Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial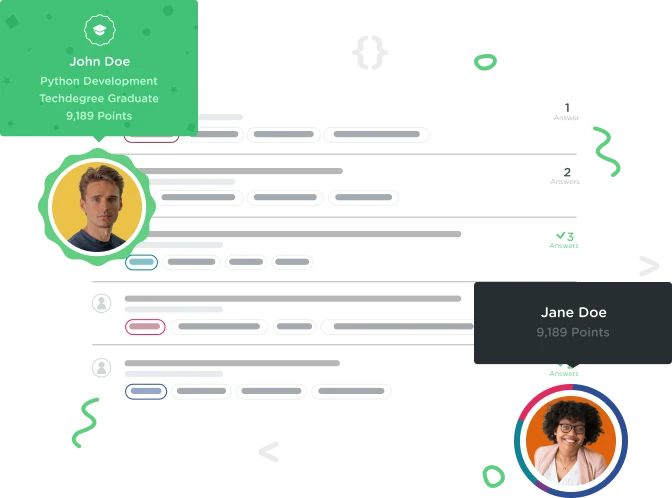

Abdul Wahab
174 Points[Simple And Exact Answer] to Guil Challenge
- First create a function expression named as checkButton
- Function takes children of the ul as argument
- Since at app start there are only four list items in the ul
- So in first list item up button is hidden 5 . In last list item down button is hidden
-
And if its not first and last child than of course make up and down button visible again
const checkButton = (list) => {
console.log(list[0]); console.log(list[0].querySelector('button.up')); // see output in console for above two lines for better understanding what are they printing
for (let i = 0; i < list.length; i++) { if (i === 0) { list[i].querySelector('button.up').style.visibility = 'hidden'; } if (i === list.length - 1) { list[i].querySelector('button.down').style.visibility = 'hidden'; } if (i !== 0 && i !== list.length - 1) { list[i].querySelector('button.up').style.visibility = 'visible'; list[i].querySelector('button.down').style.visibility = 'visible'; } }
}; Then call the check function after printing all the buttons on the screen so that our checkButton() can hide up button and down button
// loop that prints all the buttons (probably this part is on line 48 or something near 48 ) const ulchildren = listUl.children; for (let i = 0; i < ulchildren.length; i++) { addButtons(ulchildren[i]); }
// check buttons after printing all buttons checkButton(ulchildren);
-
Remember we have to always check the buttons even when an item is removed, moved up or moved down
listUl.addEventListener('click', (event) => { if (event.target.tagName == 'BUTTON') { // code for removing the child node // Call checkButton just before the closing parenthesis of the first if statement checkButton(ulchildren);
} }); Similarly we also have to again call the checkButton() when a new item is added or addItemButton.addEventListener('click', () => { let li = document.createElement('li'); li.textContent = addItemInput.value; addButtons(li); listUl.appendChild(li); addItemInput.value = ''; // like this below checkButton(ulchildren); });
1 Answer
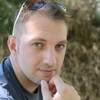
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsPlease use markdown for your posts.