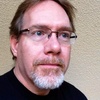
Chris Freeman
Treehouse Moderator 68,423 PointsI am unfamiliar with the built-in function lower()
. Just the method string.lower()
.
6 Answers
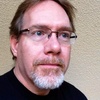
Chris Freeman
Treehouse Moderator 68,423 PointsThe sillycase challange says:
Create a function named sillycase
that takes a string and returns that string with the first half lowercased and the last half uppercased.
A key additional piece of information is in the challenge comments:
# The first half of the string, rounded with round(), should be lowercased.
# The second half should be uppercased.
# E.g. "Treehouse" should come back as "treeHOUSE"
Setting that aside for a moment, your concept of checking for odd or even length can also work. I've cleared up a few errors in your code to produce this:
def sillycase(mystring):
half = len(mystring)//2 #<-- This should use round() not integer division
if False: #len(mystring)%2 == 1: #<-- `if` lower case; comparison needs `==`
return mystring[:half].lower() + mystring[half:].upper() #<-- corrected slice limits
else: #<-- `else` lower case
return mystring[:half+1].lower() + mystring[half+1:].upper() #<-- corrected slice limits
Using round()
, sillycase
can be written as:
def sillycase(mystring):
half = round(len(mystring)/2)
return mystring[:half].lower() + mystring[half:].upper()
The difference between using round() and
\` can be seen in this test code:
In [50]: for x in range(15):
print("with x={} {}//2 gives {} vs round({}/2) gives {}".format(x, x, x//2, x, round(x/2) ))
....:
with x=0 0//2 gives 0 vs round(0/2) gives 0
with x=1 1//2 gives 0 vs round(1/2) gives 0
with x=2 2//2 gives 1 vs round(2/2) gives 1
with x=3 3//2 gives 1 vs round(3/2) gives 2
with x=4 4//2 gives 2 vs round(4/2) gives 2
with x=5 5//2 gives 2 vs round(5/2) gives 2
with x=6 6//2 gives 3 vs round(6/2) gives 3
with x=7 7//2 gives 3 vs round(7/2) gives 4
with x=8 8//2 gives 4 vs round(8/2) gives 4
with x=9 9//2 gives 4 vs round(9/2) gives 4
with x=10 10//2 gives 5 vs round(10/2) gives 5
with x=11 11//2 gives 5 vs round(11/2) gives 6
with x=12 12//2 gives 6 vs round(12/2) gives 6
with x=13 13//2 gives 6 vs round(13/2) gives 6
with x=14 14//2 gives 7 vs round(14/2) gives 7
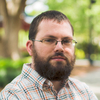
Kenneth Love
Treehouse Guest Teacher// != round()
and will not always give you the right answer. There's a reason I tell you to use round()
.
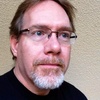
Chris Freeman
Treehouse Moderator 68,423 PointsAnswer updated to show difference between using round()
and \\

Peregrine Edison-Lahm
1,811 PointsThanks for your help! There's something I'm still not understanding: "# The first half of the string, rounded with round(), should be lowercased.# The second half should be uppercased." means that the first half should be rounded down and the second half should be rounded up? I don't understand how I could have arrived at that conclusion. However, your answer and other discussions about this challenge seem to imply that others have understood this. What am I missing? Not trying to be argumentative, just wanting to make sure I can understand the questions better next time. Thanks again.
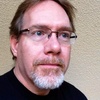
Chris Freeman
Treehouse Moderator 68,423 PointsI think there are two aspects here. First, which part of the string is to be lowercase and which uppercase. It seems that both the instructions and comments agree on this:
... returns that string with the first half lowercased and the last half uppercased.
#The first half of the string, ..., should be lowercased
The comment implying that the remaining last half should be uppercased.
The second aspect concerns rounding. The part saying rounded with round()
is implying the method used determine unclear "halving". Said in a longer form, the sentence could be written:
"The first half of the string, [How do you determing 'half', you ask? the midpoint should be] rounded with round(), should be lowercase."

Peregrine Edison-Lahm
1,811 Pointsbtw Chris, your screenshot doesn't capture the full length of the comments that you helpfully (thanks) included.
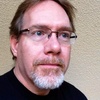
Chris Freeman
Treehouse Moderator 68,423 PointsThanks. The formating of the forum pages clips the text. You need to use the scroll bars on the bottom of each code block to see more....

Peregrine Edison-Lahm
1,811 PointsGot it. I still think the sentence leaves it ambiguous re: whether or not there should be a middle character that is not altered by upper() or lower(), but hey, I'm learning stuff and I appreciate it!
No scroll bars in the code block. I'll try in different browsers.
Thanks again for your time on this!
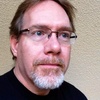
Chris Freeman
Treehouse Moderator 68,423 PointsRight! I had only included one line from the comment. I've edited the anwser above to include all three comment lines.
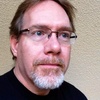
Chris Freeman
Treehouse Moderator 68,423 PointsThe other implied bit that may be considered ambiguous is there are only two parts mentioned: "first half" and "last half". The mentioning of using round()
implies that a "middle" character needs to be resolved to belong to one of those two parts. Keep at it! You'll get better at resolving the ambiguities.

Peregrine Edison-Lahm
1,811 PointsSilly me. The third line should have answered my question all along!
E.g. "Treehouse" should come back as "treeHOUSE"
Sigh....... (and d'oh)
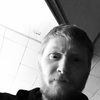
Kody Dibble
2,554 PointsI honestly have no idea how to accomplish this task.... Am I using round to round up the length of the string? Or down persay...
Also how to I divide the string..
Is there anyway to not divide the string and just use indexing?
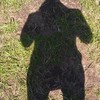
Nathan McElwain
4,575 Pointsso since you don't know the string length, you need a function that works for an argument of any length. First, you find the string's length (len()), divide by 2, and round, up or down, depending on the outcome of your length division. NOW you use your index to assign the cases of the returned string according to the rounded length you established.
def sillycase(a):
x = round(len(a)/2)
y = a[x:].upper()
z = a[:x].lower()
return z+y
Peregrine Edison-Lahm
1,811 PointsPeregrine Edison-Lahm
1,811 PointsIs it better to use the built-in string method, as below (as opposed to the built-in function, in my question above)? (Here, for cases where the total number of characters is odd, I have just gone ahead and ignored the middle character.) def sillycase(mystring): half = len(mystring)//2 first = mystring[:half+1] second = mystring[half+2:] return first.lower() + return second.upper()