Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial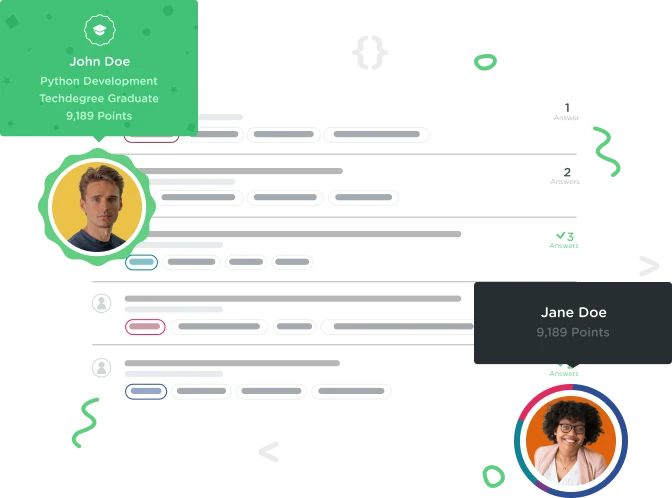

jasonswartzbaugh
3,661 PointsSillycase Code Validation
I just completed the code for the Python course "sillycase challenge" The code I wrote is:
def sillycase(getString):
firstHalf = getString[0:len(getString) / 2]
secondHalf = getString[len(getString) / 2:]
return firstHalf.lower() + secondHalf.upper()
When I click the "Check Work" button, I receive the following message:
Bummer! slice indices must be integers or None or have an index method
However, when I run this code in Python, the function gets created, and it works correctly.
Here's what I ran directly in the Python interpreter:
>>> def sillycase(getString):
... firstHalf = getString[0:len(getString) / 2]
... secondHalf = getString[len(getString) / 2:]
... return firstHalf.lower() + secondHalf.upper()
...
>>> sillycase("treehouse")
'treeHOUSE'
>>>
What gives?
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Jason,
What version of python are you running in your interpreter? I suspect it is a 2.x version if that code is running successfully.
In python 3 the behavior of the division operator has changed and it now yields a float. So the example here would be 9 / 2 = 4.5 and this would not be a valid index to slice on. In python 2 the result would be 4 and that may be what you are running when it works.
The comments in the code mention that you should use round(). That way the index you calculate can be rounded to the correct integer.
So you can do something like this round(len(getString) / 2)
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsThere is a floor division operator in python,
//
, which will yield an integer result. It passes the result of the division through the "floor" function. So 9 // 2 = 4However, you can't pass the challenge with this because it does not always produce the same result as
round()